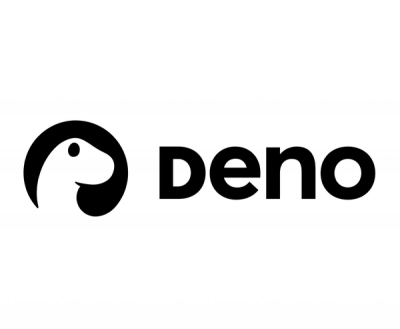
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
nue is an async control-flow library.
JavaScript
var nue = require('nue');
step1();
function step1() {
console.log('step1 start');
nue.parallel([
function(){
console.log('aaa');
},
function(){
console.log('bbb');
}],
function(err){
if (err) throw err;
console.log('step1 end\n');
step2();
}
);
}
function step2() {
console.log('step2 start');
nue.series([
function () {
console.log('ccc');
this.next('test', 2);
},
function (a, b){
console.log('ddd ' + a + b);
this.next();
}],
function (err) {
if (err) throw err;
console.log('step2 end\n');
step3();
}
);
}
function step3() {
console.log("step3 start");
var q = nue.parallelQueue(
function (data){
console.log('data: ' + data);
},
function (err) {
if (err) throw err;
console.log('step3 end\n');
step4();
}
);
for (var i = 0; i < 10; i++) {
q.push(i);
}
q.complete();
}
function step4() {
console.log("step4 start");
var q = nue.seriesQueue(
function (data){
console.log('data: ' + data);
this.next();
},
function (err) {
if (err) throw err;
console.log('step4 end\n');
}
);
for (var i = 0; i < 10; i++) {
q.push(i);
}
q.complete();
}
Result
step1 start
aaa:
bbb:
step1 end
step2 start
ccc:
ddd: test, 2
step2 end
step3 start
data: 0
data: 1
data: 2
data: 3
data: 4
step3 end
step4 start
data: 0
data: 1
data: 2
data: 3
data: 4
step4 end
FAQs
An async control-flow library suited for node.js.
The npm package nue receives a total of 1,413 weekly downloads. As such, nue popularity was classified as popular.
We found that nue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.