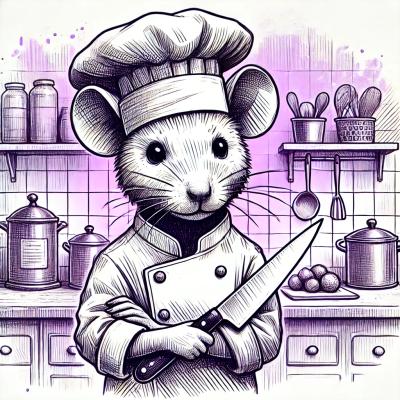
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
observable-fns
Advanced tools
Light-weight observable implementation and utils written in TypeScript. Based on zen-observable.
The observable-fns package provides a set of utilities for working with observables in JavaScript. It is designed to be lightweight and easy to use, offering a range of functionalities for creating, transforming, and managing observables.
Creating Observables
This feature allows you to create new observables. The example demonstrates creating an observable that emits 'Hello' and 'World' before completing.
const { Observable } = require('observable-fns');
const observable = new Observable(observer => {
observer.next('Hello');
observer.next('World');
observer.complete();
});
observable.subscribe({
next: value => console.log(value),
complete: () => console.log('Done')
});
Transforming Observables
This feature allows you to transform the values emitted by an observable. The example shows how to use the `map` operator to double the values emitted by the original observable.
const { Observable, map } = require('observable-fns');
const observable = new Observable(observer => {
observer.next(1);
observer.next(2);
observer.next(3);
observer.complete();
});
const transformed = observable.pipe(map(x => x * 2));
transformed.subscribe({
next: value => console.log(value),
complete: () => console.log('Done')
});
Combining Observables
This feature allows you to combine multiple observables into one. The example demonstrates merging two observables that emit 'A' and 'B' respectively.
const { Observable, merge } = require('observable-fns');
const observable1 = new Observable(observer => {
observer.next('A');
observer.complete();
});
const observable2 = new Observable(observer => {
observer.next('B');
observer.complete();
});
const combined = merge(observable1, observable2);
combined.subscribe({
next: value => console.log(value),
complete: () => console.log('Done')
});
RxJS is a library for reactive programming using observables that makes it easier to compose asynchronous or callback-based code. It is more feature-rich and widely used compared to observable-fns, offering a comprehensive set of operators and utilities.
Most.js is a reactive programming library that focuses on high performance by using a minimal API. It is similar to observable-fns in its lightweight approach but is optimized for speed and efficiency.
Bacon.js is a functional reactive programming library for JavaScript. It provides a wide range of operators and is designed to work well with event streams and property values. It offers more features compared to observable-fns but may have a steeper learning curve.
Light-weight Observable implementation and common toolbelt functions. Based on zen-observable
, re-implemented in TypeScript. Zero dependencies, tree-shakeable.
The aim is to provide a lean Observable implementation with a small footprint that's fit to be used in libraries as an alternative to the huge RxJS.
Find all the provided functions and constructors in the 👉 API Documentation
🧩 Composable functional streams
🚀 map(), filter() & friends support async handlers
🔩 Based on popular zen-observable
, re-implemented in TypeScript
🌳 Zero dependencies, tree-shakeable
npm install observable-fns
An observable is basically a stream of asynchronously emitted values that you can subscribe to. In a sense it is to the event emitter what the promise is to the callback.
The main difference to a promise is that a promise only resolves once, whereas observables can yield values repeatedly. They can also fail with an error, like a promise, and they come with a completion event to indicate that no more values will be send.
For a quick introduction on how to use observables, check out the zen-observable readme.
import { Observable, multicast } from "observable-fns"
function subscribeToServerSentEvents(url) {
// multicast() will make the observable "hot", so multiple
// subscribers will share the same event source
return multicast(new Observable(observer => {
const eventStream = new EventSource(url)
eventStream.addEventListener("message", message => observer.next(message))
eventStream.addEventListener("error", error => observer.error(error))
return () => eventStream.close()
}))
}
subscribeToServerSentEvents("http://localhost:3000/events")
.filter(event => !event.isStale)
.subscribe(event => console.log("Server sent event:", event))
You can import everything you need directly from the package:
import { Observable, flatMap } from "observable-fns"
If you write front-end code and care about bundle size, you can either depend on tree-shaking or explicitly import just the parts that you need:
import Observable from "observable-fns/observable"
import flatMap from "observable-fns/flatMap"
Functions like filter()
, flatMap()
, map()
accept asynchronous handlers – this can be a big win compared to the usual methods on Observable.prototype
that only work with synchronous handlers.
Those functions will also make sure that the values are consistently emitted in the same order as the input observable emitted them.
import { Observable, filter } from "observable-fns"
const existingGitHubUsersObservable = Observable.from(["andywer", "bcdef", "charlie"])
.pipe(
filter(async name => {
const response = await fetch(`https://github.com/${name}`)
return response.status === 200
})
)
See docs/API.md for an overview of the full API.
MIT
FAQs
Light-weight observable implementation and utils written in TypeScript. Based on zen-observable.
The npm package observable-fns receives a total of 143,026 weekly downloads. As such, observable-fns popularity was classified as popular.
We found that observable-fns demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.