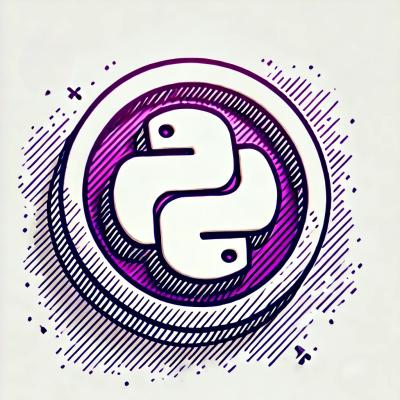
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Javascript color object with implicit color space conversions. Supports RGB, HSV, HSL and CMYK with alpha channel.
The onecolor npm package is a versatile color manipulation library that allows you to work with colors in various formats, convert between them, and perform operations like blending and adjusting color properties.
Color Creation
You can create a color object from a string representing a color in various formats (hex, RGB, HSL, etc.). The created color object can then be used for further manipulations.
const onecolor = require('onecolor');
const color = onecolor('#3498db');
console.log(color.css()); // Outputs: 'rgb(52, 152, 219)'
Color Conversion
The package allows you to convert colors between different formats such as HEX, RGB, and HSL.
const onecolor = require('onecolor');
const color = onecolor('#3498db');
console.log(color.hex()); // Outputs: '#3498db'
console.log(color.rgb()); // Outputs: 'rgb(52, 152, 219)'
console.log(color.hsl()); // Outputs: 'hsl(204, 0.68, 0.53)'
Color Manipulation
You can manipulate color properties like lightness, saturation, and hue. This example demonstrates adjusting the lightness of a color.
const onecolor = require('onecolor');
const color = onecolor('#3498db').lightness(0.5);
console.log(color.css()); // Outputs: 'rgb(26, 76, 109)'
Color Blending
The package supports blending two colors together. This example shows how to blend two colors with equal weight.
const onecolor = require('onecolor');
const color1 = onecolor('#3498db');
const color2 = onecolor('#e74c3c');
const blendedColor = color1.mix(color2, 0.5);
console.log(blendedColor.css()); // Outputs: 'rgb(153, 101, 123)'
Chroma.js is a powerful and flexible color manipulation library that supports a wide range of color spaces and provides advanced color manipulation features. Compared to onecolor, chroma-js offers more extensive functionality and better performance for complex color operations.
The color package is another popular library for color manipulation and conversion. It provides a simple API for working with colors and supports various color models. While it offers similar functionalities to onecolor, it is known for its ease of use and comprehensive documentation.
TinyColor is a small, fast library for color manipulation and conversion. It is designed to be lightweight and easy to use, making it a good choice for projects where performance and simplicity are important. Compared to onecolor, TinyColor is more focused on providing a minimalistic API with essential color manipulation features.
JavaScript color calculation toolkit for node.js and the browser.
Features:
rgb(...)
, rgba(...)
or hsv(...)
Module support:
Package managers:
npm install onecolor
bower install color
WARNING IE USERS:
This library uses some modern ecmascript methods that aren't available in IE versions below IE9.
To keep the core library small, these methods aren't polyfilled in the library itself.
If you want IE support for older IE versions, please include one-color-ieshim.js before the color library. This is only needed if you don't already have a library installed that polyfills Array.prototype.map
and Array.prototype.forEach
.
In the browser (change one-color.js to one-color-all.js to gain named color support):
<script src='one-color.js'></script>
<script>
alert('Hello, ' + one.color('#650042').lightness(.3).green(.4).hex() + ' world!');
</script>
In node.js (after npm install onecolor
):
var color = require('onecolor');
console.warn(color('rgba(100%, 0%, 0%, .5)').alpha(.4).cssa()); // 'rgba(255,0,0,0.4)'
one.color
is the parser. All of the above return color instances in
the relevant color space with the channel values (0..1) as instance
variables:
var myColor = one.color('#a9d91d');
myColor instanceof one.color.RGB; // true
myColor.red() // 0.6627450980392157
You can also parse named CSS colors (works out of the box in node.js, but the requires the slightly bigger one-color-all.js build in the browser):
one.color('maroon').lightness(.3).hex() // '#990000'
To turn a color instance back into a string, use the hex()
, css()
,
and cssa()
methods:
one.color('rgb(124, 96, 200)').hex() // '#7c60c8'
one.color('#bb7b81').cssa() // 'rgba(187,123,129,1)'
Color instances have getters/setters for all channels in all supported
colorspaces (red()
, green()
, blue()
, hue()
, saturation()
, lightness()
,
value()
, alpha()
, etc.). Thus you don't need to think about which colorspace
you're in. All the necessary conversions happen automatically:
one.color('#ff0000') // Red in RGB
.green(1) // Set green to the max value, producing yellow (still RGB)
.hue(.5, true) // Add 180 degrees to the hue, implicitly converting to HSV
.hex() // Dump as RGB hex syntax: '#2222ff'
When called without any arguments, they return the current value of the channel (0..1):
one.color('#09ffdd').green() // 1
one.color('#09ffdd').saturation() // 0.9647058823529412
When called with a single numerical argument (0..1), a new color object is returned with that channel replaced:
var myColor = one.color('#00ddff');
myColor.red(.5).red() // .5
// ... but as the objects are immutable, the original object retains its value:
myColor.red() // 0
When called with a single numerical argument (0..1) and true
as
the second argument, a new value is returned with that channel
adjusted:
one.color('#ff0000') // Red
.red(-.1, true) // Adjust red channel by -0.1
.hex() // '#e60000'
All color instances have an alpha channel (0..1), defaulting to 1 (opaque). You can simply ignore it if you don't need it.
It's preserved when converting between colorspaces:
one.color('rgba(10, 20, 30, .8)')
.green(.4)
.saturation(.2)
.alpha() // 0.8
If you need to know whether two colors represent the same 8 bit color, regardless
of colorspace, compare their hex()
values:
one.color('#f00').hex() === one.color('#e00').red(1).hex() // true
Use the equals
method to compare two color instances within a certain
epsilon (defaults to 1e-9
).
one.color('#e00').lightness(.00001, true).equals(one.color('#e00'), 1e-5) // false
one.color('#e00').lightness(.000001, true).equals(one.color('#e00'), 1e-5) // true
Before comparing the equals
method converts the other color to the right colorspace,
so you don't need to convert explicitly in this case either:
one.color('#e00').hsv().equals(one.color('#e00')) // true
Color parser function, the recommended way to create a color instance:
one.color('#a9d91d') // Regular hex syntax
one.color('a9d91d') // hex syntax, # is optional
one.color('#eee') // Short hex syntax
one.color('rgb(124, 96, 200)') // CSS rgb syntax
one.color('rgb(99%, 40%, 0%)') // CSS rgb syntax with percentages
one.color('rgba(124, 96, 200, .4)') // CSS rgba syntax
one.color('hsl(120, 75%, 75%)') // CSS hsl syntax
one.color('hsla(120, 75%, 75%, .1)') // CSS hsla syntax
one.color('hsv(220, 47%, 12%)') // CSS hsv syntax (non-standard)
one.color('hsva(120, 75%, 75%, 0)') // CSS hsva syntax (non-standard)
one.color([0, 4, 255, 120]) // CanvasPixelArray entry, RGBA
one.color(["RGB", .5, .1, .6, .9]) // The output format of color.toJSON()
The slightly bigger one-color-all.js build adds support for the standard suite of named CSS colors:
one.color('maroon')
one.color('darkolivegreen')
Existing one.color instances pass through unchanged, which is useful in APIs where you want to accept either a string or a color instance:
one.color(one.color('#fff')) // Same as one.color('#fff')
Serialization methods:
color.hex() // 6-digit hex string: '#bda65b'
color.css() // CSS rgb syntax: 'rgb(10,128,220)'
color.cssa() // CSS rgba syntax: 'rgba(10,128,220,0.8)'
color.toString() // For debugging: '[one.color.RGB: Red=0.3 Green=0.8 Blue=0 Alpha=1]'
color.toJSON() // ["RGB"|"HSV"|"HSL", <number>, <number>, <number>, <number>]
Getters -- return the value of the channel (converts to other colorspaces as needed):
color.red()
color.green()
color.blue()
color.hue()
color.saturation()
color.value()
color.lightness()
color.alpha()
color.cyan() // one-color-all.js and node.js only
color.magenta() // one-color-all.js and node.js only
color.yellow() // one-color-all.js and node.js only
color.black() // one-color-all.js and node.js only
Setters -- return new color instances with one channel changed:
color.red(<number>)
color.green(<number>)
color.blue(<number>)
color.hue(<number>)
color.saturation(<number>)
color.value(<number>)
color.lightness(<number>)
color.alpha(<number>)
color.cyan(<number>) // one-color-all.js and node.js only
color.magenta(<number>) // one-color-all.js and node.js only
color.yellow(<number>) // one-color-all.js and node.js only
color.black(<number>) // one-color-all.js and node.js only
Adjusters -- return new color instances with the channel adjusted by the specified delta (0..1):
color.red(<number>, true)
color.green(<number>, true)
color.blue(<number>, true)
color.hue(<number>, true)
color.saturation(<number>, true)
color.value(<number>, true)
color.lightness(<number>, true)
color.alpha(<number>, true)
color.cyan(<number>, true) // one-color-all.js and node.js only
color.magenta(<number>, true) // one-color-all.js and node.js only
color.yellow(<number>, true) // one-color-all.js and node.js only
color.black(<number>, true) // one-color-all.js and node.js only
Comparison with other color objects, returns true
or false
(epsilon defaults to 1e-9
):
color.equals(otherColor[, <epsilon>])
"Low level" constructors, accept 3 or 4 numerical arguments (0..1):
new one.color.RGB(<red>, <green>, <blue>[, <alpha>])
new one.color.HSL(<hue>, <saturation>, <lightness>[, <alpha>])
new one.color.HSV(<hue>, <saturation>, <value>[, <alpha>])
The one-color-all.js build includes CMYK support:
new one.color.CMYK(<cyan>, <magenta>, <yellow>, <black>[, <alpha>])
All color instances have rgb()
, hsv()
, and hsl()
methods for
explicitly converting to another color space. Like the setter and
adjuster methods they return a new color object representing the same
color in another color space.
If for some reason you need to get all the channel values in a specific colorspace, do an explicit conversion first to cut down on the number of implicit conversions:
var myColor = one.color('#0620ff').lightness(+.3).rgb();
// Alerts '0 0.06265060240963878 0.5999999999999999':
alert(myColor.red() + ' ' + myColor.green() + ' ' + myColor.blue());
git clone https://github.com/One-com/one-color.git
cd one-color
npm install
make
If you aren't up for a complete installation, there are pre-built packages in the repository as well as the npm package:
one.color is licensed under a standard 2-clause BSD license -- see the LICENSE-file for details.
FAQs
Javascript color object with implicit color space conversions. Supports RGB, HSV, HSL and CMYK with alpha channel.
The npm package onecolor receives a total of 163,131 weekly downloads. As such, onecolor popularity was classified as popular.
We found that onecolor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.