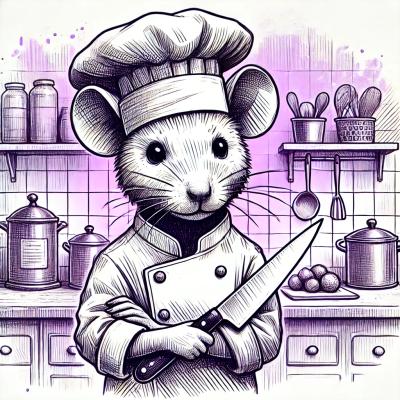
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
node-line-reader
Advanced tools
Node Line Reader is a Node.js module that helps you reading lines of text from a file.
Features:
Installation:
npm install node-line-reader
LineReader
var path = require('path');
var LineReader = require('node-line-reader').LineReader;
var reader = new LineReader(path.join('/home/user', 'some-file.txt'));
// Each execution of nextLine will get a following line of text from the input file
reader.nextLine(function (err, line) {
if (!err) {
console.log('file line: ', line);
}
});
LineTransform
var stream = getSomeReadableStream(); // Create read stream
var LineTransform = require('node-line-reader').LineTransform; // LineTransform constructor
var transform = new LineTransform();
stream.pipe(transform); // Pipe input from a file stream over to line transform
transform.on('data', function(line) {
// line - single line of text from input file
});
transform.on('end', function() {
// no more text lines
});
LineFilter
var stream = getSomeReadableStream(); // Create read stream
var LineTransform = require('node-line-reader').LineTransform; // LineTransform constructor
var LineFilter = require('node-line-reader').LineFilter; // LineFilter constructor
var transform = new LineTransform();
// Skip empty lines and lines with "et" (with leading and trailing space) in them
var filter = new LineFilter({ skipEmpty: true, exclude: [/\bet\b/ });
// Pipe input from a file stream over to line transform and to the filter
stream.pipe(transform).pipe(filter);
filter.on('data', function(line) {
// line - single line of text from input file
});
filter.on('end', function() {
// no more text lines
});
LineReader
LineReader
is a text lines reader from a specified file.
This function reads another line of text from a specified file and passes it over to the callback method.
The callback has arguments (err, line)
. The err
argument is an error that occurred while reading a line of text (null
if no error occurred). The line
is a string with line of text from a specified input file.
LineFilter
LineFilter
is a duplex stream passing through lines of text matching include and exclude rules. LineFilter
instance can be piped into antoher Writable instance.
The LineFilter
accepts options objects with following parameters:
skipEmpty
- boolean value blocking empty linesskipBlank
- boolean value blocking blank lines (lines composed of whitespace characters)include
- a single instance or an array of regular expressions (see below for text filtering rules)exclude
- a single instance or an array of regular expressions (see below for text filtering rules)Text filtering rules:
include
pattern(s) are provided, filter will pass through lines of text which are matched by the include pattern(s) onlyexclude
pattern(s) are provided, fillter will pass through all lines except the ones which are matched by the exclude pattern(s) onlyinclude
and exclude
pattern(s) are provided, filter filter will pass through lines of text which are matched by the include pattern(s) and not matched by the exclude pattern(s)When a line of text can be read from the transform, it will emit a 'readable' event.
line
Buffer | String The line of text.This event fires when there will be no more test to read.
As Stream 'close' event: Emitted when the underlying resource (for example, the backing file descriptor) has been closed. Not all streams will emit this.
As Stream 'close' event: Emitted if there was an error receiving data.
LineTransform
LineTransform
is a duplex stream converting input text into lines of text. LineTransform
instance can be piped into antoher Writable instance.
When a line of text can be read from the transform, it will emit a 'readable' event.
line
Buffer | String The line of text.This event fires when there will be no more test to read.
As Stream 'close' event: Emitted when the underlying resource (for example, the backing file descriptor) has been closed. Not all streams will emit this.
As Stream 'close' event: Emitted if there was an error receiving data.
Writen by Tom Pawlak - Blog
The MIT License (MIT)
Copyright © 2014 Tom Pawlak
FAQs
Reads lines of text from input stream
The npm package node-line-reader receives a total of 747 weekly downloads. As such, node-line-reader popularity was classified as not popular.
We found that node-line-reader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.