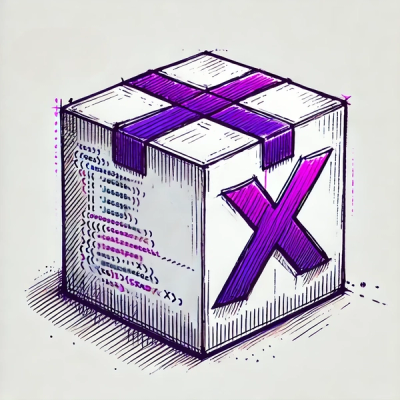
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
nodemailer-smtp-transport
Advanced tools
The nodemailer-smtp-transport package is a transport module for Nodemailer that allows you to send emails using the SMTP protocol. It provides a way to configure and use SMTP servers to send emails programmatically.
Basic SMTP Transport Configuration
This code demonstrates how to configure and use the nodemailer-smtp-transport package to send an email using an SMTP server. It sets up the SMTP transport with the necessary authentication details and sends an email with both text and HTML content.
const nodemailer = require('nodemailer');
const smtpTransport = require('nodemailer-smtp-transport');
const transporter = nodemailer.createTransport(smtpTransport({
host: 'smtp.example.com',
port: 587,
secure: false, // true for 465, false for other ports
auth: {
user: 'username',
pass: 'password'
}
}));
const mailOptions = {
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world',
html: '<b>Hello world</b>'
};
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
return console.log(error);
}
console.log('Message sent: %s', info.messageId);
});
Using OAuth2 for Authentication
This code demonstrates how to use OAuth2 for authentication with the nodemailer-smtp-transport package. It configures the transport with OAuth2 credentials and sends an email using Gmail's SMTP server.
const nodemailer = require('nodemailer');
const smtpTransport = require('nodemailer-smtp-transport');
const transporter = nodemailer.createTransport(smtpTransport({
service: 'gmail',
auth: {
type: 'OAuth2',
user: 'user@example.com',
clientId: 'your-client-id',
clientSecret: 'your-client-secret',
refreshToken: 'your-refresh-token',
accessToken: 'your-access-token'
}
}));
const mailOptions = {
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world',
html: '<b>Hello world</b>'
};
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
return console.log(error);
}
console.log('Message sent: %s', info.messageId);
});
Nodemailer is a module for Node.js applications to allow easy email sending. It supports various transport methods, including SMTP, and can be used directly without the need for additional transport modules like nodemailer-smtp-transport. It is more versatile and widely used.
EmailJS is a library for sending emails from JavaScript applications. It supports SMTP and provides a simple API for sending emails. Compared to nodemailer-smtp-transport, EmailJS is more lightweight but may lack some advanced features and flexibility.
SendGrid is a cloud-based service that provides an API for sending emails. It offers robust features like email tracking, analytics, and high deliverability. Unlike nodemailer-smtp-transport, which requires an SMTP server, SendGrid handles the email delivery infrastructure for you.
SMTP module with for Nodemailer.
See Nodemailer homepage for documentation and terms of using SMTP.
FAQs
SMTP transport for Nodemailer
The npm package nodemailer-smtp-transport receives a total of 82,796 weekly downloads. As such, nodemailer-smtp-transport popularity was classified as popular.
We found that nodemailer-smtp-transport demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.