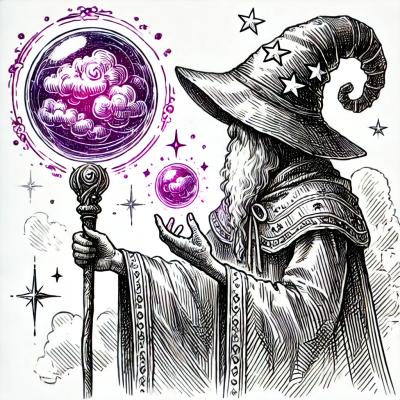
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
The p-pipe npm package allows you to compose promise-returning & async functions into a pipeline. It is useful for creating a sequence of asynchronous operations where the output of one function is passed as the input to the next.
Basic Pipeline
This feature allows you to create a basic pipeline of asynchronous functions. In this example, the `add` function increments the input by 1, and the `multiply` function doubles the result. The pipeline processes the input `5` to produce the output `12`.
const pPipe = require('p-pipe');
const add = async x => x + 1;
const multiply = async x => x * 2;
const pipeline = pPipe(add, multiply);
pipeline(5).then(result => console.log(result)); // 12
Error Handling
This feature demonstrates how p-pipe handles errors in the pipeline. If any function in the pipeline throws an error, the pipeline will stop executing and return the error. In this example, the `throwError` function throws an error, which is caught and logged.
const pPipe = require('p-pipe');
const add = async x => x + 1;
const throwError = async x => { throw new Error('Something went wrong'); };
const multiply = async x => x * 2;
const pipeline = pPipe(add, throwError, multiply);
pipeline(5).catch(error => console.error(error.message)); // 'Something went wrong'
Synchronous Functions
This feature shows that p-pipe can also handle synchronous functions. The pipeline processes the input `5` to produce the output `12`, similar to the asynchronous example.
const pPipe = require('p-pipe');
const add = x => x + 1;
const multiply = x => x * 2;
const pipeline = pPipe(add, multiply);
pipeline(5).then(result => console.log(result)); // 12
p-waterfall is another package for composing promise-returning & async functions. It executes functions in series, passing the result of each function to the next. Unlike p-pipe, p-waterfall is more focused on a waterfall model where each function depends on the result of the previous one.
The async package provides a wide range of utilities for working with asynchronous JavaScript. It includes methods for creating series, parallel, and waterfall workflows. While it is more feature-rich compared to p-pipe, it can be more complex to use for simple pipelines.
promise-pipeline is a package for creating pipelines of promise-returning functions. It is similar to p-pipe but offers additional features like conditional execution and branching. It provides more flexibility at the cost of added complexity.
Compose promise-returning & async functions into a reusable pipeline
$ npm install --save p-pipe
const pPipe = require('p-pipe');
const addUnicorn = str => Promise.resolve(`${str} Unicorn`);
const addRainbow = str => Promise.resolve(`${str} Rainbow`);
const pipeline = pPipe(addUnicorn, addRainbow);
pipeline('❤️').then(console.log);
//=> '❤️ Unicorn Rainbow'
The input
functions are applied from left to right.
Type: Function
Expected to return a Promise
or any value.
MIT © Sindre Sorhus
FAQs
Compose promise-returning & async functions into a reusable pipeline
We found that p-pipe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.