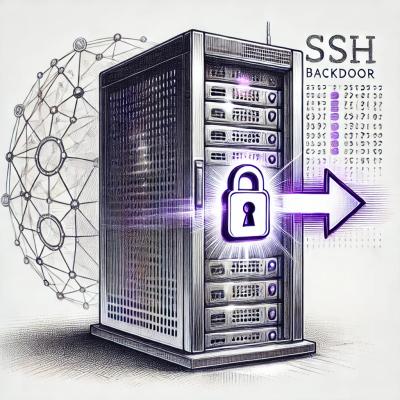
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Orion allows you to build a REST API app in just a few steps! This library is to be used in combination with Node and Express. It sets up all the necessary CRUD data endpoints, file uploads, authentication endpoints, and error handling.
The latest version of the library supports the following components:
In this documentation:
$ npm install --save express
$ npm install --save orion-api
$ node node_modules/orion-api/setup.js ./config.js ./setup.sql
The above command will create a SQL server query file named setup.sql that you can run on the
database server to set up the tables.var express = require('express');
var orion = require('orion-api');
var config = require('./config');
var app = new express();
orion.setConfig(config);
orion.setupApiEndpoints(app);
orion.startApiApp(app);
$ node server.js
$ # your app should now run at http://localhost:1337
A configuration module is required to give the application the necessary information about your project. This module should specify the connection strings, authentication and authorization settings, user roles and access levels, and most importantly, the data models.
Below is the structure of a configuration module, with description for each property.
module.exports =
{
// (Required) Secret key string for authentication purposes.
secretKey: __SECRETKEY__,
// (Required) Salt string for encrypting passwords.
salt: __SALT__,
// (Required) Database connection string.
databaseConnectionString: __DB_CONNECTION_STRING__,
// (Optional) Azure Blob Storage connection string. Required if you want to support
// file upload. Set to null if not applicable.
storageConnectionString: __STORAGE_CONNECTION_STRING__,
// (Optional) Azure Blob Storage account name. Required if you want to support
// file upload. Set to null if not applicable.
storageContainerName: __STORAGE_CONTAINER_NAME__,
// (Optional) Azure Application Insights key. Required if you want to support
// monitoring. Set to null if not applicable.
appInsightsKey: __APPLICATION_INSIGHTS_KEY__,
// (Optional) Facebook app secret. Required if you want to support Facebook
// authentication. Set to null if not applicable.
facebookAppSecret: __FACEBOOK_APP_SECRET__,
// (Optional) Facebook app ID. Required if you want to support Facebook
// authentication. Set to null if not applicable.
facebookAppId: __FACEBOOK_APP_ID__,
// (Required) Requirements for new user passwords
passwordReqs:
{
// (Required) Minimum character length
minLength: 8,
// (Required) Whether or not the password should have an uppercase character.
uppercaseChar: false,
// (Required) Whether or not the password should have an lowercase character.
lowercaseChar: false,
// (Required) Whether or not the password should have a digit character.
digitChar: false,
// (Required) Whether or not the password should have a special character.
specialChar: false
},
// (Required) Array of user roles.
// "guest" and "owner" are special user roles that don't need to be included in this list.
// "guest" role is given to an unauthenticated user, and "owner" role is given to an
// authenticated user who is requesting a resource that they own.
roles: ["member", "admin"],
// (Required) Default role assigned to authenticated user after signing up.
defaultRole: "member",
// (Required) List of data entities/tables
entities:
{
// The key to each object here will be the entity name. We suggest that you use
// singular noun form as the entity name, so it will make good sense when it is
// is used in the endpoint URL.
"item":
{
// (Required) The list of fields in the entity/table
fields:
{
// The key to each object here will be the field name. We suggest that you
// use all lowercase for the field name.
// (Required) id of the resource. This has to have the type "id" and will be
// given the primary key constraint. See the ownerid field for more details on
// the properties inside the field object.
"id": { type: "id", isEditable: false, createReq: 0, foreignKey: null },
// (Required) Owner of the resource. When the resource is created, this field
// will be set automatically to the creator ID.
"ownerid":
{
// (Required) the type of the field
// The supported types are:
// "id" : ID of the resource, primary key, needs to be unique.
// "string" : plain text, max 255 characters. default to null.
// "text" : rich text, no maximum. default to null. Note that this
// field will only be returned on individual item retrieval.
// "int" : integer. default to 0.
// "float" : floating point numbers. default to null.
// "boolean" : boolean type (0/1). default to 0.
// "timestamp" : timestamp type. Will be stored in JS's integer time
// format (the number of ms since Jan 1, 1970).
type: "int",
// (Required) whether or not this field is editable in PUT action.
isEditable: false,
// (Required) specify the requirement for this field in POST action.
// Possible values:
// 0 : This field is not required in POST body.
// 1 : This field is optional and may be included in POST body.
// 2 : This field is required in POST body. Failure to supply this value
// in POST will result in an error response.
createReq: 0,
// (Required) Specify whether this field is a foreign key to another
// entity. Set to null if it is not.
foreignKey:
{
// (Required) Name of the entity that this field is referring to.
// For example, the field we're at now is the ownerid field, which
// refers to the user entity.
foreignEntity: "user",
// (Required) The field name shown after the foreign key is resolved.
// The GET handler resolves 1 foreign key level. So if you for instance
// make a findbyid request to this item entity, the app will resolve
// this ownerid field (since it is marked as a foreign key) and replace
// it with a user object. The value of this resolvedKeyName will be
// the key to that object, so the output will be like:
// { "id": "1", "owner": { "id": 2", ... }, ... }
resolvedKeyName: "owner"
}
},
// (Optional) Timestamp of the resource creator. When the resource is created,
// this field will be set automatically to the time of the creation.
"createdtime": { type: "timestamp", isEditable: false, createReq: 0, foreignKey: null }
// You can add more fields to the entity
"name": { type: "string", isEditable: true, createReq: 2, foreignKey: null },
"date": { type: "int", isEditable: true, createReq: 2, foreignKey: null }
},
// (Required) Condition strings to be appended to read queries, based on who is
// requesting it. Roles that are not listed here won't have read access to the
// entity.
readConditionStrings:
[
// Each object in this array represents a rule that is applied to a certain
// set of user roles.
{
// (Required) A set of user roles that this rule applies to.
// For GET requests, "owner" role is given automatically in private mode.
// This automatically adds ownerid=[activeUserId] condition to the database
// read operation.
roles: ["owner", "admin"],
// (Required) A function that returns the condition string.
// This function takes the active user as argument.
// If this returns empty string return, no condition will be added so all
// requested data will be returned.
fn: function(u) { return ""; }
}
],
// (Required) Validator functions to check if a POST/PUT/DELETE request is valid
// based on who is requesting it. Roles that are not listed here won't have POST/
// PUT/DELETE access to the entity.
validators:
{
// (Required) Validator functions for POST requests.
create:
[
// Each object in this array represents a rule that is applied to a
// certain set of user roles.
{
// (Required) A set of user roles that this rule applies to.
roles: ["member"],
// (Required) The validator function. Based on the supplied arguments,
// this should return true if the request is valid, and false
// otherwise. The validator for POST takes one argument, the newly
// submitted object.
fn: function(n) { return true; }
}
],
// (Required) Validator functions for PUT requests.
update:
[
{
roles: ["owner", "admin"],
// The validator for PUT takes three arguments, the active user object,
// the current data object, and the updated data object.
fn: function(u, o, n) { return true; }
}
],
// (Required) Validator functions for DELETE requests.
delete:
[
{
roles: ["owner", "admin"],
// The validator for DELETE takes one argument, the data object to be
// deleted.
fn: function(o) { return true; }
}
]
}
},
// This is a special entity/table for storing user data. This is required if you want
// to enable authentication. All fields listed here are required, and you can add more
// to it if needed. You can also modify the condition strings and the validators.
"user":
{
fields:
{
"id": { type: "id", isEditable: false, createReq: 0, foreignKey: null },
"domain": { type: "string", isEditable: false, createReq: 0, foreignKey: null },
"domainid": { type: "string", isEditable: false, createReq: 0, foreignKey: null },
"roles": { type: "string", isEditable: false, createReq: 0, foreignKey: null },
"username": { type: "string", isEditable: true, createReq: 2, foreignKey: null },
"password": { type: "secret", isEditable: false, createReq: 2, foreignKey: null },
"email": { type: "string", isEditable: true, createReq: 2, foreignKey: null },
"firstname": { type: "string", isEditable: true, createReq: 2, foreignKey: null },
"lastname": { type: "string", isEditable: true, createReq: 2, foreignKey: null },
"createdtime": { type: "timestamp", isEditable: false, createReq: 0, foreignKey: null }
},
readConditionStrings: [{ roles: ["member", "owner", "admin"], fn: function(u) { return ""; } }],
validators:
{
create: [{ roles: ["guest"], fn: function(n) { return true; } }],
update: [{ roles: ["owner", "admin"], fn: function(u, o, n) { return true; } }],
delete: [{ roles: ["admin"], fn: function(o) { return true; } }]
}
},
// This is another special entity/table that is used for keeping track of uploaded
// files. It is required if you want to enable file upload. All fields listed here
// are required. You can modify the condition strings and the validators if needed.
"asset":
{
fields:
{
"id": { type: "id", isEditable: false, createReq: 0, foreignKey: null },
"ownerid": { type: "int", isEditable: false, createReq: 0, foreignKey: { foreignEntity: "user", resolvedKeyName: "owner" }},
"filename": { type: "string", isEditable: true, createReq: 2, foreignKey: null }
},
readConditionStrings: [{ roles: ["owner", "admin"], fn: function(u) { return ""; } }],
validators:
{
create: [{ roles: ["member"], fn: function(n) { return true; } }],
update: [],
delete: [{ roles: ["owner", "admin"], fn: function(o) { return true; } }]
}
},
// You can add as many more entities as needed. This one below is an example entity
// that represents a chat message, to help you better understand how to use the
// condition strings and validators to control resource access.
"message":
{
fields:
{
// We are setting all fields to be uneditable, except for the flagged field,
// because we want to let people flag inappropriate messages.
"id": { type: "id", isEditable: false, createReq: 0, foreignKey: null },
"ownerid": { type: "int", isEditable: false, createReq: 0, foreignKey: { foreignEntity: "user", resolvedKeyName: "owner" }},
"recipientid": { type: "int", isEditable: false, createReq: 2, foreignKey: { foreignEntity: "user", resolvedKeyName: "recipient" }},
"text": { type: "string", isEditable: false, createReq: 2, foreignKey: null },
"flagged": { type: "boolean", isEditable: true, createReq: 0, foreignKey: null },
"createdtime": { type: "timestamp", isEditable: false, createReq: 0, foreignKey: null }
},
readConditionStrings:
[
// Unauthenticated users (guest) cannot access private chat messages, so guest
// role is not listed here. Regular authenticated users (member) is allowed to
// access only messages that they send or receive. So for member role we're
// adding a condition string to make sure the ownerid or recipientid
// matches the active user id.
{
roles: ["member"],
fn: function(u) { return "ownerid=" + u + "|recipientid=" + u; }
},
// Admin role has full access to all chat messages.
{ roles: ["admin"], fn: function(u) { return ""; } }
],
validators:
{
// Same as before, unauthenticated users (guest) cannot modify private chat
// messages, so guest role is not listed here. Regular logged in users (member)
// are allowed to create messages so we're returning true here.
create: [{ roles: ["member"], fn: function(n) { return true; } }],
// We are allowing only chat recipients to flag a message. Therefore for PUT
// requests we are checking if the active user is the chat recipient. If not
// then the request is invalid.
update: [{ roles: ["member"], fn: function(u, o, n) { return u === o.recipientid; } }],
// We are only allowing admins to delete messages.
delete: [{ roles: ["admin"], fn: function(o) { return true; } }]
}
}
}
};
(The MIT License)
Copyright (c) 2017 Christopher Tjong christophertjong@hotmail.com
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Config based REST API framework
The npm package orion-api receives a total of 0 weekly downloads. As such, orion-api popularity was classified as not popular.
We found that orion-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.