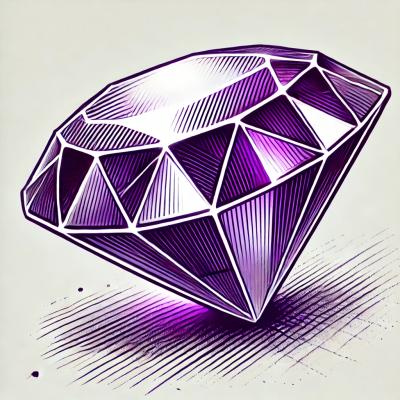
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
normalize-url
Advanced tools
The normalize-url npm package is used to normalize and sanitize URLs to ensure they have a standard and consistent format. It can be used to remove tracking parameters, sort query parameters, remove default ports, and more.
Normalizing URLs
This feature allows you to normalize a URL by converting it to a standard format. It can remove or add 'http://', sort query parameters, and convert the hostname to lowercase.
"use strict";
const normalizeUrl = require('normalize-url');
console.log(normalizeUrl('sindresorhus.com')); // Output: 'http://sindresorhus.com'
console.log(normalizeUrl('HTTP://xn--xample-hva.com:80/?b=bar&a=foo')); // Output: 'http://êxample.com/?a=foo&b=bar'"
Removing URL Tracking Parameters
This feature allows you to remove URL tracking parameters like 'utm_source' and 'utm_medium' by using a regular expression.
"use strict";
const normalizeUrl = require('normalize-url');
console.log(normalizeUrl('www.sindresorhus.com?utm_source=foo&utm_medium=bar', {removeQueryParameters: [/^utm_/]})); // Output: 'http://sindresorhus.com'"
Removing Default Ports
This feature removes the default ports from URLs (port 80 for HTTP and port 443 for HTTPS).
"use strict";
const normalizeUrl = require('normalize-url');
console.log(normalizeUrl('http://sindresorhus.com:80')); // Output: 'http://sindresorhus.com'
console.log(normalizeUrl('https://sindresorhus.com:443')); // Output: 'https://sindresorhus.com'"
Forcing HTTPS
This feature allows you to force the URL to use HTTPS instead of HTTP.
"use strict";
const normalizeUrl = require('normalize-url');
console.log(normalizeUrl('http://sindresorhus.com', {forceHttps: true})); // Output: 'https://sindresorhus.com'"
The url-parse package offers similar URL parsing and normalization functionalities. It provides a more detailed breakdown of URL components and allows manipulation of these components individually.
URI.js is a library for working with URLs. It includes URL manipulation and normalization features, but also offers more extensive options for working with URIs, such as resolving URIs, managing URI components, and building URIs from templates.
Normalize a URL
Useful when you need to display, store, deduplicate, sort, compare, etc, URLs.
Note: This package does not do URL sanitization. Garbage in, garbage out. If you use this in a server context and accept URLs as user input, it's up to you to protect against invalid URLs, path traversal attacks, etc.
npm install normalize-url
If you need Safari support, use version 4: npm i normalize-url@4
import normalizeUrl from 'normalize-url';
normalizeUrl('sindresorhus.com');
//=> 'http://sindresorhus.com'
normalizeUrl('//www.sindresorhus.com:80/../baz?b=bar&a=foo');
//=> 'http://sindresorhus.com/baz?a=foo&b=bar'
URLs with custom protocols are not normalized and just passed through by default. Supported protocols are: https
, http
, file
, and data
.
Type: string
URL to normalize, including data URL.
Type: object
Type: string
Default: 'http'
Values: 'https' | 'http'
Type: boolean
Default: true
Prepend defaultProtocol
to the URL if it's protocol-relative.
normalizeUrl('//sindresorhus.com');
//=> 'http://sindresorhus.com'
normalizeUrl('//sindresorhus.com', {normalizeProtocol: false});
//=> '//sindresorhus.com'
Type: boolean
Default: false
Normalize HTTPS to HTTP.
normalizeUrl('https://sindresorhus.com');
//=> 'https://sindresorhus.com'
normalizeUrl('https://sindresorhus.com', {forceHttp: true});
//=> 'http://sindresorhus.com'
Type: boolean
Default: false
Normalize HTTP to HTTPS.
normalizeUrl('http://sindresorhus.com');
//=> 'http://sindresorhus.com'
normalizeUrl('http://sindresorhus.com', {forceHttps: true});
//=> 'https://sindresorhus.com'
This option cannot be used with the forceHttp
option at the same time.
Type: boolean
Default: true
Strip the authentication part of the URL.
normalizeUrl('user:password@sindresorhus.com');
//=> 'https://sindresorhus.com'
normalizeUrl('user:password@sindresorhus.com', {stripAuthentication: false});
//=> 'https://user:password@sindresorhus.com'
Type: boolean
Default: false
Strip the hash part of the URL.
normalizeUrl('sindresorhus.com/about.html#contact');
//=> 'http://sindresorhus.com/about.html#contact'
normalizeUrl('sindresorhus.com/about.html#contact', {stripHash: true});
//=> 'http://sindresorhus.com/about.html'
Type: boolean
Default: false
Remove the protocol from the URL: http://sindresorhus.com
→ sindresorhus.com
.
It will only remove https://
and http://
protocols.
normalizeUrl('https://sindresorhus.com');
//=> 'https://sindresorhus.com'
normalizeUrl('https://sindresorhus.com', {stripProtocol: true});
//=> 'sindresorhus.com'
Type: boolean
Default: true
Strip the text fragment part of the URL.
Note: The text fragment will always be removed if the stripHash
option is set to true
, as the hash contains the text fragment.
normalizeUrl('http://sindresorhus.com/about.html#:~:text=hello');
//=> 'http://sindresorhus.com/about.html#'
normalizeUrl('http://sindresorhus.com/about.html#section:~:text=hello');
//=> 'http://sindresorhus.com/about.html#section'
normalizeUrl('http://sindresorhus.com/about.html#:~:text=hello', {stripTextFragment: false});
//=> 'http://sindresorhus.com/about.html#:~:text=hello'
normalizeUrl('http://sindresorhus.com/about.html#section:~:text=hello', {stripTextFragment: false});
//=> 'http://sindresorhus.com/about.html#section:~:text=hello'
Type: boolean
Default: true
Remove www.
from the URL.
normalizeUrl('http://www.sindresorhus.com');
//=> 'http://sindresorhus.com'
normalizeUrl('http://www.sindresorhus.com', {stripWWW: false});
//=> 'http://www.sindresorhus.com'
Type: Array<RegExp | string> | boolean
Default: [/^utm_\w+/i]
Remove query parameters that matches any of the provided strings or regexes.
normalizeUrl('www.sindresorhus.com?foo=bar&ref=test_ref', {
removeQueryParameters: ['ref']
});
//=> 'http://sindresorhus.com/?foo=bar'
If a boolean is provided, true
will remove all the query parameters.
normalizeUrl('www.sindresorhus.com?foo=bar', {
removeQueryParameters: true
});
//=> 'http://sindresorhus.com'
false
will not remove any query parameter.
normalizeUrl('www.sindresorhus.com?foo=bar&utm_medium=test&ref=test_ref', {
removeQueryParameters: false
});
//=> 'http://www.sindresorhus.com/?foo=bar&ref=test_ref&utm_medium=test'
Type: Array<RegExp | string>
Default: undefined
Keeps only query parameters that matches any of the provided strings or regexes.
Note: It overrides the removeQueryParameters
option.
normalizeUrl('https://sindresorhus.com?foo=bar&ref=unicorn', {
keepQueryParameters: ['ref']
});
//=> 'https://sindresorhus.com/?ref=unicorn'
Type: boolean
Default: true
Remove trailing slash.
Note: Trailing slash is always removed if the URL doesn't have a pathname unless the removeSingleSlash
option is set to false
.
normalizeUrl('http://sindresorhus.com/redirect/');
//=> 'http://sindresorhus.com/redirect'
normalizeUrl('http://sindresorhus.com/redirect/', {removeTrailingSlash: false});
//=> 'http://sindresorhus.com/redirect/'
normalizeUrl('http://sindresorhus.com/', {removeTrailingSlash: false});
//=> 'http://sindresorhus.com'
Type: boolean
Default: true
Remove a sole /
pathname in the output. This option is independent of removeTrailingSlash
.
normalizeUrl('https://sindresorhus.com/');
//=> 'https://sindresorhus.com'
normalizeUrl('https://sindresorhus.com/', {removeSingleSlash: false});
//=> 'https://sindresorhus.com/'
Type: boolean | Array<RegExp | string>
Default: false
Removes the default directory index file from path that matches any of the provided strings or regexes. When true
, the regex /^index\.[a-z]+$/
is used.
normalizeUrl('www.sindresorhus.com/foo/default.php', {
removeDirectoryIndex: [/^default\.[a-z]+$/]
});
//=> 'http://sindresorhus.com/foo'
Type: boolean
Default: false
Removes an explicit port number from the URL.
Port 443 is always removed from HTTPS URLs and 80 is always removed from HTTP URLs regardless of this option.
normalizeUrl('sindresorhus.com:123', {
removeExplicitPort: true
});
//=> 'http://sindresorhus.com'
Type: boolean
Default: true
Sorts the query parameters alphabetically by key.
normalizeUrl('www.sindresorhus.com?b=two&a=one&c=three', {
sortQueryParameters: false
});
//=> 'http://sindresorhus.com/?b=two&a=one&c=three'
FAQs
Normalize a URL
The npm package normalize-url receives a total of 4,984,211 weekly downloads. As such, normalize-url popularity was classified as popular.
We found that normalize-url demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.