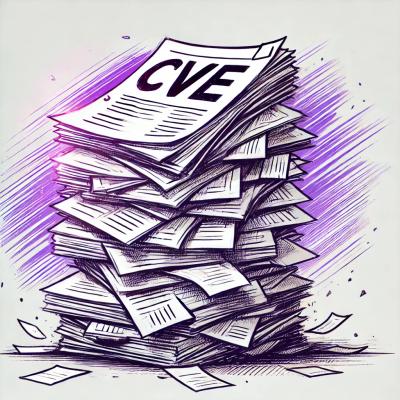
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
openapi-typescript
Advanced tools
The openapi-typescript npm package is a tool that generates TypeScript types from OpenAPI specifications. This helps developers ensure type safety and better integration between their API definitions and TypeScript code.
Generate TypeScript Types
This feature allows you to generate TypeScript types from an OpenAPI specification URL. The generated types are then saved to a file named 'types.ts'.
const openapiTS = require('openapi-typescript');
const fs = require('fs');
async function generateTypes() {
const types = await openapiTS('https://api.example.com/openapi.json');
fs.writeFileSync('types.ts', types);
}
generateTypes();
Generate Types from Local File
This feature allows you to generate TypeScript types from a local OpenAPI specification file. The generated types are saved to a file named 'types.ts'.
const openapiTS = require('openapi-typescript');
const fs = require('fs');
const path = require('path');
async function generateTypes() {
const types = await openapiTS(path.join(__dirname, 'openapi.json'));
fs.writeFileSync('types.ts', types);
}
generateTypes();
Custom Type Generation Options
This feature allows you to customize the type generation process by providing options such as a transform function. The transform function can be used to modify the schema before generating the types.
const openapiTS = require('openapi-typescript');
const fs = require('fs');
async function generateTypes() {
const types = await openapiTS('https://api.example.com/openapi.json', {
transform: (schema) => {
// Custom transformation logic
return schema;
}
});
fs.writeFileSync('types.ts', types);
}
generateTypes();
The swagger-typescript-api package generates TypeScript API client code from Swagger/OpenAPI definitions. It provides more advanced features like generating API client methods and supports both Swagger 2.0 and OpenAPI 3.0 specifications.
The typescript-fetch package is a generator for TypeScript clients using the Fetch API. It generates TypeScript code from OpenAPI specifications and is particularly useful for creating client-side code that interacts with RESTful APIs.
🚀 Convert OpenAPI 3.0 and 2.0 (Swagger) schemas to TypeScript interfaces using Node.js.
💅 The output is prettified with Prettier (and can be customized!).
👉 Works for both local and remote resources (filesystem and HTTP).
View examples:
npx openapi-typescript schema.yaml --output schema.ts
# 🤞 Loading spec from tests/v2/specs/stripe.yaml…
# 🚀 schema.yaml -> schema.ts [250ms]
npx openapi-typescript https://petstore.swagger.io/v2/swagger.json --output petstore.ts
# 🤞 Loading spec from https://petstore.swagger.io/v2/swagger.json…
# 🚀 https://petstore.swagger.io/v2/swagger.json -> petstore.ts [650ms]
Thanks to @psmyrdek for the remote spec feature!
Import any top-level item from the generated spec to use it. It works best if you also alias types to save on typing:
import { components } from './generated-schema.ts';
type APIResponse = components["schemas"]["APIResponse"];
The reason for all the ["…"]
everywhere is because OpenAPI lets you use more characters than are valid TypeScript identifiers. The goal of this project is to generate all of your schema, not merely the parts that are “TypeScript-safe.”
Also note that there’s a special operations
interface that you can import OperationObjects
by their operationId:
import { operations } from './generated-schema.ts';
type getUsersById = operations["getUsersById"];
This is the only place where our generation differs from your schema as-written, but it’s done so as a convenience and shouldn’t cause any issues (you can still use deep references as-needed).
Thanks to @gr2m for the operations feature!
stdout
npx openapi-typescript schema.yaml
In your package.json
, for each schema you’d like to transform add one generate:specs:[name]
npm-script. Then combine
them all into one generate:specs
script, like so:
"scripts": {
"generate:specs": "npm run generate:specs:one && npm run generate:specs:two && npm run generate:specs:three",
"generate:specs:one": "npx openapi-typescript one.yaml -o one.ts",
"generate:specs:two": "npx openapi-typescript two.yaml -o two.ts",
"generate:specs:three": "npx openapi-typescript three.yaml -o three.ts"
}
If you use npm-run-all, you can shorten this:
"scripts": {
"generate:specs": "run-p generate:specs:*",
You can even specify unique options per-spec, if needed. To generate them all together, run:
npm run generate:specs
Rinse and repeat for more specs.
For anything more complicated, or for generating specs dynamically, you can also use the Node API.
Option | Alias | Default | Description |
---|---|---|---|
--output [location] | -o | (stdout) | Where should the output file be saved? |
--prettier-config [location] | (optional) Path to your custom Prettier configuration for output | ||
--raw-schema | false | Generate TS types from partial schema (e.g. having components.schema at the top level) |
npm i --save-dev openapi-typescript
const { readFileSync } = require("fs");
const swaggerToTS = require("openapi-typescript").default;
const input = JSON.parse(readFileSync("spec.json", "utf8")); // Input can be any JS object (OpenAPI format)
const output = swaggerToTS(input); // Outputs TypeScript defs as a string (to be parsed, or written to a file)
The Node API is a bit more flexible: it will only take a JS object as input (OpenAPI format), and return a string of TS definitions. This lets you pull from any source (a Swagger server, local files, etc.), and similarly lets you parse, post-process, and save the output anywhere.
If your specs are in YAML, you’ll have to convert them to JS objects using a library such as js-yaml. If you’re batching large folders of specs, glob may also come in handy.
PascalCase
or follow any TypeScript-isms; faithfully reproduce your schema as closely as possible, capitalization
and all)PRs are welcome! Please see our CONTRIBUTING.md guide. Opening an issue beforehand to discuss is encouraged but not required.
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Convert OpenAPI 3.0 & 3.1 schemas to TypeScript
We found that openapi-typescript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.