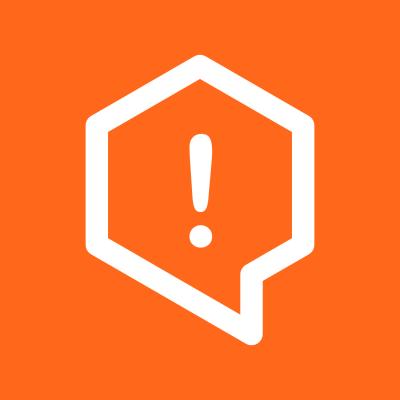
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
The Nuance UI Framework provides a set of React components for building modern user interfaces.
Welcome to the documentation for the Nuance UI Framework! This framework provides a set of React components for building user interfaces with a consistent and stylish look. Below is a detailed guide on how to use various components included in the Nuance UI Framework.
npm install nuanceui
import { NSpinner } from "./NuanceUI";
<NSpinner />;
The NSpinner
component displays a spinner while content is loading.
import { NButton, NToast } from "./NuanceUI";
const App = () => {
const [isToastVisible, setToastVisible] = useState(false);
const showToast = () => {
setToastVisible(true);
setTimeout(() => {
setToastVisible(false);
}, 3000);
};
return (
<div>
<h2>Toast</h2>
<NButton onClick={showToast}>Show Toast</NButton>
{isToastVisible && <NToast message="This is a toast message!" />}
</div>
);
};
The NToast
component displays a toast message with a customizable duration.
import { NDropdownMenu } from "./NuanceUI";
const App = () => {
const [selectedOption, setSelectedOption] =
(useState < string) | (null > null);
const options = ["Option 1", "Option 2"];
const handleSelectionChange = (newSelectedOption: string | null) => {
setSelectedOption(newSelectedOption);
};
return (
<div>
<h2>Dropdown Menu</h2>
<NDropdownMenu
options={options}
selectedOption={selectedOption}
onSelectionChange={handleSelectionChange}
/>
<p>Selected Option: {selectedOption || "None"}</p>
</div>
);
};
The NDropdownMenu
component provides a dropdown menu with customizable options.
import { NDatePicker } from "./NuanceUI";
const App = () => {
const [selectedDate, setSelectedDate] = (useState < Date) | (null > null);
const handleDateChange = (date: Date) => {
setSelectedDate(date);
};
return (
<div>
<h2>Date Time Picker</h2>
<NDatePicker onDateChange={handleDateChange} />
<p>
Selected Date: {selectedDate ? selectedDate.toDateString() : "None"}
</p>
</div>
);
};
The NDatePicker
component allows users to select a date from a calendar.
import { NDataGrid } from "./NuanceUI";
const App = () => {
const columns = [
{ field: "OrderID", width: "100px", textAlign: "center" },
// ... additional columns
];
const customerData = [
{
OrderID: 10248,
CustomerID: "VINET",
EmployeeID: 5,
Freight: 32.38,
ShipCountry: "Germany",
OrderDate: "1996-07-04T00:00:00.000Z",
Discount: 0.15,
},
// ... additional rows
];
return (
<div>
<h2>Data Grid</h2>
<NDataGrid dataSource={customerData} columns={columns} itemsPerPage={4} />
</div>
);
};
The NDataGrid
component displays tabular data with customizable columns.
import { NButton, NDialogBox } from "./NuanceUI";
const App = () => {
const [isDialogOpen, setIsDialogOpen] = useState(false);
const openDialog = () => {
setIsDialogOpen(true);
};
const closeDialog = () => {
setIsDialogOpen(false);
};
return (
<div>
<h2>Dialog</h2>
<NButton onClick={openDialog}>Open Dialog</NButton>
<NDialogBox
isOpen={isDialogOpen}
onClose={closeDialog}
title="Sample Dialog"
>
This is the content of the dialog box.
</NDialogBox>
</div>
);
};
The NDialogBox
component creates a customizable dialog box.
import { NButton, NFlexGroup, NFlexItem } from "./NuanceUI";
const App = () => {
const handleClick = () => {
throw new Error("Function not implemented.");
};
return (
<div>
<h2>Button</h2>
<NFlexGroup justify="space-between">
<NFlexItem>
<NButton onClick={handleClick} color="primary">
Primary
</NButton>
</NFlexItem>
{/* ... additional buttons */}
</NFlexGroup>
</div>
);
};
The NButton
component creates a customizable button with various color options.
import { NTextField } from "./NuanceUI";
const App = () => {
const [email, setEmail] = useState("");
const handleOnChange = (event: any) => {
setEmail(event.target.value);
};
return (
<div>
<h2>TextField</h2>
<NTextField
id="myTextField"
value={email}
onChange={handleOnChange}
placeholder="Enter your name"
/>
{/* ... additional text fields */}
</div>
);
};
The NTextField
component creates a customizable text input field.
import { NRadioButton, NCheckBox } from "./NuanceUI";
const App = () => {
const [isChecked, setIsChecked] = useState(false);
const [selectedOption, setSelectedOption] =
(useState < string) | (null > null);
const handleCheckboxChange = (event: React.ChangeEvent<HTMLInputElement>) => {
setIsChecked(event.target.checked);
};
const handleRadioChange = (value: string) => {
setSelectedOption(value);
};
return (
<div>
<h2>Radio & Checkbox</h2>
<NRadioButton
label="Option 1"
value="option1"
checked={selectedOption === "option1"}
onChange={handleRadioChange}
/>
<NRadioButton
label="Option 2"
value="option2"
checked={selectedOption === "option2"}
onChange={handleRadioChange}
/>
<p>Selected Option: {selectedOption}</p>
<NCheckBox
id="checkbox1"
checked={isChecked}
onChange={handleCheckboxChange}
label="Check me"
/>
</div>
);
};
The NRadioButton
and NCheckBox
components create customizable radio buttons and checkboxes, respectively.
FAQs
The Nuance UI Framework provides a set of React components for building modern user interfaces.
The npm package nuanceui receives a total of 0 weekly downloads. As such, nuanceui popularity was classified as not popular.
We found that nuanceui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.