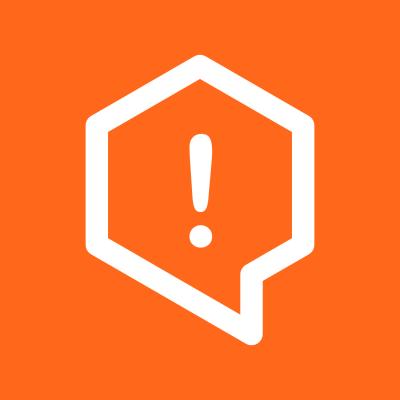
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
nodejs-helpers
Advanced tools
A set of classes to simplify interaction with Google Apis.
Install Node.js v8.9.2 or greater
Set the GCLOUD_PROJECT
environment variable:
Linux:
export GCLOUD_PROJECT=your-project-id
Windows:
set GCLOUD_PROJECT=your-project-id
Windows (PowerShell):
$env:GCLOUD_PROJECT="your-project-id"
Obtain authentication credentials.
Create local credentials by running the following command and following the oauth2 flow (read more about the command here):
gcloud auth application-default login
In non-Google Cloud environments, GCE instances created without the
correct scopes, or local workstations where the
gcloud beta auth application-default login
command fails, use a service
account by doing the following:
GOOGLE_APPLICATION_CREDENTIALS
environment variable to point to the file containing the JSON credentials.Set the GOOGLE_APPLICATION_CREDENTIALS
environment variable:
Linux:
export GOOGLE_APPLICATION_CREDENTIALS=/path/to/service_account_file.json
Windows:
set GOOGLE_APPLICATION_CREDENTIALS=/path/to/service_account_file.json
Windows (PowerShell):
$env:GOOGLE_APPLICATION_CREDENTIALS="/path/to/service_account_file.json"
Note for code running on GCE, GAE, or other environments:
On Google App Engine, the credentials should be found automatically.
On Google Compute Engine, the credentials should be found automatically, but require that you create the instance with the correct scopes.
gcloud compute instances create --scopes="https://www.googleapis.com/auth/cloud-platform,https://www.googleapis.com/auth/compute,https://www.googleapis.com/auth/compute.readonly" test-instance
If you did not create the instance with the right scopes, you can still
upload a JSON service account and set GOOGLE_APPLICATION_CREDENTIALS
as described.
Read more about Google Cloud Platform Authentication.
Using Promises
const { MessageTransport } = require("nodejs-helpers");
const topicName = "test-jal",
data = { jal: "test" },
attributes = { attr1: "1", attr2: "2" },
transport = new MessageTransport(topicName);
transport.publish(data, attributes)
.then((response) => {
console.log(response);
// 45031755284181 // messageId of the published message
})
.catch((err) => {
console.error(err);
});
Using async/await
const { MessageTransport } = require("nodejs-helpers");
async function testMessageTransport() {
try {
const topicName = "test-jal",
data = { jal: "test" },
attributes = { attr1: "1", attr2: "2" }
const transport = new MessageTransport(topicName);
const response = await transport.publish(data, attributes);
console.log(response);
// 45031755284181 // messageId of the published message
} catch (err) {
console.error(err);
}
}
(async() => {
await testMessageTransport();
})();
Using Promises
const { StorageProvider } = require("nodejs-helpers");
const storage = new StorageProvider({
forBigQuery: true,
bucketName: "now-ims-core-dev_bigquery-staging"
});
storage.save("test_jal/jal.txt", "hey")
.then((response) => {
console.log(response);
// { success: true, payload: '"h"\n"e"\n"y"' }
})
.catch((err) => {
console.error(err);
});
storage.save("test_jal/jal.txt", "hey", { forBigQuery: false })
.then((response) => {
console.log(response);
// { success: true, payload: 'hey' }
})
.catch((err) => {
console.error(err);
});
Using async/await
const { StorageProvider } = require("nodejs-helpers");
async function testStorageProvider() {
try {
const storage = new StorageProvider({
forBigQuery: true,
bucketName: "my-testing-bucket"
});
let response = await storage.save("test_jal/jal.txt", "hey");
console.log(response);
// { success: true, payload: '"h"\n"e"\n"y"' }
response = await storage.save("test_jal/jal.txt", "hey", { forBigQuery: false });
console.log(response);
// { success: true, payload: 'hey' }
} catch (err) {
console.error(err);
}
}
(async() => {
await testStorageProvider();
})();
const { Logger } = require("nodejs-helpers");
const logger = new Logger();
logger.debug("debug");
// 2018-02-28T05:21:53.214Z - debug: debug
logger.info("info");
// 2018-02-28T05:21:53.217Z - info: info
logger.error("error");
// 2018-02-28T05:21:53.218Z - error: error
FAQs
A set of classes to simplify interaction with Google Apis.
We found that nodejs-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.