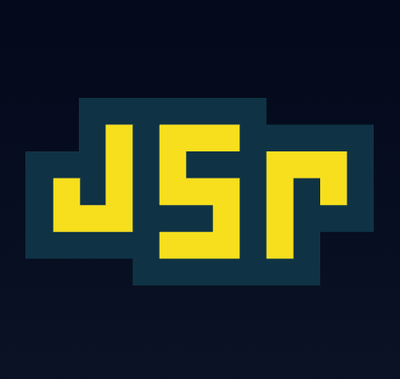
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Nunjucks is a powerful templating engine for JavaScript, inspired by Jinja2. It is designed to be fast, extendable, and easy to use, making it suitable for both server-side and client-side applications.
Template Rendering
Nunjucks allows you to render templates with dynamic data. In this example, the template 'Hello {{ name }}!' is rendered with the context { name: 'World' }, resulting in 'Hello World!'.
const nunjucks = require('nunjucks');
nunjucks.configure({ autoescape: true });
const renderedString = nunjucks.renderString('Hello {{ name }}!', { name: 'World' });
console.log(renderedString);
Template Inheritance
Nunjucks supports template inheritance, allowing you to create a base template and extend it in other templates. This example shows a base template 'base.html' and an extending template 'index.html'. The rendered output will have the title 'Home' and the content 'Welcome to the homepage!'.
const nunjucks = require('nunjucks');
nunjucks.configure('views');
// base.html
// <html>
// <head><title>{% block title %}Default Title{% endblock %}</title></head>
// <body>{% block content %}{% endblock %}</body>
// </html>
// index.html
// {% extends 'base.html' %}
// {% block title %}Home{% endblock %}
// {% block content %}Welcome to the homepage!{% endblock %}
const renderedString = nunjucks.render('index.html');
console.log(renderedString);
Custom Filters
Nunjucks allows you to create custom filters to manipulate data within templates. In this example, a custom filter 'shorten' is created to truncate a string to a specified length. The template 'Result: {{ message | shorten(7) }}' will render as 'Result: Hello, ' when provided with the context { message: 'Hello, World!' }.
const nunjucks = require('nunjucks');
nunjucks.configure();
nunjucks.addFilter('shorten', function(str, count) {
return str.slice(0, count || 5);
});
const renderedString = nunjucks.renderString('Result: {{ message | shorten(7) }}', { message: 'Hello, World!' });
console.log(renderedString);
Handlebars is another popular templating engine for JavaScript. It uses a similar syntax to Nunjucks but is more focused on simplicity and logic-less templates. Handlebars is known for its ease of use and integration with various frameworks.
EJS (Embedded JavaScript) is a templating engine that allows you to generate HTML with plain JavaScript. It is less feature-rich compared to Nunjucks but is very straightforward and easy to use, making it a good choice for simple templating needs.
Pug, formerly known as Jade, is a high-performance template engine heavily influenced by Haml. It uses indentation-based syntax, which can be more concise but also has a steeper learning curve compared to Nunjucks. Pug is known for its clean and readable templates.
Nunjucks is a full featured templating engine for javascript. It is a direct port of the Python-powered jinja2 templating engine and aims to be feature-complete with jinja2.
It was born out of frustration with other javascript templating engines. The most popular ones either are ugly and don't abstract enough (EJS) or have too different of a syntax (Jade).
The only other project like this is jinjs, which seems to have been abandoned. The code is also not Javascript, but Coco, is difficult to work on, has bugs, and is missing features. Nunjucks hopes to be a robust, pure javascript, and easily extended port of jinja2.
npm install nunjucks
The current version is v0.1.6 (changelog).
See http://nunjucks.jlongster.com/ for complete documentation.
FAQs
A powerful templating engine with inheritance, asynchronous control, and more (jinja2 inspired)
The npm package nunjucks receives a total of 859,533 weekly downloads. As such, nunjucks popularity was classified as popular.
We found that nunjucks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.