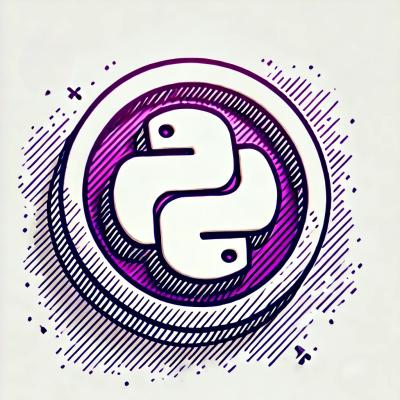
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
OpenPGP.js is a Javascript implementation of the OpenPGP protocol. This is defined in RFC 4880.
The openpgp npm package is a JavaScript implementation of the OpenPGP standard, which allows for encryption, decryption, signing, and verification of messages and files. It is widely used for secure communication and data protection.
Encrypting a message
This feature allows you to encrypt a message using a public key. The code sample demonstrates how to read a public key, create a message, and then encrypt it.
const openpgp = require('openpgp');
(async () => {
const publicKeyArmored = '-----BEGIN PGP PUBLIC KEY BLOCK ... END PGP PUBLIC KEY BLOCK-----';
const message = 'Hello, world!';
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const encrypted = await openpgp.encrypt({ message: await openpgp.createMessage({ text: message }), encryptionKeys: publicKey });
console.log(encrypted);
})();
Decrypting a message
This feature allows you to decrypt a message using a private key and passphrase. The code sample demonstrates how to read a private key, decrypt it with a passphrase, read an encrypted message, and then decrypt it.
const openpgp = require('openpgp');
(async () => {
const privateKeyArmored = '-----BEGIN PGP PRIVATE KEY BLOCK ... END PGP PRIVATE KEY BLOCK-----';
const passphrase = 'yourPassphrase';
const encryptedMessage = '-----BEGIN PGP MESSAGE ... END PGP MESSAGE-----';
const privateKey = await openpgp.decryptKey({ privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }), passphrase });
const message = await openpgp.readMessage({ armoredMessage: encryptedMessage });
const { data: decrypted } = await openpgp.decrypt({ message, decryptionKeys: privateKey });
console.log(decrypted);
})();
Signing a message
This feature allows you to sign a message using a private key and passphrase. The code sample demonstrates how to read a private key, decrypt it with a passphrase, create a message, and then sign it.
const openpgp = require('openpgp');
(async () => {
const privateKeyArmored = '-----BEGIN PGP PRIVATE KEY BLOCK ... END PGP PRIVATE KEY BLOCK-----';
const passphrase = 'yourPassphrase';
const message = 'Hello, world!';
const privateKey = await openpgp.decryptKey({ privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }), passphrase });
const signedMessage = await openpgp.sign({ message: await openpgp.createMessage({ text: message }), signingKeys: privateKey });
console.log(signedMessage);
})();
Verifying a signed message
This feature allows you to verify a signed message using a public key. The code sample demonstrates how to read a public key, read a signed message, and then verify the signature.
const openpgp = require('openpgp');
(async () => {
const publicKeyArmored = '-----BEGIN PGP PUBLIC KEY BLOCK ... END PGP PUBLIC KEY BLOCK-----';
const signedMessage = '-----BEGIN PGP SIGNED MESSAGE ... END PGP SIGNED MESSAGE-----';
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const message = await openpgp.readMessage({ armoredMessage: signedMessage });
const verificationResult = await openpgp.verify({ message, verificationKeys: publicKey });
const { verified } = verificationResult.signatures[0];
try {
await verified;
console.log('Signature is valid');
} catch (e) {
console.log('Signature is invalid');
}
})();
node-forge is a JavaScript library that provides a set of cryptographic utilities, including support for RSA, AES, and other encryption algorithms. It is more general-purpose compared to openpgp, which is specifically focused on the OpenPGP standard.
The crypto module is a built-in Node.js module that provides cryptographic functionality, including a set of wrappers for OpenSSL's hash, HMAC, cipher, decipher, sign, and verify functions. It is more low-level compared to openpgp, which provides higher-level abstractions for OpenPGP operations.
kbpgp is a JavaScript library for OpenPGP encryption and decryption, similar to openpgp. It is designed to be compatible with the Keybase platform and provides a simpler API for common OpenPGP operations.
OpenPGP.js is a JavaScript implementation of the OpenPGP protocol. It implements RFC4880 and parts of RFC4880bis.
Table of Contents
The dist/openpgp.min.js
bundle works well with recent versions of Chrome, Firefox, Safari and Edge.
The dist/node/openpgp.min.js
bundle works well in Node.js. It is used by default when you require('openpgp')
in Node.js.
Currently, Chrome, Safari and Edge have partial implementations of the
Streams specification, and Firefox
has a partial implementation behind feature flags. Chrome is the only
browser that implements TransformStream
s, which we need, so we include
a polyfill for
all other browsers. Please note that in those browsers, the global
ReadableStream
property gets overwritten with the polyfill version if
it exists. In some edge cases, you might need to use the native
ReadableStream
(for example when using it to create a Response
object), in which case you should store a reference to it before loading
OpenPGP.js. There is also the
web-streams-adapter
library to convert back and forth between them.
Version 3.0.0 of the library introduces support for public-key cryptography using elliptic curves. We use native implementations on browsers and Node.js when available. Elliptic curve cryptography provides stronger security per bits of key, which allows for much faster operations. Currently the following curves are supported:
Curve | Encryption | Signature | NodeCrypto | WebCrypto | Constant-Time |
---|---|---|---|---|---|
curve25519 | ECDH | N/A | No | No | Algorithmically** |
ed25519 | N/A | EdDSA | No | No | Algorithmically** |
p256 | ECDH | ECDSA | Yes* | Yes* | If native*** |
p384 | ECDH | ECDSA | Yes* | Yes* | If native*** |
p521 | ECDH | ECDSA | Yes* | Yes* | If native*** |
brainpoolP256r1 | ECDH | ECDSA | Yes* | No | If native*** |
brainpoolP384r1 | ECDH | ECDSA | Yes* | No | If native*** |
brainpoolP512r1 | ECDH | ECDSA | Yes* | No | If native*** |
secp256k1 | ECDH | ECDSA | Yes* | No | If native*** |
* when available
** the curve25519 and ed25519 implementations are algorithmically constant-time, but may not be constant-time after optimizations of the JavaScript compiler
*** these curves are only constant-time if the underlying native implementation is available and constant-time
Version 2.x of the library has been built from the ground up with Uint8Arrays. This allows for much better performance and memory usage than strings.
If the user's browser supports native WebCrypto via the window.crypto.subtle
API, this will be used. Under Node.js the native crypto module is used.
The library implements the RFC4880bis proposal for authenticated encryption using native AES-EAX, OCB, or GCM. This makes symmetric encryption up to 30x faster on supported platforms. Since the specification has not been finalized and other OpenPGP implementations haven't adopted it yet, the feature is currently behind a flag. Note: activating this setting can break compatibility with other OpenPGP implementations, and also with future versions of OpenPGP.js. Don't use it with messages you want to store on disk or in a database. You can enable it by setting openpgp.config.aeadProtect = true
.
You can change the AEAD mode by setting one of the following options:
openpgp.config.preferredAEADAlgorithm = openpgp.enums.aead.eax // Default, native
openpgp.config.preferredAEADAlgorithm = openpgp.enums.aead.ocb // Non-native
openpgp.config.preferredAEADAlgorithm = openpgp.enums.aead.experimentalGCM // **Non-standard**, fastest
For environments that don't provide native crypto, the library falls back to asm.js implementations of AES, SHA-1, and SHA-256.
Install OpenPGP.js using npm and save it in your dependencies:
npm install --save openpgp
And import it as a CommonJS module:
const openpgp = require('openpgp');
Or as an ES6 module, from an .mjs file:
import * as openpgp from 'openpgp';
Import as an ES6 module, using /dist/openpgp.mjs.
import * as openpgp from './openpgpjs/dist/openpgp.mjs';
Install OpenPGP.js using npm and save it in your devDependencies:
npm install --save-dev openpgp
And import it as an ES6 module:
import * as openpgp from 'openpgp';
You can also only import the functions you need, as follows:
import { readMessage, decrypt } from 'openpgp';
Or, if you want to use the lightweight build (which is smaller, and lazily loads non-default curves on demand):
import * as openpgp from 'openpgp/lightweight';
To test whether the lazy loading works, try to generate a key with a non-standard curve:
import { generateKey } from 'openpgp/lightweight';
await generateKey({ curve: 'brainpoolP512r1', userIDs: [{ name: 'Test', email: 'test@test.com' }] });
For more examples of how to generate a key, see Generate new key pair. It is recommended to use curve25519
instead of brainpoolP512r1
by default.
Grab openpgp.min.js
from unpkg.com/openpgp/dist, and load it in a script tag:
<script src="openpgp.min.js"></script>
Or, to load OpenPGP.js as an ES6 module, grab openpgp.min.mjs
from unpkg.com/openpgp/dist, and import it as follows:
<script type="module">
import * as openpgp from './openpgp.min.mjs';
</script>
To offload cryptographic operations off the main thread, you can implement a Web Worker in your application and load OpenPGP.js from there. For an example Worker implementation, see test/worker/worker_example.js
.
Since TS is not fully integrated in the library, TS-only dependencies are currently listed as devDependencies
, so to compile the project you’ll need to add @openpgp/web-stream-tools
manually (NB: only versions below v0.12 are compatible with OpenPGP.js v5):
npm install --save-dev @openpgp/web-stream-tools@0.0.11-patch-0
If you notice missing or incorrect type definitions, feel free to open a PR.
Here are some examples of how to use OpenPGP.js v5. For more elaborate examples and working code, please check out the public API unit tests. If you're upgrading from v4 it might help to check out the changelog and documentation.
Encryption will use the algorithm specified in config.preferredSymmetricAlgorithm (defaults to aes256), and decryption will use the algorithm used for encryption.
(async () => {
const message = await openpgp.createMessage({ binary: new Uint8Array([0x01, 0x01, 0x01]) });
const encrypted = await openpgp.encrypt({
message, // input as Message object
passwords: ['secret stuff'], // multiple passwords possible
format: 'binary' // don't ASCII armor (for Uint8Array output)
});
console.log(encrypted); // Uint8Array
const encryptedMessage = await openpgp.readMessage({
binaryMessage: encrypted // parse encrypted bytes
});
const { data: decrypted } = await openpgp.decrypt({
message: encryptedMessage,
passwords: ['secret stuff'], // decrypt with password
format: 'binary' // output as Uint8Array
});
console.log(decrypted); // Uint8Array([0x01, 0x01, 0x01])
})();
Encryption will use the algorithm preferred by the public (encryption) key (defaults to aes256 for keys generated in OpenPGP.js), and decryption will use the algorithm used for encryption.
const openpgp = require('openpgp'); // use as CommonJS, AMD, ES6 module or via window.openpgp
(async () => {
// put keys in backtick (``) to avoid errors caused by spaces or tabs
const publicKeyArmored = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`;
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // encrypted private key
const passphrase = `yourPassphrase`; // what the private key is encrypted with
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }),
passphrase
});
const encrypted = await openpgp.encrypt({
message: await openpgp.createMessage({ text: 'Hello, World!' }), // input as Message object
encryptionKeys: publicKey,
signingKeys: privateKey // optional
});
console.log(encrypted); // '-----BEGIN PGP MESSAGE ... END PGP MESSAGE-----'
const message = await openpgp.readMessage({
armoredMessage: encrypted // parse armored message
});
const { data: decrypted, signatures } = await openpgp.decrypt({
message,
verificationKeys: publicKey, // optional
decryptionKeys: privateKey
});
console.log(decrypted); // 'Hello, World!'
// check signature validity (signed messages only)
try {
await signatures[0].verified; // throws on invalid signature
console.log('Signature is valid');
} catch (e) {
throw new Error('Signature could not be verified: ' + e.message);
}
})();
Encrypt to multiple public keys:
(async () => {
const publicKeysArmored = [
`-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`,
`-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`
];
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // encrypted private key
const passphrase = `yourPassphrase`; // what the private key is encrypted with
const plaintext = 'Hello, World!';
const publicKeys = await Promise.all(publicKeysArmored.map(armoredKey => openpgp.readKey({ armoredKey })));
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readKey({ armoredKey: privateKeyArmored }),
passphrase
});
const message = await openpgp.createMessage({ text: plaintext });
const encrypted = await openpgp.encrypt({
message, // input as Message object
encryptionKeys: publicKeys,
signingKeys: privateKey // optional
});
console.log(encrypted); // '-----BEGIN PGP MESSAGE ... END PGP MESSAGE-----'
})();
If you expect an encrypted message to be signed with one of the public keys you have, and do not want to trust the decrypted data otherwise, you can pass the decryption option expectSigned = true
, so that the decryption operation will fail if no valid signature is found:
(async () => {
// put keys in backtick (``) to avoid errors caused by spaces or tabs
const publicKeyArmored = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`;
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // encrypted private key
const passphrase = `yourPassphrase`; // what the private key is encrypted with
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }),
passphrase
});
const encryptedAndSignedMessage = `-----BEGIN PGP MESSAGE-----
...
-----END PGP MESSAGE-----`;
const message = await openpgp.readMessage({
armoredMessage: encryptedAndSignedMessage // parse armored message
});
// decryption will fail if all signatures are invalid or missing
const { data: decrypted, signatures } = await openpgp.decrypt({
message,
decryptionKeys: privateKey,
expectSigned: true,
verificationKeys: publicKey, // mandatory with expectSigned=true
});
console.log(decrypted); // 'Hello, World!'
})();
By default, encrypt
will not use any compression when encrypting symmetrically only (i.e. when no encryptionKeys
are given).
It's possible to change that behaviour by enabling compression through the config, either for the single encryption:
(async () => {
const message = await openpgp.createMessage({ binary: new Uint8Array([0x01, 0x02, 0x03]) }); // or createMessage({ text: 'string' })
const encrypted = await openpgp.encrypt({
message,
passwords: ['secret stuff'], // multiple passwords possible
config: { preferredCompressionAlgorithm: openpgp.enums.compression.zlib } // compress the data with zlib
});
})();
or by changing the default global configuration:
openpgp.config.preferredCompressionAlgorithm = openpgp.enums.compression.zlib
Where the value can be any of:
openpgp.enums.compression.zip
openpgp.enums.compression.zlib
openpgp.enums.compression.uncompressed
(default)(async () => {
const readableStream = new ReadableStream({
start(controller) {
controller.enqueue(new Uint8Array([0x01, 0x02, 0x03]));
controller.close();
}
});
const message = await openpgp.createMessage({ binary: readableStream });
const encrypted = await openpgp.encrypt({
message, // input as Message object
passwords: ['secret stuff'], // multiple passwords possible
format: 'binary' // don't ASCII armor (for Uint8Array output)
});
console.log(encrypted); // raw encrypted packets as ReadableStream<Uint8Array>
// Either pipe the above stream somewhere, pass it to another function,
// or read it manually as follows:
for await (const chunk of encrypted) {
console.log('new chunk:', chunk); // Uint8Array
}
})();
For more information on using ReadableStreams, see the MDN Documentation on the Streams API.
You can also pass a Node.js Readable
stream, in
which case OpenPGP.js will return a Node.js Readable
stream as well, which you
can .pipe()
to a Writable
stream, for example.
(async () => {
const publicKeyArmored = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`; // Public key
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // Encrypted private key
const passphrase = `yourPassphrase`; // Password that private key is encrypted with
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }),
passphrase
});
const readableStream = new ReadableStream({
start(controller) {
controller.enqueue('Hello, world!');
controller.close();
}
});
const encrypted = await openpgp.encrypt({
message: await openpgp.createMessage({ text: readableStream }), // input as Message object
encryptionKeys: publicKey,
signingKeys: privateKey // optional
});
console.log(encrypted); // ReadableStream containing '-----BEGIN PGP MESSAGE ... END PGP MESSAGE-----'
const message = await openpgp.readMessage({
armoredMessage: encrypted // parse armored message
});
const decrypted = await openpgp.decrypt({
message,
verificationKeys: publicKey, // optional
decryptionKeys: privateKey
});
const chunks = [];
for await (const chunk of decrypted.data) {
chunks.push(chunk);
}
const plaintext = chunks.join('');
console.log(plaintext); // 'Hello, World!'
})();
ECC keys (smaller and faster to generate):
Possible values for curve
are: curve25519
, ed25519
, p256
, p384
, p521
,
brainpoolP256r1
, brainpoolP384r1
, brainpoolP512r1
, and secp256k1
.
Note that both the curve25519
and ed25519
options generate a primary key for signing using Ed25519
and a subkey for encryption using Curve25519.
(async () => {
const { privateKey, publicKey, revocationCertificate } = await openpgp.generateKey({
type: 'ecc', // Type of the key, defaults to ECC
curve: 'curve25519', // ECC curve name, defaults to curve25519
userIDs: [{ name: 'Jon Smith', email: 'jon@example.com' }], // you can pass multiple user IDs
passphrase: 'super long and hard to guess secret', // protects the private key
format: 'armored' // output key format, defaults to 'armored' (other options: 'binary' or 'object')
});
console.log(privateKey); // '-----BEGIN PGP PRIVATE KEY BLOCK ... '
console.log(publicKey); // '-----BEGIN PGP PUBLIC KEY BLOCK ... '
console.log(revocationCertificate); // '-----BEGIN PGP PUBLIC KEY BLOCK ... '
})();
RSA keys (increased compatibility):
(async () => {
const { privateKey, publicKey } = await openpgp.generateKey({
type: 'rsa', // Type of the key
rsaBits: 4096, // RSA key size (defaults to 4096 bits)
userIDs: [{ name: 'Jon Smith', email: 'jon@example.com' }], // you can pass multiple user IDs
passphrase: 'super long and hard to guess secret' // protects the private key
});
})();
Using a revocation certificate:
(async () => {
const { publicKey: revokedKeyArmored } = await openpgp.revokeKey({
key: await openpgp.readKey({ armoredKey: publicKeyArmored }),
revocationCertificate,
format: 'armored' // output armored keys
});
console.log(revokedKeyArmored); // '-----BEGIN PGP PUBLIC KEY BLOCK ... '
})();
Using the private key:
(async () => {
const { publicKey: revokedKeyArmored } = await openpgp.revokeKey({
key: await openpgp.readKey({ armoredKey: privateKeyArmored }),
format: 'armored' // output armored keys
});
console.log(revokedKeyArmored); // '-----BEGIN PGP PUBLIC KEY BLOCK ... '
})();
(async () => {
const publicKeyArmored = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`;
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // encrypted private key
const passphrase = `yourPassphrase`; // what the private key is encrypted with
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }),
passphrase
});
const unsignedMessage = await openpgp.createCleartextMessage({ text: 'Hello, World!' });
const cleartextMessage = await openpgp.sign({
message: unsignedMessage, // CleartextMessage or Message object
signingKeys: privateKey
});
console.log(cleartextMessage); // '-----BEGIN PGP SIGNED MESSAGE ... END PGP SIGNATURE-----'
const signedMessage = await openpgp.readCleartextMessage({
cleartextMessage // parse armored message
});
const verificationResult = await openpgp.verify({
message: signedMessage,
verificationKeys: publicKey
});
const { verified, keyID } = verificationResult.signatures[0];
try {
await verified; // throws on invalid signature
console.log('Signed by key id ' + keyID.toHex());
} catch (e) {
throw new Error('Signature could not be verified: ' + e.message);
}
})();
(async () => {
const publicKeyArmored = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`;
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // encrypted private key
const passphrase = `yourPassphrase`; // what the private key is encrypted with
const publicKey = await openpgp.readKey({ armoredKey: publicKeyArmored });
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }),
passphrase
});
const message = await openpgp.createMessage({ text: 'Hello, World!' });
const detachedSignature = await openpgp.sign({
message, // Message object
signingKeys: privateKey,
detached: true
});
console.log(detachedSignature);
const signature = await openpgp.readSignature({
armoredSignature: detachedSignature // parse detached signature
});
const verificationResult = await openpgp.verify({
message, // Message object
signature,
verificationKeys: publicKey
});
const { verified, keyID } = verificationResult.signatures[0];
try {
await verified; // throws on invalid signature
console.log('Signed by key id ' + keyID.toHex());
} catch (e) {
throw new Error('Signature could not be verified: ' + e.message);
}
})();
(async () => {
var readableStream = new ReadableStream({
start(controller) {
controller.enqueue(new Uint8Array([0x01, 0x02, 0x03]));
controller.close();
}
});
const publicKeyArmored = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`;
const privateKeyArmored = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`; // encrypted private key
const passphrase = `yourPassphrase`; // what the private key is encrypted with
const privateKey = await openpgp.decryptKey({
privateKey: await openpgp.readPrivateKey({ armoredKey: privateKeyArmored }),
passphrase
});
const message = await openpgp.createMessage({ binary: readableStream }); // or createMessage({ text: ReadableStream<String> })
const signatureArmored = await openpgp.sign({
message,
signingKeys: privateKey
});
console.log(signatureArmored); // ReadableStream containing '-----BEGIN PGP MESSAGE ... END PGP MESSAGE-----'
const verificationResult = await openpgp.verify({
message: await openpgp.readMessage({ armoredMessage: signatureArmored }), // parse armored signature
verificationKeys: await openpgp.readKey({ armoredKey: publicKeyArmored })
});
for await (const chunk of verificationResult.data) {}
// Note: you *have* to read `verificationResult.data` in some way or other,
// even if you don't need it, as that is what triggers the
// verification of the data.
try {
await verificationResult.signatures[0].verified; // throws on invalid signature
console.log('Signed by key id ' + verificationResult.signatures[0].keyID.toHex());
} catch (e) {
throw new Error('Signature could not be verified: ' + e.message);
}
})();
The full documentation is available at openpgpjs.org.
To date the OpenPGP.js code base has undergone two complete security audits from Cure53. The first audit's report has been published here.
It should be noted that js crypto apps deployed via regular web hosting (a.k.a. host-based security) provide users with less security than installable apps with auditable static versions. Installable apps can be deployed as a Firefox or Chrome packaged app. These apps are basically signed zip files and their runtimes typically enforce a strict Content Security Policy (CSP) to protect users against XSS. This blogpost explains the trust model of the web quite well.
It is also recommended to set a strong passphrase that protects the user's private key on disk.
To create your own build of the library, just run the following command after cloning the git repo. This will download all dependencies, run the tests and create a minified bundle under dist/openpgp.min.js
to use in your project:
npm install && npm test
For debugging browser errors, you can run npm start
and open http://localhost:8080/test/unittests.html
in a browser, or run the following command:
npm run browsertest
You want to help, great! It's probably best to send us a message on Gitter before you start your undertaking, to make sure nobody else is working on it, and so we can discuss the best course of action. Other than that, just go ahead and fork our repo, make your changes and send us a pull request! :)
GNU Lesser General Public License (3.0 or any later version). Please take a look at the LICENSE file for more information.
FAQs
OpenPGP.js is a Javascript implementation of the OpenPGP protocol. This is defined in RFC 4880.
The npm package openpgp receives a total of 286,518 weekly downloads. As such, openpgp popularity was classified as popular.
We found that openpgp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.