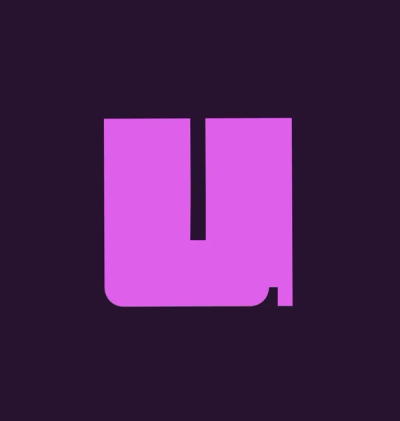
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
openbci-ganglion
Advanced tools
A Node.js module for OpenBCI ~ written with love by Push The World!
We are proud to support all functionality of the Ganglion (4 channel). Push The World is actively developing and maintaining this module.
The purpose of this module is to get connected and start streaming as fast as possible.
Get connected and start streaming right now
const Ganglion = require('openbci-ganglion');
const ganglion = new Ganglion();
ganglion.once('ganglionFound', (peripheral) => {
// Stop searching for BLE devices once a ganglion is found.
ganglion.searchStop();
ganglion.on('sample', (sample) => {
/** Work with sample */
console.log(sample.sampleNumber);
for (let i = 0; i < ganglion.numberOfChannels(); i++) {
console.log("Channel " + (i + 1) + ": " + sample.channelData[i].toFixed(8) + " Volts.");
}
});
ganglion.once('ready', () => {
ganglion.streamStart();
});
ganglion.connect(peripheral);
});
// Start scanning for BLE devices
ganglion.searchStart();
Please ensure Python 2.7 is installed for all OS.
libbluetooth-dev
libudev-dev
In order to stream data on Linux without root/sudo access, you may need to give the node
binary privileges to start and stop BLE advertising.
sudo setcap cap_net_raw+eip $(eval readlink -f `which node`)
Note: this command requires setcap
to be installed. Install it with the libcap2-bin
package.
sudo apt-get install libcap2-bin
See @don's set up guide on Bluetooth LE with Node.js and Noble on Windows.
Install from npm:
npm install openbci-ganglion
The Ganglion driver used by OpenBCI's Processing GUI and Electron Hub.
Check out the automatic tests written for it!
Initializing the board:
const Ganglion = require('openbci-ganglion');
const ganglion = new Ganglion();
For initializing with options, such as verbose print outs:
const Ganglion = require('openbci-ganglion');
const ourBoard = new Ganglion({
verbose: true
});
For initializing with callback, such as to catch errors on noble
startup:
const Ganglion = require('openbci-ganglion');
const ourBoard = new Ganglion((error) => {
if (error) {
console.log("error", error);
} else {
console.log("no error");
}
});
For initializing with options and callback, such as verbose and to catch errors on noble
startup:
const Ganglion = require('openbci-ganglion');
const ourBoard = new Ganglion({
verbose: true
},(error) => {
if (error) {
console.log("error", error);
} else {
console.log("no error");
}
});
You MUST wait for the 'ready' event to be emitted before streaming/talking with the board. The ready happens asynchronously so installing the 'sample' listener and writing before the ready event might result in... nothing at all.
const Ganglion = require('openbci-ganglion');
const ourBoard = new Ganglion();
ourBoard.connect(portName).then(function(boardSerial) {
ourBoard.on('ready',function() {
/** Start streaming, reading registers, what ever your heart desires */
});
}).catch(function(err) {
/** Handle connection errors */
});
sampleNumber
(a Number
between 0-255)channelData
(channel data indexed at 0 filled with floating point Numbers
in Volts)accelData
(Array
with X, Y, Z accelerometer values when new data available)timeStamp
(Number
the boardTime
plus the NTP calculated offset)The power of this module is in using the sample emitter, to be provided with samples to do with as you wish.
.connect(localName | peripheral)
streamStart()
const Ganglion = require('openbci-ganglion');
const ourBoard = new Ganglion();
ourBoard.connect(localName).then(function() {
ourBoard.on('ready',function() {
ourBoard.streamStart();
ourBoard.on('sample',function(sample) {
/** Work with sample */
});
});
}).catch(function(err) {
/** Handle connection errors */
});
Close the connection with .streamStop()
and disconnect with .disconnect()
const Ganglion = require('openbci-ganglion');
const ourBoard = new Ganglion();
ourBoard.streamStop().then(ourBoard.disconnect());
See Reference Guide for a complete list of impedance tests.
Create new instance of a Ganglion board.
options (optional)
Board optional configurations.
debug
{Boolean} - Print out a raw dump of bytes sent and received (Default false
)nobleAutoStart
{Boolean} - Automatically initialize noble
. Subscribes to blue tooth state changes and such. (Default true
)nobleScanOnPowerOn
{Boolean} - Start scanning for Ganglion BLE devices as soon as power turns on. (Default true
)sendCounts
{Boolean} - Send integer raw counts instead of scaled floats. (Default false
)simulate
{Boolean} - Full functionality, just mock data. Must attach Daisy module by setting simulatorDaisyModuleAttached
to true
in order to get 16 channels. (Default false
)simulatorBoardFailure
{Boolean} - Simulates board communications failure. This occurs when the RFduino on the board is not polling the RFduino on the dongle. (Default false
)simulatorHasAccelerometer
- {Boolean} - Sets simulator to send packets with accelerometer data. (Default true
)simulatorInjectAlpha
- {Boolean} - Inject a 10Hz alpha wave in Channels 1 and 2 (Default true
)simulatorInjectLineNoise
{String} - Injects line noise on channels. (3 Possible Options)
60Hz
- 60Hz line noise (Default) [America]50Hz
- 50Hz line noise [Europe]none
- Do not inject line noise.simulatorSampleRate
{Number} - The sample rate to use for the simulator. Simulator will set to 125 if simulatorDaisyModuleAttached
is set true
. However, setting this option overrides that setting and this sample rate will be used. (Default is 250
)verbose
{Boolean} - Print out useful debugging events (Default false
)Note, we have added support for either all lowercase OR camel case for the options, use whichever style you prefer.
callback (optional)
Callback function to catch errors. Returns only error if an error was encountered.
Used to enable the accelerometer. Will result in accelerometer packets arriving 10 times a second.
Note that the accelerometer is enabled by default.
Returns {Promise} - fulfilled once the command was sent to the board.
Used to disable the accelerometer. Prevents accelerometer data packets from arriving.
Note that the accelerometer is enabled by default.
Returns {Promise} - fulfilled once the command was sent to the board.
Used to start a scan if power is on. Useful if a connection is dropped.
Turn off a specified channel
channelNumber
A number (1-4) specifying which channel you want to turn off.
Returns {Promise} - fulfilled once the command was sent to the board.
Turn on a specified channel
channelNumber
A number (1-4) specifying which channel you want to turn on.
Returns {Promise} - fulfilled once the command was sent to the board.
The essential precursor method to be called initially to establish a ble connection to the OpenBCI ganglion board.
id {String | Object}
A string localName
or peripheral
(from noble
) object.
Returns {Promise} - fulfilled by a successful connection to the board.
Destroys the multi packet buffer. The mulit packet buffer holds data from the multi packet messages.
Closes the connection to the board. Waits for stop streaming command to be sent if currently streaming.
stopStreaming {Boolean} (optional)
true
if you want to stop streaming before disconnecting. (Default false
)
Returns {Promise} - fulfilled by a successful close, rejected otherwise.
Gets the local name of the attached Ganglion device. This is only valid after .connect()
Returns {null|String} - The local name.
Get's the multi packet buffer.
Returns {null|Buffer} - Can be null if no multi packets received.
Call to start testing impedance.
Returns {Promise} - that fulfills when all the commands are sent to the board.
Call to stop testing impedance.
Returns {Promise} - that fulfills when all the commands are sent to the board.
Checks if the driver is connected to a board.
Returns {Boolean} - true if connected
Checks if bluetooth is powered on. Cannot start scanning till this is true.
Returns {Boolean} - true if bluetooth is powered on.
Checks if noble is currently scanning. See .searchStart()
and [.searchStop
()](#method-search-stop
)
Returns {Boolean} - true if searching.
Checks if the board is currently sending samples.
Returns {Boolean} - true if streaming
Get the current number of channels available to use. (i.e. 4).
Returns {Number} - The total number of available channels.
Prints all register settings for the for board.
Returns {Promise} - Fulfilled if the command was sent to board.
Get the current sample rate.
Note: This is dependent on if you configured the board correctly on setup options. Specifically as a daisy.
Returns {Number} - The current sample rate.
Call to make noble
start scanning for Ganglions.
maxSearchTime {Number}
The amount of time to spend searching. (Default is 20 seconds)
Returns {Promise} - fulfilled if scan was started.
Call to make noble
stop scanning for Ganglions.
Returns {Promise} - fulfilled if scan was stopped.
Sends a soft reset command to the board.
Returns {Promise} - Fulfilled if the command was sent to board.
Sends a start streaming command to the board.
Note, You must have called and fulfilled .connect()
AND observed a 'ready'
emitter before calling this method.
Returns {Promise} - fulfilled if the command was sent.
Sends a stop streaming command to the board.
Note, You must have called and fulfilled .connect()
AND observed a 'ready'
emitter before calling this method.
Returns {Promise} - fulfilled if the command was sent.
Puts the board in synthetic data generation mode. Must call streamStart still.
Returns {Promise} - fulfilled if the command was sent.
Puts the board in synthetic data generation mode. Must call streamStart still.
Returns {Promise} - Indicating if the command was sent.
Used to send data to the board.
data {Array | Buffer | Number | String}
The data to write out.
Returns {Promise} - fulfilled if command was able to be sent.
Example
Sends a single character command to the board.
// ourBoard has fulfilled the promise on .connect() and 'ready' has been observed previously
ourBoard.write('a');
Sends an array of bytes
// ourBoard has fulfilled the promise on .connect() and 'ready' has been observed previously
ourBoard.write(['x','0','1','0','0','0','0','0','0','X']);
Call crazy? Go for it...
ourBoard.write('t');
ourBoard.write('a');
ourBoard.write('c');
ourBoard.write('o');
Emitted when the module receives accelerometer data.
Returns an object with properties:
accelData {Array}
Array of floats for each dimension in g's.
NOTE: Only present if sendCounts
is true
.
accelDataCounts {Array}
Array of integers for each dimension in counts.
NOTE: Only present if sendCounts
is false
.
Example (if sendCounts
is false
):
{
"accelData": [0.0, 0.0, 0.0, 0.0]
}
Example (if sendCounts
is true
):
{
"accelDataCounts": [0, 0, 0, 0]
}
Emitted when a packet (or packets) are dropped. Returns an array.
Emitted when there is an on the serial port.
Emitted when there is a new impedance available.
Returns an object with properties:
channelNumber {Number}
The channel number: 1, 2, 3, 4 respectively and 0 for reference.
impedanceValue {Number}
The impedance in ohms.
Example:
{
"channelNumber": 0,
"impedanceValue": 0
}
Emitted when there is a new raw data packet available.
Emitted when the board is in a ready to start streaming state.
Emitted when there is a new sample available.
Returns an object with properties:
channelData {Array}
Array of floats for each channel in volts..
NOTE: Only present if sendCounts
is true
.
channelDataCounts {Array}
Array of integers for each channel in counts.
NOTE: Only present if sendCounts
is false
.
sampleNumber {Number}
The sample number. Only goes up to 254.
timeStamp {Number}
The time the sample is packed up. Not accurate for ERP.
Example (if sendCounts
is false
):
{
"channelData": [0.0, 0.0, 0.0, 0.0],
"sampleNumber": 0,
"timeStamp": 0
}
Example (if sendCounts
is true
):
{
"channelDataCounts": [0, 0, 0, 0],
"sampleNumber": 0,
"timeStamp": 0
}
Emitted when a noble scan is started.
Emitted when a noble scan is stopped.
LabStreamingLayer is a tool for streaming or recording time-series data. It can be used to interface with Matlab, Python, Unity, and many other programs.
To use LSL with the NodeJS SDK, go to our labstreaminglayer example, which contains code that is ready to start an LSL stream of OpenBCI data.
Follow the directions in the readme to get started.
npm install
npm test
development
: git checkout development
git checkout -b my-new-feature
npm test
)git commit -m 'Add some feature'
git push origin my-new-feature
development
branch when submitting! :DMIT
v1.1.0
Add support for BLED112
FAQs
The official Node.js SDK for the OpenBCI Ganglion Biosensor Board.
The npm package openbci-ganglion receives a total of 35 weekly downloads. As such, openbci-ganglion popularity was classified as not popular.
We found that openbci-ganglion demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.