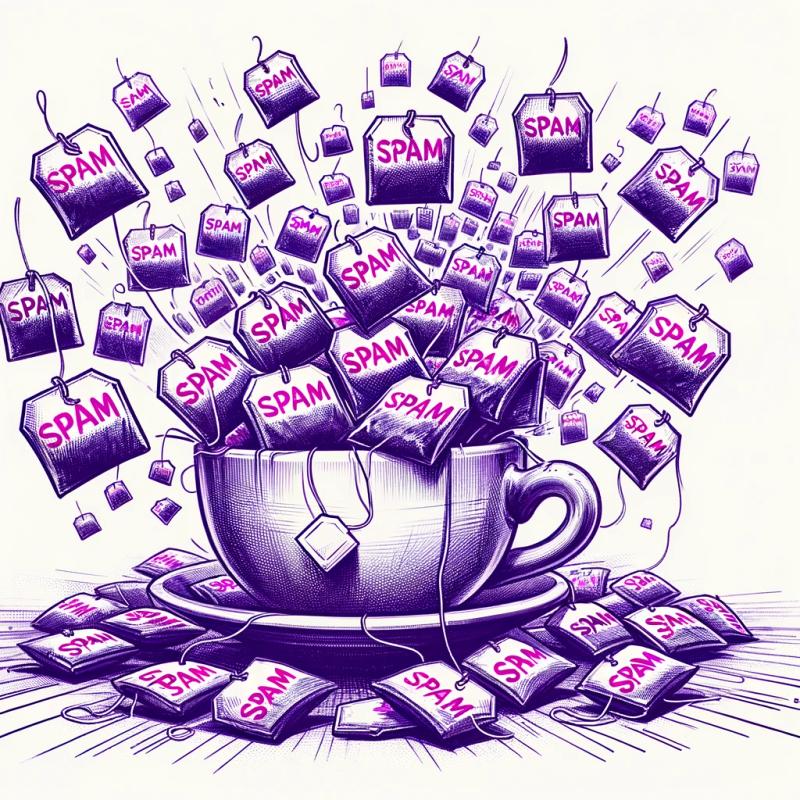
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
Command line argument parser with format inspired by esbuild
--format esm
or short -f esm
-v
, -vPfz
or -vvv
--format:ts esm
or -f:ts esm
--external:fs
or short -e:fs
Supports sub-commands, see below.
Install otps
using pnpm:
pnpm add otps
Or npm:
npm install --save otps
Let's get started by writing a simple command line application that takes one
parameter named format
(or f
for short).
First, create a app.js
file:
import { parse, Value } from 'otps'
const [params, args] = parse(process.argv.slice(2), {
format: Value(),
f: 'format',
})
Then run the program:
node app.js --format esm index.ts
# => params: { format: 'esm' }
# args: ['index.ts']
Or equivalent:
node app.js -f esm index.ts
# => params: { format: 'esm' }
# args: ['index.ts']
Let's create a more advanced app that uses a verbose
parameter (with a v
alias) and a sub-commands build
with a level
parameter that takes one of
two values debug
or info
.
Create a subcmdapp.js
file:
import { parse, Flag, OneOf } from 'otps'
const [params, args] = parse(process.argv.slice(2), {
verbose: Flag(),
v: 'verbose',
build: {
level: OneOf('debug', 'info'),
},
})
Then run the program:
node subcmdapp.js -vvv build --level info index.ts
# => params: { verbose: 3, build: { level: 'info' } }
# args: ['index.ts']
cmds
For easier managment of sub-commands, use cmds
.
Create a cmdsapp.js
file:
import { parse, Value, cmds } from 'otps'
const [params, args] = parse(process.argv.slice(2), {
build: {
format: Value(),
production: {
version: Value(),
},
},
})
cmds(params, args)({
build(params, args) {
const format = params.format
cmds(params, args)({
production(params, args) {
const version = params.version
console.log(`production build: ${format}, name: app@${version}`)
console.log(args)
}
}, (params, args) => {
console.log(`development build: ${format}, name: app@dev`, args)
console.log(args)
})
}
})
Then run the program:
node cmdsapp.js build --format esm production --version 1.0 src/index.ts
# => production build: esm, name: app@1.0
# ['src/index.ts']
node cmdsapp.js build --format esm src/index.ts
# => production build: esm, name: app@dev
# ['src/index.ts']
Parameters are defined using the following syntax: { name: Func }
or with aliases { name: Func, n: 'name' }
. Where Func
is one of:
Value()
:
parse(['--format', 'esm'], { format: Value() })
// => { format: 'esm' }
OneOf(string | regex, ...)
:
parse(['--level', 'dEbUg'], { level: OneOf(/^debug$/i, 'info') })
// => { level: 'dEbUg' }
Flag()
:
parse(['-v'], { v: Flag() })
// => { v: true }
parse(['-vvv'], { v: Flag() })
// => { v: 3 }
parse(['-vP'], { v: Flag(), P: Flag() })
// => { v: true, P: true }
Key()
:
parse(['--external:fs'], { external: Key() })
// => { external: { fs: true } }
parse(['--external:fs', '-e:fs'], { external: Key(), e: 'external' })
// => { external: { fs: 2 } }
Key(Value() | OneOf(...))
:
parse(['--define:DEBUG', 'true'], { define: Key(Value()) })
// => { define: { DEBUG: 'true' } }
parse(['--define:LEVEL', 'debug'], { define: Key(OneOf('debug', 'info')) })
// => { define: { LEVEL: 'debug' } }
Maybe(Value() | OneOf(...))
:
parse(['--format'], { format: Maybe(Value()) })
// => { format: true }
parse(['--format', 'esm'], { format: Maybe(Value()) })
// => { format: 'esm' }
parse(['--level'], { level: Maybe(OneOf('debug', 'info')) })
// => { level: true }
parse(['--level', 'info'], { level: Maybe(OneOf('debug', 'info')) })
// => { level: 'info' }
Many(Value() | OneOf(...) | Maybe(...))
:
parse(['--format', 'esm', '--format', 'cjs'], { format: Many(Value()) })
// => { format: ['esm', 'cjs'] }
parse(['--format', '--format', 'cjs'], { format: Many(Maybe(OneOf('esm', 'cjs'))) })
// => { format: [true, 'cjs'] }
FAQs
parse command-line arguments
The npm package otps receives a total of 116 weekly downloads. As such, otps popularity was classified as not popular.
We found that otps demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.