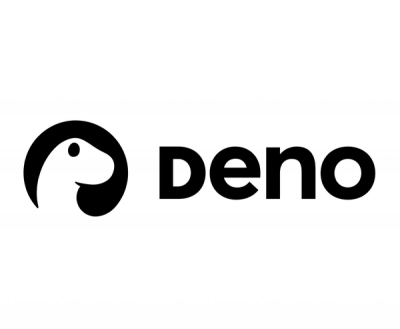
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
notepack.io
Advanced tools
notepack.io is a fast and efficient implementation of the MessagePack serialization format for JavaScript. It is designed to serialize and deserialize data quickly, making it suitable for performance-critical applications such as real-time data transmission and storage.
Serialization
This feature allows you to serialize JavaScript objects into a compact binary format using MessagePack. The code sample demonstrates how to encode a simple object.
const notepack = require('notepack.io');
const data = { key: 'value' };
const serialized = notepack.encode(data);
console.log(serialized);
Deserialization
This feature allows you to deserialize MessagePack binary data back into JavaScript objects. The code sample shows how to decode a binary buffer into an object.
const notepack = require('notepack.io');
const serialized = Buffer.from([129, 163, 107, 101, 121, 165, 118, 97, 108, 117, 101]);
const deserialized = notepack.decode(serialized);
console.log(deserialized);
msgpack-lite is another implementation of the MessagePack format for JavaScript. It is known for its simplicity and ease of use, but it may not be as fast as notepack.io in certain scenarios.
msgpack5 is a versatile MessagePack implementation that supports both Node.js and browser environments. It offers a good balance between performance and feature set, making it a strong alternative to notepack.io.
msgpack is the official MessagePack library for JavaScript. While it is reliable and well-maintained, it may not offer the same level of performance optimization as notepack.io.
A fast Node.js implementation of the latest MessagePack spec.
undefined
is encoded as fixext 1 [0, 0]
, i.e. <Buffer d4 00 00>
Date
objects are encoded as fixext 8 [0, ms]
, e.g. new Date('2000-06-13T00:00:00.000Z')
=> <Buffer d7 00 00 00 00 df b7 62 9c 00>
ArrayBuffer
are encoded as ext 8/16/32 [0, data]
, e.g. Uint8Array.of(1, 2, 3, 4)
=> <Buffer c7 04 00 01 02 03 04>
npm install notepack.io
var notepack = require('notepack.io');
var encoded = notepack.encode({ foo: 'bar'}); // <Buffer 81 a3 66 6f 6f a3 62 61 72>
var decoded = notepack.decode(encoded); // { foo: 'bar' }
A browser version of notepack is also available (7.6 kB minified)
<script src="https://rawgit.com/darrachequesne/notepack/master/dist/notepack.js"></script>
<script>
console.log(notepack.decode(notepack.encode([1, '2', new Date()])));
// [1, "2", Thu Dec 08 2016 00:00:01 GMT+0100 (CET)]
</script>
Performance is currently comparable to msgpack-node (which presumably needs optimizing and suffers from JS-native overhead) and is significantly faster than other implementations. Several micro-optimizations are used to improve the performance of short string and Buffer operations.
The ./benchmarks/run
output on my machine is:
$ node -v
v6.9.1
$ ./benchmarks/run
Encoding (this will take a while):
+----------------------------+-------------------+-----------------+----------------+---------------+
| │ tiny │ small │ medium │ large |
+----------------------------+-------------------+-----------------+----------------+---------------+
| notepack │ 1,118,284 ops/sec │ 290,050 ops/sec │ 19,670 ops/sec │ 146 ops/sec |
+----------------------------+-------------------+-----------------+----------------+---------------+
| msgpack-js │ 116,413 ops/sec │ 29,831 ops/sec │ 3,199 ops/sec │ 57.73 ops/sec |
+----------------------------+-------------------+-----------------+----------------+---------------+
| msgpack-lite │ 229,063 ops/sec │ 92,016 ops/sec │ 12,620 ops/sec │ 169 ops/sec |
+----------------------------+-------------------+-----------------+----------------+---------------+
| JSON.stringify (to Buffer) │ 811,373 ops/sec │ 111,166 ops/sec │ 2,556 ops/sec │ 2.83 ops/sec |
+----------------------------+-------------------+-----------------+----------------+---------------+
Decoding (this will take a while):
+--------------------------+-------------------+-----------------+----------------+---------------+
| │ tiny │ small │ medium │ large |
+--------------------------+-------------------+-----------------+----------------+---------------+
| notepack │ 860,763 ops/sec │ 179,459 ops/sec │ 17,642 ops/sec │ 184 ops/sec |
+--------------------------+-------------------+-----------------+----------------+---------------+
| msgpack-js │ 522,966 ops/sec │ 132,121 ops/sec │ 12,445 ops/sec │ 179 ops/sec |
+--------------------------+-------------------+-----------------+----------------+---------------+
| msgpack-lite │ 566,976 ops/sec │ 114,859 ops/sec │ 9,520 ops/sec │ 156 ops/sec |
+--------------------------+-------------------+-----------------+----------------+---------------+
| JSON.parse (from Buffer) │ 1,177,912 ops/sec │ 277,076 ops/sec │ 18,430 ops/sec │ 38.74 ops/sec |
+--------------------------+-------------------+-----------------+----------------+---------------+
* Note that JSON is provided as an indicative comparison only
MIT
FAQs
A fast Node.js implementation of the latest MessagePack spec
The npm package notepack.io receives a total of 317,370 weekly downloads. As such, notepack.io popularity was classified as popular.
We found that notepack.io demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.