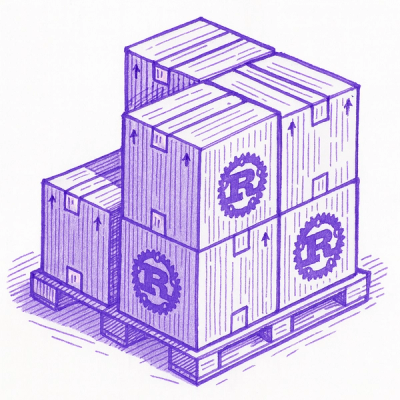
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
node-laravel-encryptor
Advanced tools
NodeJS version of Laravel's Encrypter Class, tested 5.4.30 to 6.0 Illuminate/Encryption/Encrypter.php
With this module you can create the encrypted payload for a cookie from Node Js and be read by Laravel.
Laravel only allows AES-128-CBC
AES-256-CBC
.
If no algorithm is defined default is AES-256-CBC
{
"iv": "iv in base64",
"value": "encrypted data",
"mac": "Hash HMAC"
}
$> npm i node-laravel-encryptor
const {Encryptor} = require('node-laravel-encryptor');
let encryptor = new Encryptor({
key: 'Laravel APP_KEY without base64:',
});
encryptor
.encrypt({foo: 'bar'})
.then(enc => console.log(enc));
encryptor
.decrypt(enc)
.then(dec => console.log(dec));
base64:
DEPRECIATED
if no key_length
is given default is 64.
arguments:
if no serialize
option is given default is to serialize.
arguments:
if no serialize
option is given default is to unserialize.
$> npm run test
To be able to run PHP test you must have installed:
$> npm run test
node Laravel Encrypter
✓ should cipher and decipher
✓ should fail cipher and decipher object without serialize
✓ should cipher and decipher with no key_length defined
✓ should cipher and decipher with no serialize nor unserialize
✓ should fail cipher not valid Laravel Key
✓ should fail cipher not valid algorithm
✓ should fail decipher not valid data
✓ should cipher and decipher multiple times
✓ should decipher data at Laravel correctly (52ms)
✓ should decipher from Laravel correctly (51ms)
10 passing (128ms)
FAQs
node version Laravel Illuminate/Encryption/Encrypter.php
The npm package node-laravel-encryptor receives a total of 703 weekly downloads. As such, node-laravel-encryptor popularity was classified as not popular.
We found that node-laravel-encryptor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.