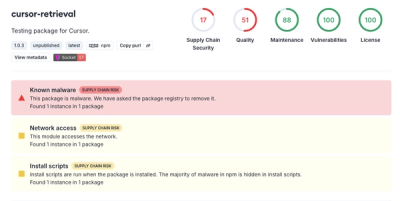
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
p2p-media-loader-hlsjs
Advanced tools
P2P sharing of segmented media streams (i.e. HLS) using WebRTC for Hls.js
Useful links:
General steps are:
P2P Media Loader supports many players that use Hls.js as media engine. Lets pick Clappr just for this example:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Clappr/Hls.js with P2P Media Loader</title>
<meta charset="utf-8">
<script src="https://cdn.jsdelivr.net/npm/p2p-media-loader-core@latest/build/p2p-media-loader-core.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/p2p-media-loader-hlsjs@latest/build/p2p-media-loader-hlsjs.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/clappr@latest"></script>
</head>
<body>
<div id="player"></div>
<script>
if (p2pml.hlsjs.Engine.isSupported()) {
var engine = new p2pml.hlsjs.Engine();
var player = new Clappr.Player({
parentId: "#player",
source: "https://akamai-axtest.akamaized.net/routes/lapd-v1-acceptance/www_c4/Manifest.m3u8",
mute: true,
autoPlay: true,
hlsjsConfig: {
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
}
});
p2pml.hlsjs.initClapprPlayer(player);
} else {
document.write("Not supported :(");
}
</script>
</body>
</html>
The library uses window.p2pml.hlsjs
as a root namespace in Web browser for:
Engine
- hls.js support engineinitHlsJsPlayer
- hls.js player integrationinitClapprPlayer
- Clappr player integrationinitFlowplayerHlsJsPlayer
- Flowplayer integrationinitJwPlayer
- JW Player integrationinitMediaElementJsPlayer
- MediaElement.js player integrationinitVideoJsContribHlsJsPlayer
- Video.js player integrationversion
- API versionEngine
hls.js support engine.
Engine.isSupported()
Returns result from p2pml.core.HybridLoader.isSupported()
.
engine = new Engine([settings])
Creates a new Engine
instance.
settings
structure:
segments
forwardSegmentCount
- Number of segments for building up predicted forward segments sequence; used to predownload and share via P2P. Default is 20;loader
HybridLoader
(see P2P Media Loader Core API for details);engine.getSettings()
Returns engine instance settings.
engine.createLoaderClass()
Creates hls.js loader class bound to this engine.
engine.setPlayingSegment(url)
Notifies engine about current playing segment url.
Needed for own integrations with other players. If you write one, you should update engine with current playing segment url from your player.
engine.destroy()
Destroys engine; destroy loader and segment manager.
We support many players, but it is possible to write your own integration in case it is no supported at the moment. Feel free to make pull requests with your player integrations.
In order a player to be able to integrate with the Engine, it should meet following requirements:
hls.js
.hls
configuration. This is needed for us to be able to override hls.js loader
.hls
object, you just call p2pml.hlsjs.initHlsJsPlayer(hls)
;hlsFragChanged
- call engine.setPlayingSegment(url)
to notify Engine about current playing segment url;hlsDestroying
- call engine.destroy()
to inform Engine about destroying hls.js player;initHlsJsPlayer(player)
hls.js player integration.
player
should be valid hls.js instance.
Example
var engine = new p2pml.hlsjs.Engine();
var hls = new Hls({
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
});
p2pml.hlsjs.initHlsJsPlayer(hls);
hls.loadSource("https://example.com/path/to/your/playlist.m3u8");
var video = document.getElementById("video");
hls.attachMedia(video);
initClapprPlayer(player)
Clappr player integration.
player
should be valid Clappr player instance.
Example
var engine = new p2pml.hlsjs.Engine();
var player = new Clappr.Player({
parentId: "#video",
source: "https://example.com/path/to/your/playlist.m3u8",
hlsjsConfig: {
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
}
});
p2pml.hlsjs.initClapprPlayer(player);
initFlowplayerHlsJsPlayer(player)
Flowplayer integration.
player
should be valid Flowplayer instance.
Example
var engine = new p2pml.hlsjs.Engine();
var player = flowplayer("#video", {
clip: {
sources: [{
src: "https://example.com/path/to/your/playlist.m3u8",
type: "application/x-mpegurl"
}]
},
hlsjs: {
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
}
});
p2pml.hlsjs.initFlowplayerHlsJsPlayer(player);
initJwPlayer(player)
JW Player integration.
player
should be valid JW Player instance.
Example
var engine = new p2pml.hlsjs.Engine();
var player = jwplayer("player");
player.setup({
file: "https://example.com/path/to/your/playlist.m3u8"
});
var provider = require("@hola.org/jwplayer-hlsjs");
provider.attach();
p2pml.hlsjs.initJwPlayer(player, {
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
});
initMediaElementJsPlayer(mediaElement)
MediaElement.js player integration.
mediaElement
should be valid value received from success handler of the MediaElementPlayer.
Example
var engine = new p2pml.hlsjs.Engine();
// allow only one supported renderer
mejs.Renderers.order = [ "native_hls" ];
var player = new MediaElementPlayer("video", {
hls: {
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
},
success: function (mediaElement) {
p2pml.hlsjs.initMediaElementJsPlayer(mediaElement);
}
});
player.setSrc("https://example.com/path/to/your/playlist.m3u8");
player.load();
initVideoJsContribHlsJsPlayer(player)
Video.js player integration.
player
should be valid Video.js player instance.
Example
var engine = new p2pml.hlsjs.Engine();
var player = videojs("video", {
html5: {
hlsjsConfig: {
liveSyncDurationCount: 7,
loader: engine.createLoaderClass()
}
}
});
p2pml.hlsjs.initVideoJsContribHlsJsPlayer(player);
player.src({
src: "https://example.com/path/to/your/playlist.m3u8",
type: "application/x-mpegURL"
});
FAQs
P2P Media Loader hls.js integration
We found that p2p-media-loader-hlsjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.