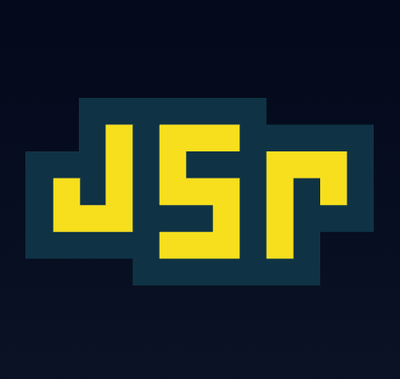
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The obj-case npm package provides utilities for manipulating and accessing properties within JavaScript objects using dot notation. It simplifies the process of getting, setting, and deleting nested properties.
Get Property
This feature allows you to retrieve the value of a nested property within an object using a dot-notated string.
const objCase = require('obj-case');
const obj = { a: { b: { c: 42 } } };
const value = objCase.get(obj, 'a.b.c');
console.log(value); // 42
Set Property
This feature allows you to set the value of a nested property within an object using a dot-notated string.
const objCase = require('obj-case');
const obj = { a: { b: { } } };
objCase.set(obj, 'a.b.c', 42);
console.log(obj); // { a: { b: { c: 42 } } }
Delete Property
This feature allows you to delete a nested property within an object using a dot-notated string.
const objCase = require('obj-case');
const obj = { a: { b: { c: 42 } } };
objCase.del(obj, 'a.b.c');
console.log(obj); // { a: { b: { } } }
Lodash is a popular utility library that provides a wide range of functions for manipulating arrays, objects, and other data types. It includes methods like _.get, _.set, and _.unset for working with nested properties, similar to obj-case.
Dot-prop is a lightweight package specifically designed for getting, setting, and deleting nested properties in objects using dot notation. It offers similar functionality to obj-case but is more focused and minimalistic.
Object-path is another utility library for accessing and manipulating deep properties in objects using dot notation. It provides a comprehensive set of methods for working with nested properties, similar to obj-case.
Work with objects of different cased keys. Anything supported by nbubna/Case. Makes finding and editing objects between languages a little more forgiving while still not modifying the original JSON.
Install with component(1):
$ component install segmentio/obj-case
Returns the value for the object with the given key
var obj = { my : { super_cool : { climbingShoes : 'x' }}};
objCase.find(obj, 'my.superCool.CLIMBING SHOES'); // 'x'
Deletes a nested key
var obj = { 'a wild' : { mouse : { APPEARED : true }}};
objCase.del(obj, 'aWild.mouse.appeared');
console.log(obj); // { 'a wild' : { mouse : {} }}
Replaces a nested key's value
var obj = { replacing : { keys : 'is the best' }};
objCase.replace(obj, 'replacing.keys', 'is just okay');
console.log(obj) // { replacing : { keys : 'is just okay' }}
MIT
FAQs
Work with objects of different cased keys
The npm package obj-case receives a total of 626,928 weekly downloads. As such, obj-case popularity was classified as popular.
We found that obj-case demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.