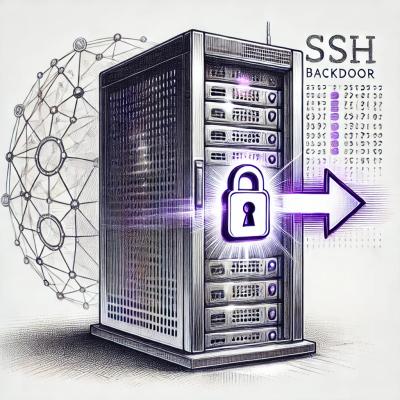
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
An auth library for react to login with google and github. It works with redirect url to get the code and then exchange it for the access token with your server side api to get the user details.
The OAuthify library provides a seamless integration for adding OAuth-based login functionality into your React application. This package comes with pre-built headless components for Google and GitHub login buttons, making the OAuth flow implementation straightforward and efficient.
To install OAuthify, run:
npm install oauthify
Implementing OAuthify in your React application involves three simple steps:
<OAuthifyProvider />
.<OAuthifyRedirect />
component to handle the OAuth callback.<GoogleLoginButton />
or <GithubLoginButton />
components as needed.To use the OAuthify Provider, wrap your entire application with <OAuthifyProvider>
:
import React from 'react';
import {
OAuthifyProvider,
GoogleLoginButton,
GitHubLoginButton,
GoogleIcon,
GithubIcon,
} from 'oauthify';
import { useAuth } from './contexts/Auth.context';
const googleClientId = 'xxxxxxxxx';
const githubClientId = 'XXXXXXXX';
const App = () => {
return (
<OAuthifyProvider>
<div>
<h1>My App</h1>
<LoginComponent />
</div>
</OAuthifyProvider>
);
};
const LoginComponent = () => {
const { onSuccess, onFailure, setOnSuccess } = useOAuthify();
const handleSuccess = () => {
// Handle the success state of LoginWithGoogle or LoginWithGithub
};
const handleFailure = () => {
// Handle the success state of LoginWithGoogle or LoginWithGithub
};
React.useEffect(() => {
handleSuccess();
}, [onSuccess]);
React.useEffect(() => {
handleFailure();
}, [onFailure]);
return (
<>
<div className="flex flex-row justify-center items-center my-6 space-x-2">
<GoogleLoginButton clientId={googleClientId} redirectUri={redirectUri}>
<div
style={{
display: 'flex',
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: '4px',
border: '1px solid #e1e4e8',
padding: '6px 12px',
fontSize: '14px',
}}
>
<div className="mr-2">
{' '}
<GoogleIcon size={16} />{' '}
</div>{' '}
{formType === 'signin' ? 'Sign in' : 'Sign up'} with Google
</div>{' '}
</GoogleLoginButton>
<GitHubLoginButton clientId={githubClientId} redirectUri={redirectUri}>
>
<div
style={{
display: 'flex',
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: '4px',
border: '1px solid #e1e4e8',
padding: '6px 12px',
fontSize: '14px',
}}
>
<div className="mr-2">
{' '}
<GithubIcon size={16} />
</div>{' '}
{formType === 'signin' ? 'Sign in' : 'Sign up'} with GitHub
</div>
</GitHubLoginButton>
</div>
</>
);
};
export default App;
To use the Google login button:
GoogleLoginButton
component:import React from 'react';
import { GoogleLoginButton } from 'oauthify';
const App = () => {
const handleSuccess = (response) => {
console.log('Google login success:', response);
};
const handleFailure = (error) => {
console.error('Google login failure:', error);
};
return (
<div>
<GoogleLoginButton
clientId="YOUR_GOOGLE_CLIENT_ID"
redirectUri={`${window.location.origin}/oauthify-redirect`}
onSuccess={handleSuccess}
onFailure={handleFailure}
>
Login with Google
</GoogleLoginButton>
</div>
);
};
export default App;
In your router configuration file:
import { OAuthifyRedirect } from 'oauthify';
{
path: '/oauthify-redirect',
component: OAuthifyRedirect
}
To use the GitHub login button:
GitHubLoginButton
component:import React from 'react';
import { GitHubLoginButton } from 'oauthify';
const App = () => {
const handleSuccess = (response) => {
console.log('GitHub login success:', response);
};
const handleFailure = (error) => {
console.error('GitHub login failure:', error);
};
return (
<div>
<GitHubLoginButton
clientId="YOUR_GITHUB_CLIENT_ID"
redirectUri={`${window.location.origin}/oauthify-redirect`}
onSuccess={handleSuccess}
onFailure={handleFailure}
>
Login with GitHub
</GitHubLoginButton>
</div>
);
};
export default App;
We welcome and appreciate contributions! If you want to contribute, please follow these steps:
git checkout -b feature/YourFeature
).git commit -am 'Add some feature'
).git push origin feature/YourFeature
).We encourage contributions for adding support for other providers, improving documentation, and fixing bugs. If you find this project helpful, please give it a star on GitHub to help others discover it!
This project is licensed under the MIT License - see the LICENSE.md file for details.
FAQs
An auth library for react to login with google and github. It works with redirect url to get the code and then exchange it for the access token with your server side api to get the user details.
The npm package oauthify receives a total of 5 weekly downloads. As such, oauthify popularity was classified as not popular.
We found that oauthify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.