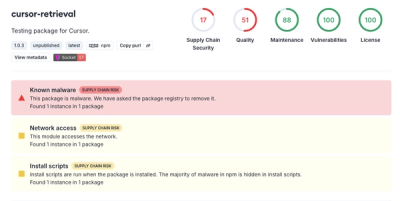
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
padded-grid
Advanced tools
A comprehensive toolkit for maintaining consistent typography and spacing in React applications. Featuring debug overlays for grids and baselines, stack components for vertical rhythm, and spacing utilities that help ensure precise typography and layout d
Padded Grid is a lightweight, debugging / dev-tool library for visualizing and maintaining consistent grid systems in React applications. Inspired by tools like Figma, it provides powerful, customizable grid overlays and spacing utilities to ensure precise alignment and spacing during development.
Install via npm or yarn:
npm install padded-grid
Add padded-grid to your React project to visualize grids and manage spacing during development:
import { XGrid, YGrid, Spacer } from 'padded-grid';
import 'padded-grid/styles.css';
function App() {
const showGrid = process.env.NODE_ENV === 'development';
return (
<div>
{/* Baseline grid for typography alignment */}
<YGrid
config={{
baseUnit: 8,
height: "100%",
}}
visibility={showGrid ? 'visible' : 'hidden'}
/>
{/* Column grid overlay */}
<XGrid
config={{
columns: 12,
gap: 16,
maxWidth: "1200px",
}}
visibility={showGrid ? 'visible' : 'hidden'}
/>
{/* Spacer for dynamic spacing */}
<Spacer
height="16px"
width="100%"
config={{
baseUnit: 8,
color: "#ff0000",
}}
visibility="visible"
/>
<main>Your content...</main>
</div>
);
}
The XGrid component provides column-based grid overlays for layout visualization.
interface XGConfig {
columns?: number | GridColumnsPattern; // Fixed number or pattern of columns
columnWidth?: CSSValue; // For auto-calculated columns
gap?: CSSValue; // Gap between columns
align?: 'start' | 'center' | 'end'; // Grid alignment
color?: CSSProperties['color']; // Guide color
maxWidth?: CSSValue; // Maximum grid width
padding?: CSSProperties['padding']; // Grid padding
variant?: 'line'; // Optional line variant
zIndex?: number; // Z-index for grid
}
interface XGProps {
config: XGConfig;
visibility?: 'hidden' | 'visible';
className?: string;
style?: Partial<XGStyles>;
}
// Fixed columns
<XGrid
config={{
columns: 12,
gap: 16,
maxWidth: "1200px",
}}
visibility="visible"
/>
// Custom column pattern
<XGrid
config={{
columns: ['64px', '1fr', '2fr', '1fr', '64px'],
gap: 24,
color: "#0000ff1a",
}}
visibility="visible"
/>
The YGrid component provides baseline grid overlays for typography alignment.
interface YGConfig {
baseUnit?: number; // Base unit for spacing (default: 8)
height?: CSSValue; // Grid height (default: '100%')
variant?: 'line' | 'flat'; // Grid style variant (default: "line")
color?: CSSProperties['color']; // Guide color
zIndex?: number; // Z-index for layering
}
interface YGProps {
config: YGConfig;
visibility?: 'hidden' | 'visible';
className?: string;
style?: Partial<YGStyles>;
}
// Basic baseline grid
<YGrid
config={{
baseUnit: 8,
height: "100%",
}}
visibility="visible"
/>
// Custom variant and color
<YGrid
config={{
baseUnit: 8,
variant: "flat",
color: "rgba(255,0,0,0.1)",
}}
visibility="visible"
/>
The Spacer component provides a flexible way to add spacing in your layouts. It adjusts its dimensions dynamically and supports optional measurement indicators for development.
Use Spacer to manage dynamic spacing between elements, instead of wrapping your Stacks with useless noisy paddings.
interface SpacerProps {
height?: CSSValue; // Height of the spacer
width?: CSSValue; // Width of the spacer
config?: {
baseUnit?: number; // Base unit for spacing (default: 8)
variant?: 'line'; // Style variant for the spacer
color?: CSSProperties['color']; // Color of the spacer
zIndex?: number; // Z-index for layering
};
indicatorNode?: (value: number, dimension: 'width' | 'height') => ReactNode; // Custom indicator renderer
visibility?: 'hidden' | 'visible'; // Visibility of the spacer
className?: string; // Additional class names
style?: CSSProperties; // Inline styles
}
// Simple spacer
<Spacer
height="16px"
width="100%"
visibility="visible"
/>
// Spacer with custom configuration
<Spacer
height="32px"
config={{
baseUnit: 8,
color: "#ff0000",
}}
visibility="visible"
/>
// Spacer with measurement indicators
<Spacer
height="16px"
indicatorNode={(value, dimension) => (
<div>{`${dimension}: ${value}px`}</div>
)}
visibility="visible"
/>
MIT © François Denavaut
1.1.0
FAQs
A comprehensive toolkit for maintaining consistent typography and spacing in React applications. Featuring debug overlays for grids and baselines, stack components for vertical rhythm, and spacing utilities that help ensure precise typography and layout d
The npm package padded-grid receives a total of 28 weekly downloads. As such, padded-grid popularity was classified as not popular.
We found that padded-grid demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.