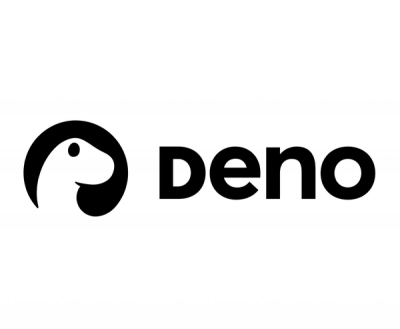
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
node-retrieve-globals
Advanced tools
Execute a string of JavaScript using Node.js and return the global variable values and functions.
Execute a string of JavaScript using Node.js and return the global variable values and functions.
vm
module to execute JavaScript
node:vm
module is not a security mechanism. Do not use it to run untrusted code.codeGeneration
(e.g. eval
) is disabled by default; use setCreateContextOptions({codeGeneration: { strings: true, wasm: true } })
to re-enable.Available on npm
npm install node-retrieve-globals
Works from Node.js with ESM and CommonJS:
import { RetrieveGlobals } from "node-retrieve-globals";
// const { RetrieveGlobals } = require("node-retrieve-globals");
And then:
let code = `var a = 1;
const b = "hello";
function hello() {}`;
let vm = new RetrieveGlobals(code);
await vm.getGlobalContext();
// or sync:
// vm.getGlobalContextSync();
Returns:
{ a: 1, b: "hello", hello: function hello() {} }
let code = `let ref = myData;`;
let vm = new RetrieveGlobals(code);
await vm.getGlobalContext({ myData: "hello" });
// or sync:
// vm.getGlobalContextSync({ myData: "hello" });
Returns:
{ ref: "hello" }
// Defaults shown
let options = {
reuseGlobal: false, // re-use Node.js `global`, important if you want `console.log` to log to your console as expected.
dynamicImport: false, // allows `import()`
};
await vm.getGlobalContext({}, options);
FAQs
Execute a string of JavaScript using Node.js and return the global variable values and functions.
The npm package node-retrieve-globals receives a total of 31,457 weekly downloads. As such, node-retrieve-globals popularity was classified as popular.
We found that node-retrieve-globals demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.