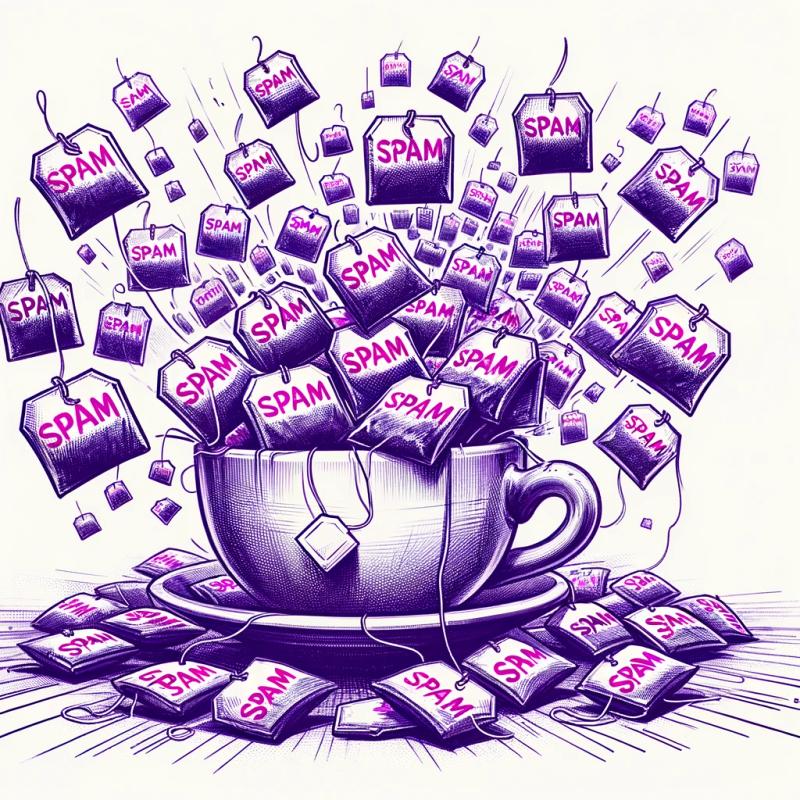
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
openweathermap-api-module
Advanced tools
Readme
Simple Node module to call the openweathermap API
This wrapper is based on the free tier of OpenWeatherMap API. You can get your API key here.
$ npm install openweathermap-api-module
const OpenWeatherMapRequests = require('openweathermap-api-module')
const apiKey = 'api key'
const client = new OpenWeatherMapRequests(apiKey)
client.currentWeatherByCityName('boston').then(console.log).catch(console.error)
Check out the Openweathermap API documentation for more information!
Mixin for handling API requests options
const client = new OpenWeatherMapRequest(ApiKey, {})
Call Current weather for One location by city Name
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherByCityName({cityName: 'boston,us'})
client.currentWeatherByCityName({cityName: 'boston,us', lang: 'fr'})
Returns Object response - Object with data from request Type
Mixin for handling API requests options
args
Call Current weather for One location by city ID
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherByCityId({cityId: 2643743})
client.currentWeatherByCityId({cityId: '2643743', units: 'metric'})
Returns Object response - Object with data from request Type
Call Current weather for One location by Geographic Coordinates
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherByGeoCoordinates({coordinates: { lon:'139.01', lat:'35.02' } })
client.currentWeatherByGeoCoordinates({coordinates: { lon:'139.01', lat:'35.02' }, lang: 'fr' })
Returns Object response - Object with data from request Type
Call Current weather for One location by Zipcode
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherByZipCode({ zipCode: '90247,us' })
client.currentWeatherByZipCode({ zipCode: '90247,us', lang: 'fr' })
Returns Object response - Object with data from request Type
Call Current weather for many locations by Rectangle Box
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherByRectangleZone({ bbox: { lonLeft: '12', latBottom: '32', lonRight: '15',
latTop: '37', zoom: '10' } })
client.currentWeatherByRectangleZone({ bbox: { lonLeft: '12', latBottom: '32', lonRight: '15',
latTop: '37', zoom: '10' }, units: 'imperial' })
Returns Object response - Object with data from request Type
Call Current weather for many locations by Circle Radius
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherByCircleZone({ circle: { lat: 55.5, lon: 37, cnt: 10 } })
client.currentWeatherByCircleZone({ circle: { lat: 55.5, lon: 37, cnt: 10 }, format: 'html' })
Returns Object response - Object with data from request Type
Call Current weather for many locations by City Ids
args
Object Arguments for mixin handler
const client = new OpenWeatherMapRequests(API_KEY)
client.currentWeatherBy({ cityIds: [524901,703448,2643743] })
client.currentWeatherBy({ cityIds: [524901,703448,2643743], lang: 'fr' })
Returns Object response - Object with data from request Type
Current Weather API handler. Key is inherited from OpenWeatherMapRequests
apiKey
baseUrl
baseUrl-null
String API base URL from OpenWeatherMap APIconst client = new WeatherApiHandler('Api_key','base_url')
Call Current weather for One location by city Name
// api.openweathermap.org/data/2.5/weather?q=London
// api.openweathermap.org/data/2.5/weather?q=London,uk
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getLocationByCityName('boston')
client.getLocationByCityName('London,uk')
client.getLocationByCityName('tokyo', { units: 'imperial' })
client.getLocationByCityName('tokyo', { format: 'xml', units: 'imperial', lang='fr' })
Returns Object requestResponse - Return Object from API call
Call Current weather for One location by ID
cityId
(String | Number) Id of the Cityargs
(optional, default {}
)options
Object? options for request
// api.openweathermap.org/data/2.5/weather?id=2643743
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getLocationByCityId(2643743)
client.getLocationByCityId('2643743')
Returns Object requestResponse - Return Object from API call
Call Current weather for One location by Coordinates
coordinates
Object Object including Coordinates. Ex: { lat: 139.01, lon: 35.02 }
args
(optional, default {}
)options
Object? options for request
// api.openweathermap.org/data/2.5/weather?lat=35&lon=139
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getLocationByGeoCoordinates({lon: 139.01, lat: 35.02})
client.getLocationByGeoCoordinates({lon: 139.01, lat: 35.02}, { format: 'xml' })
Returns Object requestResponse - Return Object from API call
Call Current weather for One location by Zip Codes
zipCode
args
(optional, default {}
)zipcode
String Zipcode from Country, ex: 'zipcode,country' (optional, default "90247,us"
)options
Object? options for request
// api.openweathermap.org/data/2.5/weather?zip=94040,us
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getLocationByZipCode('94040,us')
Returns Object requestResponse - Return Object from API call
Call current weather data for several cities using a Bounding Box bbox bounding box [lon-left,lat-bottom,lon-right,lat-top,zoom]
bbox
Object Bounding Box for location. Example: bbox={ lonLeft: '0', latBottom: '0', lonRight: '0', latTop: '0', zoom: '0' }args
(optional, default {}
)options
Object? options for request
// api.openweathermap.org/data/2.5//box/city?bbox=12,32,15,37,10
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getManyLocationsByRectangleZone({ lonLeft: '12', latBottom: '32', lonRight: '15',
latTop: '37', zoom: '10' })
client.getManyLocationsByRectangleZone({ lonLeft: '12', latBottom: '32', lonRight: '15',
latTop: '37', zoom: '10' }, { units: 'metric' })
Returns Object requestResponse - Return Object from API call
Call current weather data for several cities using a Longitude and Latitude and get all cities around it
circle
Object Circle object with longitude, latitude and number of cities to show ex: { lat: 55.5, lon: 37, cnt: 10 }args
(optional, default {}
)options
Object? options for request
// api.openweathermap.org/data/2.5//box/city?lat=55.5&lon37.5&cnt=10
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getManyLocationsByCircleZone({ lat: 55.5, lon: 37, cnt: 10 })
client.getManyLocationsByCircleZone({ lat: 55.5, lon: 37, cnt: 10 }, { lang: 'fr' })
Returns Object requestResponse - Return Object from API call
Call for several city IDs
cityIds
args
(optional, default {}
)citiIds
Array Array filled with city IDs, ex: [524901,703448,2643743]options
Object? options for request
// http://api.openweathermap.org/data/2.5/group?id=524901,703448,2643743
const client = new CurrentWeatherHandler(API_KEY, BASE_URL)
client.getManyLocationsByRectangleZone([524901,703448,2643743])
client.getManyLocationsByRectangleZone([524901,703448,2643743], { lang: 'fr' })
Returns Object requestResponse - Return Object from API call
FAQs
Simple Node module to call the openweathermap API
The npm package openweathermap-api-module receives a total of 0 weekly downloads. As such, openweathermap-api-module popularity was classified as not popular.
We found that openweathermap-api-module demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.