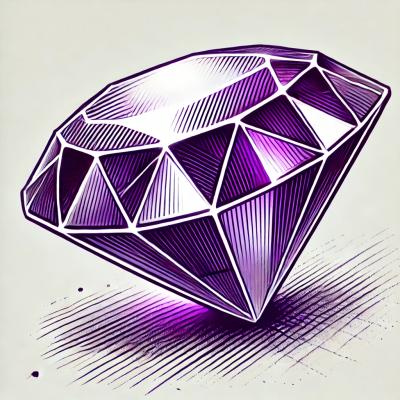
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
paillier-bigint
Advanced tools
An implementation of the Paillier cryptosystem using native JS (stage 3) implementation of BigInt
An implementation of the Paillier cryptosystem relying on the native JS (stage 3) implementation of BigInt. It can be
used by any Web Browser or webview supporting
BigInt
and with Node.js (>=10.4.0). In the latter case, for multi-threaded primality tests (during key generation), you should
use Node.js 11 or enable at runtime with node --experimental-worker
with Node.js >=10.5.0.
The operations supported on BigInts are not constant time. BigInt can be therefore unsuitable for use in cryptography. Many platforms provide native support for cryptography, such as Web Cryptography API or Node.js Crypto.
The Paillier cryptosystem, named after and invented by Pascal Paillier in 1999, is a probabilistic asymmetric algorithm for public key cryptography. A notable feature of the Paillier cryptosystem is its homomorphic properties.
The product of two ciphertexts will decrypt to the sum of their corresponding plaintexts,
D( E(m1) · E(m2) ) mod n^2 = m1 + m2 mod n
The product of a ciphertext with a plaintext raising g will decrypt to the sum of the corresponding plaintexts,
D( E(m1) · g^(m2) ) mod n^2 = m1 + m2 mod n
An encrypted plaintext raised to the power of another plaintext will decrypt to the product of the two plaintexts,
D( E(m1)^(m2) mod n^2 ) = m1 · m2 mod n,
D( E(m2)^(m1) mod n^2 ) = m1 · m2 mod n.
More generally, an encrypted plaintext raised to a constant k will decrypt to the product of the plaintext and the constant,
D( E(m1)^k mod n^2 ) = k · m1 mod n.
However, given the Paillier encryptions of two messages there is no known way to compute an encryption of the product of these messages without knowing the private key.
n
, or keyLength
in bits.p
and q
randomly and independently of each other such that gcd( p·q, (p-1)(q-1) )=1
and n=p·q
has a key length of keyLength. For instance:
p
with a bit length of keyLength/2 + 1
.q
with a bit length of keyLength/2
.n=p·q
is keyLength
.λ = lcm(p-1, q-1)
with lcm(a, b) = a·b / gcd(a, b)
.g
in Z*
of n^2
. g
can be computed as follows (there are other ways):
α
and β
in Z*
of n
.g=( α·n + 1 ) β^n mod n^2
.μ=( L( g^λ mod n^2 ) )^(-1) mod n
where L(x)=(x-1)/n
.The public (encryption) key is (n, g).
The private (decryption) key is (λ, μ).
Let m
in Z*
of n
be the clear-text message,
Select random integer r
in (1, n^2)
.
Compute ciphertext as: c = g^m · r^n mod n^2
Let c
be the ciphertext to decrypt, where c
in (0, n^2)
.
m = L( c^λ mod n^2 ) · μ mod n
paillier-bigint
is distributed for web browsers and/or webviews supporting BigInt as an ES6 module or an IIFE file; and for Node.js (>=10.4.0), as a CJS module.
paillier-bigint
can be imported to your project with npm
:
npm install paillier-bigint
NPM installation defaults to the ES6 module for browsers and the CJS one for Node.js.
For web browsers, you can also directly download the minimised version of the IIFE file or the ES6 module from GitHub.
Every input number should be a string in base 10, an integer, or a bigint. All the output numbers are of type bigint
.
An example with Node.js:
// import paillier
const paillier = require('paillier-bigint.js');
// (asynchronous) creation of a random private, public key pair for the Paillier cryptosystem
const {publicKey, privateKey} = await paillier.generateRandomKeys(3072);
// optionally, you can create your public/private keys from known parameters
const publicKey = new paillier.PublicKey(n, g);
const privateKey = new paillier.PrivateKey(lambda, mu, p, q, publicKey);
// encrypt m
let c = publicKey.encrypt(m);
// decrypt c
let d = privateKey.decrypt(c);
// homomorphic addition of two ciphertexts (encrypted numbers)
let c1 = publicKey.encrypt(m1);
let c2 = publicKey.encrypt(m2);
let encryptedSum = publicKey.addition(c1, c2);
let sum = privateKey.decrypt(encryptedSum); // m1 + m2
// multiplication by k
let c1 = publicKey.encrypt(m1);
let encryptedMul = publicKey.multiply(c1, k);
let mul = privateKey.decrypt(encryptedMul); // k · m1
From a browser, you can just load the module in a html page as:
<script type="module">
import * as paillier from 'paillier-bigint-latest.browser.mod.min.js';
// (asynchronous) creation of a random private, public key pair for the Paillier cryptosystem
paillier.generateRandomKeys(3072).then((keyPair) => {
const publicKey = keyPair.publicKey;
const privateKey = keyPair.privateKey;
// ...
});
// You can also create your public/private keys from known parameters
const publicKey = new paillier.PublicKey(n, g);
const privateKey = new paillier.PrivateKey(lambda, mu, p, q, publicKey);
// encrypt m is just
let c = publicKey.encrypt(m);
// decrypt c
let d = privateKey.decrypt(c);
// homomorphic addition of two ciphertexts (encrypted numbers)
let c1 = publicKey.encrypt(m1);
let c2 = publicKey.encrypt(m2);
let encryptedSum = publicKey.addition(c1, c2);
let sum = privateKey.decrypt(encryptedSum); // m1 + m2
// multiplication by k
let c1 = publicKey.encrypt(m1);
let encryptedMul = publicKey.multiply(c1, k);
let mul = privateKey.decrypt(encryptedMul); // k · m1
</script>
Promise
Generates a pair private, public key for the Paillier cryptosystem in synchronous mode
Class for a Paillier public key
Class for Paillier private keys.
Object
Kind: global class
number
bigint
bigint
bigint
Creates an instance of class PaillierPublicKey
Param | Type | Description |
---|---|---|
n | bigint | stringBase10 | number | the public modulo |
g | bigint | stringBase10 | number | the public generator |
number
Get the bit length of the public modulo
Kind: instance property of PublicKey
Returns: number
- - bit length of the public modulo
bigint
Paillier public-key encryption
Kind: instance method of PublicKey
Returns: bigint
- - the encryption of m with this public key
Param | Type | Description |
---|---|---|
m | bigint | stringBase10 | number | a cleartext number |
bigint
Homomorphic addition
Kind: instance method of PublicKey
Returns: bigint
- - the encryption of (m_1 + ... + m_2) with this public key
Param | Type | Description |
---|---|---|
...ciphertexts | bigint | number | n >= 2 ciphertexts (c_1,..., c_n) that are the encryption of (m_1, ..., m_n) with this public key |
bigint
Pseudo-homomorphic Paillier multiplication
Kind: instance method of PublicKey
Returns: bigint
- - the encryption of k·m with this public key
Param | Type | Description |
---|---|---|
c | bigint | a number m encrypted with this public key |
k | bigint | stringBase10 | number | either a cleartext message (number) or a scalar |
Kind: global class
number
bigint
bigint
Creates an instance of class PaillierPrivateKey
Param | Type | Description |
---|---|---|
lambda | bigint | stringBase10 | number | |
mu | bigint | stringBase10 | number | |
p | bigint | stringBase10 | number | a big prime |
q | bigint | stringBase10 | number | a big prime |
publicKey | PaillierPublicKey |
number
Get the bit length of the public modulo
Kind: instance property of PrivateKey
Returns: number
- - bit length of the public modulo
bigint
Get the public modulo n=p·q
Kind: instance property of PrivateKey
Returns: bigint
- - the public modulo n=p·q
bigint
Paillier private-key decryption
Kind: instance method of PrivateKey
Returns: bigint
- - the decryption of c with this private key
Param | Type | Description |
---|---|---|
c | bigint | stringBase10 | a (big) number encrypted with the public key |
Promise
Generates a pair private, public key for the Paillier cryptosystem in synchronous mode
Kind: global constant
Returns: Promise
- - a promise that resolves to a KeyPair of public, private keys
Param | Type | Description |
---|---|---|
bitLength | number | the bit lenght of the public modulo |
simplevariant | boolean | use the simple variant to compute the generator |
Class for a Paillier public key
Kind: global constant
number
bigint
bigint
bigint
Creates an instance of class PaillierPublicKey
Param | Type | Description |
---|---|---|
n | bigint | stringBase10 | number | the public modulo |
g | bigint | stringBase10 | number | the public generator |
number
Get the bit length of the public modulo
Kind: instance property of PublicKey
Returns: number
- - bit length of the public modulo
bigint
Paillier public-key encryption
Kind: instance method of PublicKey
Returns: bigint
- - the encryption of m with this public key
Param | Type | Description |
---|---|---|
m | bigint | stringBase10 | number | a cleartext number |
bigint
Homomorphic addition
Kind: instance method of PublicKey
Returns: bigint
- - the encryption of (m_1 + ... + m_2) with this public key
Param | Type | Description |
---|---|---|
...ciphertexts | bigint | number | n >= 2 ciphertexts (c_1,..., c_n) that are the encryption of (m_1, ..., m_n) with this public key |
bigint
Pseudo-homomorphic Paillier multiplication
Kind: instance method of PublicKey
Returns: bigint
- - the encryption of k·m with this public key
Param | Type | Description |
---|---|---|
c | bigint | a number m encrypted with this public key |
k | bigint | stringBase10 | number | either a cleartext message (number) or a scalar |
Class for Paillier private keys.
Kind: global constant
number
bigint
bigint
Creates an instance of class PaillierPrivateKey
Param | Type | Description |
---|---|---|
lambda | bigint | stringBase10 | number | |
mu | bigint | stringBase10 | number | |
p | bigint | stringBase10 | number | a big prime |
q | bigint | stringBase10 | number | a big prime |
publicKey | PaillierPublicKey |
number
Get the bit length of the public modulo
Kind: instance property of PrivateKey
Returns: number
- - bit length of the public modulo
bigint
Get the public modulo n=p·q
Kind: instance property of PrivateKey
Returns: bigint
- - the public modulo n=p·q
bigint
Paillier private-key decryption
Kind: instance method of PrivateKey
Returns: bigint
- - the decryption of c with this private key
Param | Type | Description |
---|---|---|
c | bigint | stringBase10 | a (big) number encrypted with the public key |
Object
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
publicKey | PublicKey | a Paillier's public key |
privateKey | PrivateKey | the associated Paillier's private key |
FAQs
An implementation of the Paillier cryptosystem using native JS (ECMA 2020) implementation of BigInt
The npm package paillier-bigint receives a total of 8,830 weekly downloads. As such, paillier-bigint popularity was classified as popular.
We found that paillier-bigint demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.