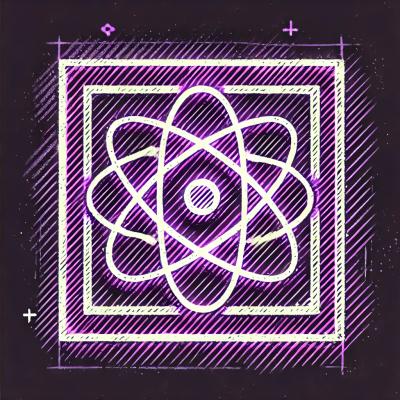
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
OctaviaDB - A lightweight, AES-encrypted NoSQL database for fast and secure local storage in Node.js.
npm install octavia-db
const OctaviaDB = require('octavia-db');
// Initialize the OctaviaDB instance
const db = new OctaviaDB({
database: './my-database', // Path to your database directory
password: 'my-secret-password', // Password for encryption
autoCommitInterval: 5000 // Auto commit changes every 5 seconds
});
// Get information about the database
console.log(db.info());
const OctaviaDB = require('octavia-db');
const db = new OctaviaDB({
database: './my-database',
password: 'my-secret-password',
autoCommitInterval: 5000
});
// Create a new collection
const collection = db.collection('users');
// Insert a single document
collection.insert({ id: 1, name: 'John Doe', age: 30 });
collection.insert({ id: 2, name: 'Jane Smith', age: 25 });
// Insert multiple documents
collection.insertMany([
{ id: 3, name: 'Michael Johnson', age: 35 },
{ id: 4, name: 'Sarah Lee', age: 40 }
]);
// Find a document by a query
const user = collection.find({ id: 1 });
console.log(user); // Output: { id: 1, name: 'John Doe', age: 30 }
// Update a document
collection.update({ id: 1 }, { age: 31 });
// Remove a document
collection.remove({ id: 2 });
// Find multiple documents
const usersAbove30 = collection.findMany({ age: 30 });
console.log(usersAbove30); // Output: [ { id: 1, name: 'John Doe', age: 31 }, { id: 3, name: 'Michael Johnson', age: 35 } ]
// Info about the collection
console.log(collection.info());
const OctaviaDB = require('octavia-db');
const db = new OctaviaDB({
database: './my-database',
password: 'my-secret-password',
autoCommitInterval: 5000
});
// Create a new data object
const dataObject = db.dataObject('settings');
// Set key-value pairs in the data object
dataObject.set('theme', 'dark');
dataObject.set('notifications', true);
dataObject.set('language', 'en');
// Get values from the data object
console.log(dataObject.get('theme')); // Output: 'dark'
console.log(dataObject.get('notifications')); // Output: true
console.log(dataObject.get('language')); // Output: 'en'
// Update a value in the data object
dataObject.set('theme', 'light');
// Remove value
dataObject.remove('language');
// Info about the data object
console.log(dataObject.info()); // Outputs stats on the data object (size, creation time, etc.)
const OctaviaDB = require('octavia-db');
const db = new OctaviaDB({
database: './my-database',
password: 'my-secret-password',
autoCommitInterval: 5000
});
// Create a collection and insert data
const collection = db.collection('users');
collection.insert({ id: 1, name: 'John Doe', age: 30 });
collection.insert({ id: 2, name: 'Jane Smith', age: 25 });
// Delete a collection
collection.delete();
// Create a new data object
const dataObject = db.dataObject('settings');
dataObject.set('theme', 'dark');
dataObject.set('notifications', true);
// Delete a data object
dataObject.delete();
// Delete the entire database (be cautious with this!)
db.delete();
const OctaviaDB = require('octavia-db');
const db = new OctaviaDB({
database: './my-database',
password: 'my-secret-password',
autoCommitInterval: 5000
});
// Check if a collection exists
const collectionExists = db.collectionExists('users');
console.log(`Collection 'users' exists: ${collectionExists}`); // Output: true/false
// Check if a data object exists
const dataObjectExists = db.documentExists('settings');
console.log(`Data Object 'settings' exists: ${dataObjectExists}`); // Output: true/false
// database methods
db.info() // Returns information about the database
db.delete() // Deletes the entire database
db.collection(collectionName, encrypt) // Creates a new collection within the database.
db.dataObject(dataObjectName, encrypt) // Creates a new data object within the database.
db.collectionExists(collectionName) // Checks if a collection exists.
db.documentExists(documentName) // Checks if a document exists.
// collection methods.
collection.info() // information about collection
collection.delete() // delete collection
collection.insert(data, immediateCommit) // Inserts a new item into the collection.
collection.insertMany(dataArray, immediateCommit) // Inserts multiple items into the collection.
collection.find(query) // Finds a single item that matches the query.
collection.findMany(query) // Finds multiple items that match the query.
collection.update(query, newData, immediateCommit) // Updates an item in the collection.
collection.updateMany(query, newData, immediateCommit) // Updates multiple items in the collection.
collection.remove(query, immediateCommit) // Removes an item from the collection.
collection.removeMany(query, immediateCommit) // Removes multiple items from the collection.
// DataObject Methods
dataObj.set(key, value, immediateCommit) // Sets a key-value pair in the data object.
dataObj.get(key) // Retrieves a value from the data object.
dataObj.remove(key) // remove a value from the data object.
dataObj.info() // Returns information about the data object.
FAQs
OctaviaDB - A lightweight, AES-encrypted NoSQL database for fast and secure local storage in Node.js.
The npm package octavia-db receives a total of 19 weekly downloads. As such, octavia-db popularity was classified as not popular.
We found that octavia-db demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.