node-mocks-http
Advanced tools
Comparing version
@@ -6,6 +6,6 @@ 'use strict'; | ||
var app = exports = module.exports = {}; | ||
var app = (exports = module.exports = {}); | ||
var trustProxyDefaultSymbol = '@@symbol:trust_proxy_default'; | ||
app.init = function(){ | ||
app.init = function () { | ||
this.cache = {}; | ||
@@ -17,3 +17,3 @@ this.settings = {}; | ||
app.defaultConfiguration = function(){ | ||
app.defaultConfiguration = function () { | ||
this.enable('x-powered-by'); | ||
@@ -41,4 +41,6 @@ this.set('etag', 'weak'); | ||
Object.defineProperty(this, 'router', { | ||
get: function() { | ||
throw new Error('\'app.router\' is deprecated!\nPlease see the 3.x to 4.x migration guide for details on how to update your app.'); | ||
get: function () { | ||
throw new Error( | ||
"'app.router' is deprecated!\nPlease see the 3.x to 4.x migration guide for details on how to update your app." | ||
); | ||
} | ||
@@ -48,7 +50,7 @@ }); | ||
app.lazyrouter = function() {}; | ||
app.handle = function() {}; | ||
app.route = function() {}; | ||
app.render = function() {}; | ||
app.listen = function() {}; | ||
app.lazyrouter = function () {}; | ||
app.handle = function () {}; | ||
app.route = function () {}; | ||
app.render = function () {}; | ||
app.listen = function () {}; | ||
@@ -59,11 +61,11 @@ app.use = function use() { | ||
app.engine = function() { | ||
app.engine = function () { | ||
return this; | ||
}; | ||
app.param = function() { | ||
app.param = function () { | ||
return this; | ||
}; | ||
app.set = function(setting, val){ | ||
app.set = function (setting, val) { | ||
if (arguments.length === 1) { | ||
@@ -77,24 +79,24 @@ return this.settings[setting]; | ||
app.path = function(){ | ||
app.path = function () { | ||
return ''; | ||
}; | ||
app.enabled = function(setting){ | ||
app.enabled = function (setting) { | ||
return !!this.set(setting); | ||
}; | ||
app.disabled = function(setting){ | ||
app.disabled = function (setting) { | ||
return !this.set(setting); | ||
}; | ||
app.enable = function(setting){ | ||
app.enable = function (setting) { | ||
return this.set(setting, true); | ||
}; | ||
app.disable = function(setting){ | ||
app.disable = function (setting) { | ||
return this.set(setting, false); | ||
}; | ||
methods.forEach(function(method){ | ||
app[method] = function(){ | ||
methods.forEach(function (method) { | ||
app[method] = function () { | ||
return this; | ||
@@ -104,3 +106,3 @@ }; | ||
app.all = function(){ | ||
app.all = function () { | ||
return this; | ||
@@ -107,0 +109,0 @@ }; |
@@ -16,3 +16,3 @@ 'use strict'; | ||
function createApplication() { | ||
var app = function() {}; | ||
var app = function () {}; | ||
@@ -19,0 +19,0 @@ mixin(app, EventEmitter.prototype, false); |
@@ -13,8 +13,11 @@ 'use strict'; | ||
var req = exports = module.exports = { | ||
__proto__: http.IncomingMessage.prototype | ||
}; | ||
var req = | ||
(exports = | ||
module.exports = | ||
{ | ||
__proto__: http.IncomingMessage.prototype | ||
}); | ||
req.header = function(name) { | ||
switch (name = name.toLowerCase()) { | ||
req.header = function (name) { | ||
switch ((name = name.toLowerCase())) { | ||
case 'referer': | ||
@@ -30,3 +33,3 @@ case 'referrer': | ||
req.accepts = function() { | ||
req.accepts = function () { | ||
var accept = accepts(this); | ||
@@ -36,3 +39,3 @@ return accept.types.apply(accept, arguments); | ||
req.acceptsEncodings = function(){ | ||
req.acceptsEncodings = function () { | ||
var accept = accepts(this); | ||
@@ -44,3 +47,3 @@ return accept.encodings.apply(accept, arguments); | ||
req.acceptsCharsets = function(){ | ||
req.acceptsCharsets = function () { | ||
var accept = accepts(this); | ||
@@ -52,3 +55,3 @@ return accept.charsets.apply(accept, arguments); | ||
req.acceptsLanguages = function(){ | ||
req.acceptsLanguages = function () { | ||
var accept = accepts(this); | ||
@@ -60,3 +63,3 @@ return accept.languages.apply(accept, arguments); | ||
req.range = function(size){ | ||
req.range = function (size) { | ||
var range = this.get('Range'); | ||
@@ -87,3 +90,3 @@ if (!range) { | ||
req.is = function(types) { | ||
req.is = function (types) { | ||
if (!Array.isArray(types)) { | ||
@@ -101,11 +104,11 @@ types = [].slice.call(arguments); | ||
defineGetter(req, 'secure', function secure(){ | ||
defineGetter(req, 'secure', function secure() { | ||
return this.protocol === 'https'; | ||
}); | ||
defineGetter(req, 'ip', function ip(){ | ||
defineGetter(req, 'ip', function ip() { | ||
return this.options.ip || '127.0.0.1'; | ||
}); | ||
defineGetter(req, 'ips', function ips(){ | ||
defineGetter(req, 'ips', function ips() { | ||
return [this.ip]; | ||
@@ -152,3 +155,3 @@ }); | ||
defineGetter(req, 'fresh', function(){ | ||
defineGetter(req, 'fresh', function () { | ||
var method = this.method; | ||
@@ -161,3 +164,3 @@ var statusCode = this.res.statusCode; | ||
if (statusCode >= 200 && statusCode < 300 || statusCode === 304) { | ||
if ((statusCode >= 200 && statusCode < 300) || statusCode === 304) { | ||
return fresh(this.headers, this.res._headers || {}); | ||
@@ -164,0 +167,0 @@ } |
import { Request, Response, CookieOptions } from 'express'; | ||
import { IncomingMessage, OutgoingMessage } from 'http'; | ||
export type RequestMethod = 'CONNECT' | 'DELETE' | 'GET' | 'HEAD' | 'OPTIONS' | 'PATCH' | 'POST' | 'PUT' | 'TRACE'; | ||
export type RequestMethod = | ||
'CONNECT' | 'DELETE' | 'GET' | 'HEAD' | 'OPTIONS' | 'PATCH' | 'POST' | 'PUT' | 'TRACE'; | ||
export interface Params { | ||
@@ -21,3 +19,3 @@ [key: string]: any; | ||
export interface Headers { | ||
'accept'?: string; | ||
accept?: string; | ||
'accept-language'?: string; | ||
@@ -32,8 +30,8 @@ 'accept-patch'?: string; | ||
'access-control-max-age'?: string; | ||
'age'?: string; | ||
'allow'?: string; | ||
age?: string; | ||
allow?: string; | ||
'alt-svc'?: string; | ||
'authorization'?: string; | ||
authorization?: string; | ||
'cache-control'?: string; | ||
'connection'?: string; | ||
connection?: string; | ||
'content-disposition'?: string; | ||
@@ -46,9 +44,9 @@ 'content-encoding'?: string; | ||
'content-type'?: string; | ||
'cookie'?: string; | ||
'date'?: string; | ||
'expect'?: string; | ||
'expires'?: string; | ||
'forwarded'?: string; | ||
'from'?: string; | ||
'host'?: string; | ||
cookie?: string; | ||
date?: string; | ||
expect?: string; | ||
expires?: string; | ||
forwarded?: string; | ||
from?: string; | ||
host?: string; | ||
'if-match'?: string; | ||
@@ -59,20 +57,20 @@ 'if-modified-since'?: string; | ||
'last-modified'?: string; | ||
'location'?: string; | ||
'pragma'?: string; | ||
location?: string; | ||
pragma?: string; | ||
'proxy-authenticate'?: string; | ||
'proxy-authorization'?: string; | ||
'public-key-pins'?: string; | ||
'range'?: string; | ||
'referer'?: string; | ||
range?: string; | ||
referer?: string; | ||
'retry-after'?: string; | ||
'set-cookie'?: string[]; | ||
'strict-transport-security'?: string; | ||
'tk'?: string; | ||
'trailer'?: string; | ||
tk?: string; | ||
trailer?: string; | ||
'transfer-encoding'?: string; | ||
'upgrade'?: string; | ||
upgrade?: string; | ||
'user-agent'?: string; | ||
'vary'?: string; | ||
'via'?: string; | ||
'warning'?: string; | ||
vary?: string; | ||
via?: string; | ||
warning?: string; | ||
'www-authenticate'?: string; | ||
@@ -129,3 +127,3 @@ [header: string]: string | string[] | undefined; | ||
[key: string]: any; | ||
} | ||
}; | ||
@@ -142,3 +140,3 @@ export interface ResponseOptions { | ||
options: CookieOptions; | ||
} | ||
}; | ||
@@ -161,4 +159,4 @@ export type MockResponse<T extends OutgoingMessage> = T & { | ||
cookies: {[name: string]: ResponseCookie}; | ||
} | ||
cookies: { [name: string]: ResponseCookie }; | ||
}; | ||
@@ -174,2 +172,5 @@ export function createRequest<T extends IncomingMessage = Request>(options?: RequestOptions): MockRequest<T>; | ||
export function createMocks<T1 extends IncomingMessage = Request, T2 extends OutgoingMessage = Response>(reqOptions?: RequestOptions, resOptions?: ResponseOptions): Mocks<T1, T2>; | ||
export function createMocks<T1 extends IncomingMessage = Request, T2 extends OutgoingMessage = Response>( | ||
reqOptions?: RequestOptions, | ||
resOptions?: ResponseOptions | ||
): Mocks<T1, T2>; |
@@ -41,7 +41,17 @@ 'use strict'; | ||
var standardRequestOptions = [ | ||
'method', 'url', 'originalUrl', 'baseUrl', 'path', 'params', 'session', 'cookies', 'headers', 'body', 'query', 'files' | ||
'method', | ||
'url', | ||
'originalUrl', | ||
'baseUrl', | ||
'path', | ||
'params', | ||
'session', | ||
'cookies', | ||
'headers', | ||
'body', | ||
'query', | ||
'files' | ||
]; | ||
function createRequest(options) { | ||
if (!options) { | ||
@@ -63,4 +73,3 @@ options = {}; | ||
mockRequest.baseUrl = options.baseUrl || mockRequest.url; | ||
mockRequest.path = options.path || | ||
(options.url ? url.parse(options.url).pathname : ''); | ||
mockRequest.path = options.path || (options.url ? url.parse(options.url).pathname : ''); | ||
mockRequest.params = options.params ? options.params : {}; | ||
@@ -90,10 +99,6 @@ if (options.session) { | ||
if (!mockRequest.query.hasOwnProperty) { | ||
Object.defineProperty( | ||
mockRequest.query, | ||
'hasOwnProperty', | ||
{ | ||
enumerable: false, | ||
value: Object.hasOwnProperty.bind(mockRequest.query) | ||
} | ||
); | ||
Object.defineProperty(mockRequest.query, 'hasOwnProperty', { | ||
enumerable: false, | ||
value: Object.hasOwnProperty.bind(mockRequest.query) | ||
}); | ||
} | ||
@@ -132,15 +137,15 @@ } | ||
*/ | ||
mockRequest.get = | ||
mockRequest.getHeader = | ||
mockRequest.header = function(name) { | ||
name = name.toLowerCase(); | ||
switch (name) { | ||
case 'referer': | ||
case 'referrer': | ||
return mockRequest.headers.referrer || mockRequest.headers.referer; | ||
default: | ||
return mockRequest.headers[name]; | ||
} | ||
}; | ||
mockRequest.getHeader = | ||
mockRequest.header = | ||
function (name) { | ||
name = name.toLowerCase(); | ||
switch (name) { | ||
case 'referer': | ||
case 'referrer': | ||
return mockRequest.headers.referrer || mockRequest.headers.referer; | ||
default: | ||
return mockRequest.headers[name]; | ||
} | ||
}; | ||
@@ -172,3 +177,3 @@ /** | ||
*/ | ||
mockRequest.is = function(types) { | ||
mockRequest.is = function (types) { | ||
if (!Array.isArray(types)) { | ||
@@ -201,3 +206,3 @@ types = [].slice.call(arguments); | ||
*/ | ||
mockRequest.accepts = function(types) { | ||
mockRequest.accepts = function (types) { | ||
var Accepts = accepts(mockRequest); | ||
@@ -214,4 +219,3 @@ return Accepts.type(types); | ||
*/ | ||
mockRequest.acceptsEncodings = function(encodings){ | ||
mockRequest.acceptsEncodings = function (encodings) { | ||
if (!Array.isArray(encodings)) { | ||
@@ -233,4 +237,3 @@ encodings = [].slice.call(arguments); | ||
*/ | ||
mockRequest.acceptsCharsets = function(charsets){ | ||
mockRequest.acceptsCharsets = function (charsets) { | ||
if (!Array.isArray(charsets)) { | ||
@@ -252,4 +255,3 @@ charsets = [].slice.call(arguments); | ||
*/ | ||
mockRequest.acceptsLanguages = function(languages){ | ||
mockRequest.acceptsLanguages = function (languages) { | ||
if (!Array.isArray(languages)) { | ||
@@ -289,3 +291,3 @@ languages = [].slice.call(arguments); | ||
*/ | ||
mockRequest.range = function(size, opts) { | ||
mockRequest.range = function (size, opts) { | ||
var range = mockRequest.get('Range'); | ||
@@ -307,3 +309,3 @@ if (!range) { | ||
*/ | ||
mockRequest.param = function(parameterName, defaultValue) { | ||
mockRequest.param = function (parameterName, defaultValue) { | ||
if (mockRequest.params.hasOwnProperty(parameterName)) { | ||
@@ -330,3 +332,3 @@ return mockRequest.params[parameterName]; | ||
*/ | ||
mockRequest._setParameter = function(key, value) { | ||
mockRequest._setParameter = function (key, value) { | ||
mockRequest.params[key] = value; | ||
@@ -341,3 +343,3 @@ }; | ||
*/ | ||
mockRequest._setSessionVariable = function(variable, value) { | ||
mockRequest._setSessionVariable = function (variable, value) { | ||
if (typeof mockRequest.session !== 'object') { | ||
@@ -355,3 +357,3 @@ mockRequest.session = {}; | ||
*/ | ||
mockRequest._setCookiesVariable = function(variable, value) { | ||
mockRequest._setCookiesVariable = function (variable, value) { | ||
mockRequest.cookies[variable] = value; | ||
@@ -366,3 +368,3 @@ }; | ||
*/ | ||
mockRequest._setSignedCookiesVariable = function(variable, value) { | ||
mockRequest._setSignedCookiesVariable = function (variable, value) { | ||
if (typeof mockRequest.signedCookies !== 'object') { | ||
@@ -380,3 +382,3 @@ mockRequest.signedCookies = {}; | ||
*/ | ||
mockRequest._setHeadersVariable = function(variable, value) { | ||
mockRequest._setHeadersVariable = function (variable, value) { | ||
variable = variable.toLowerCase(); | ||
@@ -392,3 +394,3 @@ mockRequest.headers[variable] = value; | ||
*/ | ||
mockRequest._setFilesVariable = function(variable, value) { | ||
mockRequest._setFilesVariable = function (variable, value) { | ||
mockRequest.files[variable] = value; | ||
@@ -409,3 +411,3 @@ }; | ||
*/ | ||
mockRequest._setMethod = function(method) { | ||
mockRequest._setMethod = function (method) { | ||
mockRequest.method = method; | ||
@@ -427,3 +429,3 @@ }; | ||
*/ | ||
mockRequest._setURL = function(value) { | ||
mockRequest._setURL = function (value) { | ||
mockRequest.url = value; | ||
@@ -445,3 +447,3 @@ }; | ||
*/ | ||
mockRequest._setBaseUrl = function(value) { | ||
mockRequest._setBaseUrl = function (value) { | ||
mockRequest.baseUrl = value; | ||
@@ -463,3 +465,3 @@ }; | ||
*/ | ||
mockRequest._setOriginalUrl = function(value) { | ||
mockRequest._setOriginalUrl = function (value) { | ||
mockRequest.originalUrl = value; | ||
@@ -488,3 +490,3 @@ }; | ||
*/ | ||
mockRequest._setBody = function(body) { | ||
mockRequest._setBody = function (body) { | ||
mockRequest.body = body; | ||
@@ -505,7 +507,6 @@ }; | ||
*/ | ||
mockRequest._addBody = function(key, value) { | ||
mockRequest._addBody = function (key, value) { | ||
mockRequest.body[key] = value; | ||
}; | ||
/** | ||
@@ -520,9 +521,8 @@ * Function: send | ||
*/ | ||
mockRequest.send = function(data) { | ||
if(Buffer.isBuffer(data)){ | ||
mockRequest.send = function (data) { | ||
if (Buffer.isBuffer(data)) { | ||
this.emit('data', data); | ||
}else if(typeof data === 'object' || typeof data === 'number'){ | ||
} else if (typeof data === 'object' || typeof data === 'number') { | ||
this.emit('data', Buffer.from(JSON.stringify(data))); | ||
}else if(typeof data === 'string'){ | ||
} else if (typeof data === 'string') { | ||
this.emit('data', Buffer.from(data)); | ||
@@ -540,3 +540,3 @@ } | ||
if (!mockRequest.hostname) { | ||
mockRequest.hostname = (function() { | ||
mockRequest.hostname = (function () { | ||
if (!mockRequest.headers.host) { | ||
@@ -548,3 +548,3 @@ return ''; | ||
return hostname.join('.'); | ||
}()); | ||
})(); | ||
} | ||
@@ -558,3 +558,3 @@ | ||
*/ | ||
mockRequest.subdomains = (function() { | ||
mockRequest.subdomains = (function () { | ||
if (!mockRequest.headers.host) { | ||
@@ -568,3 +568,3 @@ return []; | ||
return subdomains.slice(offset); | ||
}()); | ||
})(); | ||
@@ -584,7 +584,7 @@ /** | ||
let resolvePromise = null; | ||
const promiseExecutor = resolve => { | ||
const promiseExecutor = (resolve) => { | ||
resolvePromise = resolve; | ||
}; | ||
const promiseResolver = () => { | ||
@@ -596,3 +596,3 @@ if (resolvePromise) { | ||
}; | ||
const dataEventHandler = chunk => { | ||
const dataEventHandler = (chunk) => { | ||
if (ended || closed || error) { | ||
@@ -618,3 +618,3 @@ return; | ||
}; | ||
const errorEventHandler = err => { | ||
const errorEventHandler = (err) => { | ||
if (closed || error) { | ||
@@ -626,3 +626,3 @@ return; | ||
}; | ||
mockRequest.on('data', dataEventHandler); | ||
@@ -637,3 +637,3 @@ mockRequest.on('end', endEventHandler); | ||
}); | ||
try { | ||
@@ -650,3 +650,3 @@ for (;;) { | ||
} | ||
const hasChunks = i < chunks.length; | ||
@@ -661,3 +661,3 @@ if (!hasChunks) { | ||
} | ||
const chunk = chunks[i]; | ||
@@ -664,0 +664,0 @@ chunks[i] = undefined; |
@@ -37,3 +37,2 @@ 'use strict'; | ||
function createResponse(options) { | ||
if (!options) { | ||
@@ -81,4 +80,3 @@ options = {}; | ||
mockResponse.cookie = function(name, value, opt) { | ||
mockResponse.cookie = function (name, value, opt) { | ||
mockResponse.cookies[name] = { | ||
@@ -92,3 +90,3 @@ value: value, | ||
mockResponse.clearCookie = function(name, opt) { | ||
mockResponse.clearCookie = function (name, opt) { | ||
var opts = opt || {}; | ||
@@ -101,3 +99,3 @@ opts.expires = new Date(1); | ||
mockResponse.status = function(code) { | ||
mockResponse.status = function (code) { | ||
mockResponse.statusCode = code; | ||
@@ -118,4 +116,3 @@ return this; | ||
*/ | ||
mockResponse.writeHead = function(statusCode, statusMessage, headers) { | ||
mockResponse.writeHead = function (statusCode, statusMessage, headers) { | ||
if (_endCalled) { | ||
@@ -165,8 +162,5 @@ throw new Error('The end() method has already been called.'); | ||
*/ | ||
mockResponse.send = function(a, b, c) { | ||
var _formatData = function(data) { | ||
mockResponse.send = function (a, b, c) { | ||
var _formatData = function (data) { | ||
if (typeof data === 'object') { | ||
if (data.statusCode) { | ||
@@ -183,7 +177,5 @@ mockResponse.statusCode = data.statusCode; | ||
} | ||
} else { | ||
_data += data; | ||
} | ||
}; | ||
@@ -257,3 +249,2 @@ | ||
/** | ||
@@ -273,4 +264,3 @@ * Function: json | ||
*/ | ||
mockResponse.json = function(a, b) { | ||
mockResponse.json = function (a, b) { | ||
mockResponse.setHeader('Content-Type', 'application/json'); | ||
@@ -281,3 +271,3 @@ if (typeof a !== 'undefined') { | ||
mockResponse.write(JSON.stringify(b), 'utf8'); | ||
} else if(typeof b !== 'undefined' && typeof b === 'number') { | ||
} else if (typeof b !== 'undefined' && typeof b === 'number') { | ||
mockResponse.statusCode = b; | ||
@@ -309,4 +299,3 @@ mockResponse.write(JSON.stringify(a), 'utf8'); | ||
*/ | ||
mockResponse.jsonp = function(a, b) { | ||
mockResponse.jsonp = function (a, b) { | ||
mockResponse.setHeader('Content-Type', 'text/javascript'); | ||
@@ -317,3 +306,3 @@ if (typeof a !== 'undefined') { | ||
_data += JSON.stringify(b); | ||
} else if(typeof b !== 'undefined' && typeof b === 'number') { | ||
} else if (typeof b !== 'undefined' && typeof b === 'number') { | ||
mockResponse.statusCode = b; | ||
@@ -347,3 +336,3 @@ _data += JSON.stringify(a); | ||
*/ | ||
mockResponse.contentType = mockResponse.type = function(type) { | ||
mockResponse.contentType = mockResponse.type = function (type) { | ||
return mockResponse.set('Content-Type', type.indexOf('/') >= 0 ? type : mime.lookup(type)); | ||
@@ -360,3 +349,3 @@ }; | ||
*/ | ||
mockResponse.location = function(location) { | ||
mockResponse.location = function (location) { | ||
return mockResponse.set('Location', location); | ||
@@ -377,4 +366,3 @@ }; | ||
mockResponse.write = function(data, encoding) { | ||
mockResponse.write = function (data, encoding) { | ||
mockResponse.headersSent = true; | ||
@@ -392,5 +380,4 @@ | ||
} | ||
}; | ||
}; | ||
/** | ||
@@ -402,3 +389,3 @@ * Function: getEndArguments | ||
* https://nodejs.org/api/http.html#http_response_end_data_encoding_callback | ||
* | ||
* | ||
*/ | ||
@@ -418,3 +405,3 @@ function getEndArguments(args) { | ||
callback = args[1]; | ||
} else if (type === 'string' || args[1] instanceof String){ | ||
} else if (type === 'string' || args[1] instanceof String) { | ||
encoding = args[1]; | ||
@@ -434,3 +421,3 @@ } | ||
* the connection request. This must be called. | ||
* | ||
* | ||
* Signature: response.end([data[, encoding]][, callback]) | ||
@@ -440,3 +427,3 @@ * | ||
* | ||
* data - Optional data to return. Must be a string or Buffer instance. | ||
* data - Optional data to return. Must be a string or Buffer instance. | ||
* Appended to previous calls to <send>. | ||
@@ -447,3 +434,3 @@ * encoding - Optional encoding value. | ||
*/ | ||
mockResponse.end = function() { | ||
mockResponse.end = function () { | ||
if (_endCalled) { | ||
@@ -459,5 +446,5 @@ // Do not emit this event twice. | ||
_endCalled = true; | ||
var args = getEndArguments(arguments); | ||
if (args.data) { | ||
@@ -491,7 +478,7 @@ if (args.data instanceof Buffer) { | ||
} | ||
mockResponse.emit('end'); | ||
mockResponse.writableFinished = true; //Reference: https://nodejs.org/docs/latest-v12.x/api/http.html#http_request_writablefinished | ||
mockResponse.emit('finish'); | ||
if (args.callback) { | ||
@@ -501,3 +488,2 @@ args.callback(); | ||
}; | ||
@@ -514,12 +500,12 @@ /** | ||
*/ | ||
mockResponse.vary = function(fields) { | ||
mockResponse.vary = function (fields) { | ||
var header = mockResponse.getHeader('Vary') || ''; | ||
var values = header.length ? header.split(', ') : []; | ||
fields = Array.isArray(fields) ? fields : [ fields ]; | ||
fields = Array.isArray(fields) ? fields : [fields]; | ||
fields = fields.filter(function(field) { | ||
fields = fields.filter(function (field) { | ||
var regex = new RegExp(field, 'i'); | ||
var matches = values.filter(function(value) { | ||
var matches = values.filter(function (value) { | ||
return value.match(regex); | ||
@@ -538,5 +524,5 @@ }); | ||
* Set _Content-Disposition_ header to _attachment_ with optional `filename`. | ||
* | ||
* | ||
* Example: | ||
* | ||
* | ||
* res.attachment('download.csv') | ||
@@ -553,5 +539,5 @@ * | ||
} | ||
mockResponse.set('Content-Disposition', contentDisposition(filename)); | ||
return this; | ||
@@ -580,5 +566,3 @@ }; | ||
// concat the new and prev vals | ||
value = Array.isArray(prev) ? prev.concat(val) | ||
: Array.isArray(val) ? [prev].concat(val) | ||
: [prev, val]; | ||
value = Array.isArray(prev) ? prev.concat(val) : Array.isArray(val) ? [prev].concat(val) : [prev, val]; | ||
} | ||
@@ -616,3 +600,4 @@ | ||
return mockResponse.getHeader(field); | ||
} else { // eslint-disable-line | ||
} else { | ||
// eslint-disable-line | ||
for (var key in field) { | ||
@@ -630,3 +615,3 @@ mockResponse.setHeader(key, field[key]); | ||
*/ | ||
mockResponse.getHeaders = function() { | ||
mockResponse.getHeaders = function () { | ||
return JSON.parse(JSON.stringify(mockResponse._headers)); | ||
@@ -641,3 +626,3 @@ }; | ||
*/ | ||
mockResponse.get = mockResponse.getHeader = function(name) { | ||
mockResponse.get = mockResponse.getHeader = function (name) { | ||
return mockResponse._headers[name.toLowerCase()]; | ||
@@ -651,3 +636,3 @@ }; | ||
*/ | ||
mockResponse.getHeaderNames = function() { | ||
mockResponse.getHeaderNames = function () { | ||
return Object.keys(mockResponse._headers); // names are already stored in lowercase | ||
@@ -661,3 +646,3 @@ }; | ||
*/ | ||
mockResponse.hasHeader = function(name) { | ||
mockResponse.hasHeader = function (name) { | ||
return name.toLowerCase() in mockResponse._headers; | ||
@@ -672,3 +657,3 @@ }; | ||
*/ | ||
mockResponse.setHeader = function(name, value) { | ||
mockResponse.setHeader = function (name, value) { | ||
mockResponse._headers[name.toLowerCase()] = value; | ||
@@ -683,3 +668,3 @@ return this; | ||
*/ | ||
mockResponse.removeHeader = function(name) { | ||
mockResponse.removeHeader = function (name) { | ||
delete mockResponse._headers[name.toLowerCase()]; | ||
@@ -697,7 +682,7 @@ }; | ||
*/ | ||
mockResponse.setEncoding = function(encoding) { | ||
mockResponse.setEncoding = function (encoding) { | ||
_encoding = encoding; | ||
}; | ||
mockResponse.getEncoding = function() { | ||
mockResponse.getEncoding = function () { | ||
return _encoding; | ||
@@ -711,3 +696,3 @@ }; | ||
*/ | ||
mockResponse.redirect = function(a, b) { | ||
mockResponse.redirect = function (a, b) { | ||
switch (arguments.length) { | ||
@@ -738,3 +723,3 @@ case 1: | ||
*/ | ||
mockResponse.render = function(a, b, c) { | ||
mockResponse.render = function (a, b, c) { | ||
_renderView = a; | ||
@@ -777,3 +762,3 @@ | ||
*/ | ||
mockResponse.format = function(supported) { | ||
mockResponse.format = function (supported) { | ||
supported = supported || {}; | ||
@@ -815,7 +800,7 @@ var types = Object.keys(supported); | ||
mockResponse.destroy = function() { | ||
mockResponse.destroy = function () { | ||
return writableStream.destroy.apply(this, arguments); | ||
}; | ||
mockResponse.destroySoon = function() { | ||
mockResponse.destroySoon = function () { | ||
return writableStream.destroySoon.apply(this, arguments); | ||
@@ -833,3 +818,3 @@ }; | ||
*/ | ||
mockResponse._isEndCalled = function() { | ||
mockResponse._isEndCalled = function () { | ||
return _endCalled; | ||
@@ -844,3 +829,3 @@ }; | ||
*/ | ||
mockResponse._getHeaders = function() { | ||
mockResponse._getHeaders = function () { | ||
return mockResponse._headers; | ||
@@ -854,3 +839,3 @@ }; | ||
*/ | ||
mockResponse._getLocals = function() { | ||
mockResponse._getLocals = function () { | ||
return mockResponse.locals; | ||
@@ -864,3 +849,3 @@ }; | ||
*/ | ||
mockResponse._getData = function() { | ||
mockResponse._getData = function () { | ||
return _data; | ||
@@ -874,3 +859,3 @@ }; | ||
*/ | ||
mockResponse._getJSONData = function() { | ||
mockResponse._getJSONData = function () { | ||
return JSON.parse(_data); | ||
@@ -885,7 +870,6 @@ }; | ||
*/ | ||
mockResponse._getBuffer = function() { | ||
mockResponse._getBuffer = function () { | ||
return _buffer; | ||
}; | ||
/** | ||
@@ -897,3 +881,3 @@ * Function: _getChunks | ||
*/ | ||
mockResponse._getChunks = function() { | ||
mockResponse._getChunks = function () { | ||
return _chunks; | ||
@@ -907,3 +891,3 @@ }; | ||
*/ | ||
mockResponse._getStatusCode = function() { | ||
mockResponse._getStatusCode = function () { | ||
return mockResponse.statusCode; | ||
@@ -917,3 +901,3 @@ }; | ||
*/ | ||
mockResponse._getStatusMessage = function() { | ||
mockResponse._getStatusMessage = function () { | ||
return mockResponse.statusMessage; | ||
@@ -928,3 +912,3 @@ }; | ||
*/ | ||
mockResponse._isJSON = function() { | ||
mockResponse._isJSON = function () { | ||
return mockResponse.getHeader('Content-Type') === 'application/json'; | ||
@@ -943,3 +927,3 @@ }; | ||
*/ | ||
mockResponse._isUTF8 = function() { | ||
mockResponse._isUTF8 = function () { | ||
if (!_encoding) { | ||
@@ -963,3 +947,3 @@ return false; | ||
*/ | ||
mockResponse._isDataLengthValid = function() { | ||
mockResponse._isDataLengthValid = function () { | ||
if (mockResponse.getHeader('Content-Length')) { | ||
@@ -980,3 +964,3 @@ return mockResponse.getHeader('Content-Length').toString() === _data.length.toString(); | ||
*/ | ||
mockResponse._getRedirectUrl = function() { | ||
mockResponse._getRedirectUrl = function () { | ||
return _redirectUrl; | ||
@@ -994,3 +978,3 @@ }; | ||
*/ | ||
mockResponse._getRenderView = function() { | ||
mockResponse._getRenderView = function () { | ||
return _renderView; | ||
@@ -1008,3 +992,3 @@ }; | ||
*/ | ||
mockResponse._getRenderData = function() { | ||
mockResponse._getRenderData = function () { | ||
return _renderData; | ||
@@ -1014,5 +998,4 @@ }; | ||
return mockResponse; | ||
} | ||
module.exports.createResponse = createResponse; |
@@ -12,3 +12,3 @@ 'use strict'; | ||
enumerable: true, | ||
get: function() { | ||
get: function () { | ||
return true; | ||
@@ -15,0 +15,0 @@ } |
@@ -12,3 +12,2 @@ 'use strict'; | ||
function IncomingMessage() { | ||
@@ -46,7 +45,7 @@ Stream.Readable.call(this); | ||
IncomingMessage.prototype.read = function() {}; | ||
IncomingMessage.prototype._read = function() {}; | ||
IncomingMessage.prototype.destroy = function() {}; | ||
IncomingMessage.prototype.read = function () {}; | ||
IncomingMessage.prototype._read = function () {}; | ||
IncomingMessage.prototype.destroy = function () {}; | ||
IncomingMessage.prototype.setTimeout = function(msecs, callback) { | ||
IncomingMessage.prototype.setTimeout = function (msecs, callback) { | ||
if (callback) { | ||
@@ -57,3 +56,3 @@ setTimeout(callback, msecs); | ||
IncomingMessage.prototype._addHeaderLines = function(headers, n) { | ||
IncomingMessage.prototype._addHeaderLines = function (headers, n) { | ||
if (headers && headers.length) { | ||
@@ -79,3 +78,3 @@ var raw, dest; | ||
IncomingMessage.prototype._addHeaderLine = function(field, value, dest) { | ||
IncomingMessage.prototype._addHeaderLine = function (field, value, dest) { | ||
field = field.toLowerCase(); | ||
@@ -118,3 +117,3 @@ switch (field) { | ||
IncomingMessage.prototype._dump = function() { | ||
IncomingMessage.prototype._dump = function () { | ||
if (!this._dumped) { | ||
@@ -121,0 +120,0 @@ this._dumped = true; |
@@ -41,3 +41,3 @@ 'use strict'; | ||
417: 'Expectation Failed', | ||
418: 'I\'m a teapot', | ||
418: "I'm a teapot", | ||
422: 'Unprocessable Entity', | ||
@@ -44,0 +44,0 @@ 423: 'Locked', |
'use strict'; | ||
module.exports.convertKeysToLowerCase = function(map) { | ||
module.exports.convertKeysToLowerCase = function (map) { | ||
var newMap = {}; | ||
for(var key in map) { | ||
for (var key in map) { | ||
newMap[key.toLowerCase()] = map[key]; | ||
@@ -7,0 +7,0 @@ } |
{ | ||
"author": "Howard Abrams <howard.abrams@gmail.com> (http://www.github.com/howardabrams)", | ||
"name": "node-mocks-http", | ||
"description": "Mock 'http' objects for testing Express routing functions", | ||
"version": "1.14.0", | ||
"homepage": "https://github.com/howardabrams/node-mocks-http", | ||
"description": "Mock 'http' objects for testing Express, Next.js and Koa routing functions", | ||
"version": "1.14.1", | ||
"homepage": "https://github.com/eugef/node-mocks-http", | ||
"bugs": { | ||
"url": "https://github.com/howardabrams/node-mocks-http/issues" | ||
"url": "https://github.com/eugef/node-mocks-http/issues" | ||
}, | ||
@@ -20,2 +20,7 @@ "contributors": [ | ||
"url": "https://github.com/JohnnyEstilles" | ||
}, | ||
{ | ||
"name": "Eugene Fidelin", | ||
"email": "eugene.fidelin@gmail.com", | ||
"url": "https://github.com/eugef" | ||
} | ||
@@ -37,3 +42,3 @@ ], | ||
"type": "git", | ||
"url": "git://github.com/howardabrams/node-mocks-http.git" | ||
"url": "git://github.com/eugef/node-mocks-http.git" | ||
}, | ||
@@ -46,2 +51,4 @@ "main": "./lib/http-mock.js", | ||
"dependencies": { | ||
"@types/express": "^4.17.21", | ||
"@types/node": "^20.10.6", | ||
"accepts": "^1.3.7", | ||
@@ -60,5 +67,3 @@ "content-disposition": "^0.5.3", | ||
"@types/chai": "^4.3.11", | ||
"@types/express": "^4.17.17", | ||
"@types/mocha": "^10.0.6", | ||
"@types/node": "^14", | ||
"chai": "^4.2.0", | ||
@@ -70,4 +75,6 @@ "eslint": "^7.7.0", | ||
"gulp-mocha": "^8.0.0", | ||
"husky": "^8.0.3", | ||
"istanbul": "^0.4.5", | ||
"mocha": "^8.1.1", | ||
"prettier": "^3.1.1", | ||
"sinon": "^9.0.3", | ||
@@ -82,6 +89,9 @@ "sinon-chai": "^3.5.0", | ||
"spec:ts": "gulp spec:ts", | ||
"pretest": "prettier --check .", | ||
"test": "gulp test", | ||
"test:ts": "gulp test:ts", | ||
"types": "tsd . --files ./test/**/*.test-d.ts", | ||
"postversion": "npm publish && git push --follow-tags" | ||
"format": "prettier --write --list-different .", | ||
"postversion": "npm publish && git push --follow-tags", | ||
"prepare": "husky install" | ||
}, | ||
@@ -88,0 +98,0 @@ "files": [ |
175
README.md
[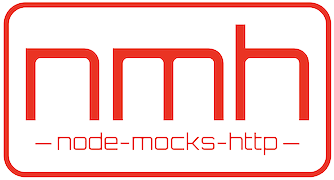](https://github.com/eugef/node-mocks-http) | ||
--- | ||
[![NPM version][npm-badge]][npm-url] | ||
Mock 'http' objects for testing [Express][express-url], [Next.js][nextjs-url] and [Koa][koa-url] routing functions, | ||
Mock 'http' objects for testing [Express][express-url], [Next.js][nextjs-url] and [Koa][koa-url] routing functions, | ||
but could be used for testing any [Node.js][node-url] web server applications that have code that requires mockups of the `request` and `response` objects. | ||
@@ -16,11 +16,15 @@ | ||
```bash | ||
$ npm install --save-dev node-mocks-http | ||
$ npm install node-mocks-http --save-dev | ||
``` | ||
> Our example includes `--save-dev` based on the assumption that **node-mocks-http** will be used as a development dependency. | ||
or | ||
```bash | ||
$ yarn add node-mocks-http --dev | ||
``` | ||
After installing the package include the following in your test files: | ||
```js | ||
var httpMocks = require('node-mocks-http'); | ||
const httpMocks = require('node-mocks-http'); | ||
``` | ||
@@ -39,3 +43,3 @@ | ||
```js | ||
var routeHandler = function( request, response ) { ... }; | ||
const routeHandler = function( request, response ) { ... }; | ||
``` | ||
@@ -47,28 +51,26 @@ | ||
```js | ||
exports['routeHandler - Simple testing'] = function(test) { | ||
var request = httpMocks.createRequest({ | ||
exports['routeHandler - Simple testing'] = function (test) { | ||
const request = httpMocks.createRequest({ | ||
method: 'GET', | ||
url: '/user/42', | ||
params: { | ||
id: 42 | ||
id: 42 | ||
} | ||
}); | ||
var response = httpMocks.createResponse(); | ||
const response = httpMocks.createResponse(); | ||
routeHandler(request, response); | ||
var data = response._getJSONData(); // short-hand for JSON.parse( response._getData() ); | ||
test.equal("Bob Dog", data.name); | ||
const data = response._getJSONData(); // short-hand for JSON.parse( response._getData() ); | ||
test.equal('Bob Dog', data.name); | ||
test.equal(42, data.age); | ||
test.equal("bob@dog.com", data.email); | ||
test.equal('bob@dog.com', data.email); | ||
test.equal(200, response.statusCode ); | ||
test.ok( response._isEndCalled()); | ||
test.ok( response._isJSON()); | ||
test.ok( response._isUTF8()); | ||
test.equal(200, response.statusCode); | ||
test.ok(response._isEndCalled()); | ||
test.ok(response._isJSON()); | ||
test.ok(response._isUTF8()); | ||
test.done(); | ||
}; | ||
@@ -82,10 +84,10 @@ ``` | ||
```ts | ||
it("should handle expressjs requests", () => { | ||
it('should handle expressjs requests', () => { | ||
const mockExpressRequest = httpMocks.createRequest({ | ||
method: 'GET', | ||
url: '/user/42', | ||
params: { | ||
id: 42 | ||
} | ||
}); | ||
method: 'GET', | ||
url: '/user/42', | ||
params: { | ||
id: 42 | ||
} | ||
}); | ||
const mockExpressResponse = httpMocks.createResponse(); | ||
@@ -96,10 +98,10 @@ | ||
const data = response._getJSONData(); | ||
test.equal("Bob Dog", data.name); | ||
test.equal('Bob Dog', data.name); | ||
test.equal(42, data.age); | ||
test.equal("bob@dog.com", data.email); | ||
test.equal('bob@dog.com', data.email); | ||
test.equal(200, response.statusCode ); | ||
test.ok( response._isEndCalled()); | ||
test.ok( response._isJSON()); | ||
test.ok( response._isUTF8()); | ||
test.equal(200, response.statusCode); | ||
test.ok(response._isEndCalled()); | ||
test.ok(response._isJSON()); | ||
test.ok(response._isUTF8()); | ||
@@ -115,10 +117,10 @@ test.done(); | ||
```ts | ||
it("should handle nextjs requests", () => { | ||
it('should handle nextjs requests', () => { | ||
const mockExpressRequest = httpMocks.createRequest<NextApiRequest>({ | ||
method: 'GET', | ||
url: '/user/42', | ||
params: { | ||
id: 42 | ||
} | ||
}); | ||
method: 'GET', | ||
url: '/user/42', | ||
params: { | ||
id: 42 | ||
} | ||
}); | ||
const mockExpressResponse = httpMocks.createResponse<NextApiResponse>(); | ||
@@ -131,2 +133,3 @@ | ||
## API | ||
### .createRequest() | ||
@@ -140,18 +143,18 @@ | ||
option | description | default value | ||
------ | ----------- | ------------- | ||
`method`| request HTTP method | 'GET' | ||
`url` | request URL | '' | ||
`originalUrl` | request original URL | `url` | ||
`baseUrl` | request base URL | `url` | ||
`path` | request path | '' | ||
`params` | object hash with params | {} | ||
`session` | object hash with session values | `undefined` | ||
`cookies` | object hash with request cookies | {} | ||
`socket` | object hash with request socket | {} | ||
`signedCookies` | object hash with signed cookies | `undefined` | ||
`headers` | object hash with request headers | {} | ||
`body` | object hash with body | {} | ||
`query` | object hash with query values | {} | ||
`files` | object hash with values | {} | ||
| option | description | default value | | ||
| --------------- | -------------------------------- | ------------- | | ||
| `method` | request HTTP method | 'GET' | | ||
| `url` | request URL | '' | | ||
| `originalUrl` | request original URL | `url` | | ||
| `baseUrl` | request base URL | `url` | | ||
| `path` | request path | '' | | ||
| `params` | object hash with params | {} | | ||
| `session` | object hash with session values | `undefined` | | ||
| `cookies` | object hash with request cookies | {} | | ||
| `socket` | object hash with request socket | {} | | ||
| `signedCookies` | object hash with signed cookies | `undefined` | | ||
| `headers` | object hash with request headers | {} | | ||
| `body` | object hash with body | {} | | ||
| `query` | object hash with query values | {} | | ||
| `files` | object hash with values | {} | | ||
@@ -163,3 +166,3 @@ The object returned from this function also supports the [Express request](http://expressjs.com/en/4x/api.html#req) functions ([`.accepts()`](http://expressjs.com/en/4x/api.html#req.accepts), [`.is()`](http://expressjs.com/en/4x/api.html#req.is), [`.get()`](http://expressjs.com/en/4x/api.html#req.get), [`.range()`](http://expressjs.com/en/4x/api.html#req.range), etc.). Please send a PR for any missing functions. | ||
```js | ||
httpMocks.createResponse(options) | ||
httpMocks.createResponse(options); | ||
``` | ||
@@ -169,12 +172,12 @@ | ||
option | description | default value | ||
------ | ----------- | ------------- | ||
`locals` | object that contains `response` local variables | `{}` | ||
`eventEmitter` | event emitter used by `response` object | `mockEventEmitter` | ||
`writableStream` | writable stream used by `response` object | `mockWritableStream` | ||
`req` | Request object being responded to | null | ||
| option | description | default value | | ||
| ---------------- | ----------------------------------------------- | -------------------- | | ||
| `locals` | object that contains `response` local variables | `{}` | | ||
| `eventEmitter` | event emitter used by `response` object | `mockEventEmitter` | | ||
| `writableStream` | writable stream used by `response` object | `mockWritableStream` | | ||
| `req` | Request object being responded to | null | | ||
> NOTE: The out-of-the-box mock event emitter included with `node-mocks-http` is | ||
not a functional event emitter and as such does not actually emit events. If you | ||
wish to test your event handlers you will need to bring your own event emitter. | ||
> not a functional event emitter and as such does not actually emit events. If you | ||
> wish to test your event handlers you will need to bring your own event emitter. | ||
@@ -184,4 +187,4 @@ > Here's an example: | ||
```js | ||
var httpMocks = require('node-mocks-http'); | ||
var res = httpMocks.createResponse({ | ||
const httpMocks = require('node-mocks-http'); | ||
const res = httpMocks.createResponse({ | ||
eventEmitter: require('events').EventEmitter | ||
@@ -203,31 +206,30 @@ }); | ||
```js | ||
var httpMocks = require('node-mocks-http'); | ||
var req = httpMocks.createRequest(); | ||
var res = httpMocks.createResponse({ | ||
eventEmitter: require('events').EventEmitter | ||
const httpMocks = require('node-mocks-http'); | ||
const req = httpMocks.createRequest(); | ||
const res = httpMocks.createResponse({ | ||
eventEmitter: require('events').EventEmitter | ||
}); | ||
// ... | ||
it('should do something', function(done) { | ||
res.on('end', function() { | ||
expect(response._getData()).to.equal('data sent in request'); | ||
done(); | ||
it('should do something', function (done) { | ||
res.on('end', function () { | ||
expect(response._getData()).to.equal('data sent in request'); | ||
done(); | ||
}); | ||
route(req,res); | ||
route(req, res); | ||
req.send('data sent in request'); | ||
}); | ||
}); | ||
function route(req,res){ | ||
var data= []; | ||
req.on("data", chunk => { | ||
data.push(chunk) | ||
function route(req, res) { | ||
let data = []; | ||
req.on('data', (chunk) => { | ||
data.push(chunk); | ||
}); | ||
req.on("end", () => { | ||
data = Buffer.concat(data) | ||
req.on('end', () => { | ||
data = Buffer.concat(data); | ||
res.write(data); | ||
res.end(); | ||
}); | ||
} | ||
@@ -240,3 +242,3 @@ // ... | ||
```js | ||
httpMocks.createMocks(reqOptions, resOptions) | ||
httpMocks.createMocks(reqOptions, resOptions); | ||
``` | ||
@@ -267,3 +269,2 @@ | ||
## Release Notes | ||
@@ -279,4 +280,3 @@ | ||
License | ||
--- | ||
## License | ||
@@ -287,3 +287,2 @@ Licensed under [MIT](LICENSE). | ||
[npm-url]: https://www.npmjs.com/package/node-mocks-http | ||
[express-url]: https://expressjs.com | ||
@@ -290,0 +289,0 @@ [nextjs-url]: https://nextjs.org |
77130
1.23%2057
0.64%12
20%277
-0.36%+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added