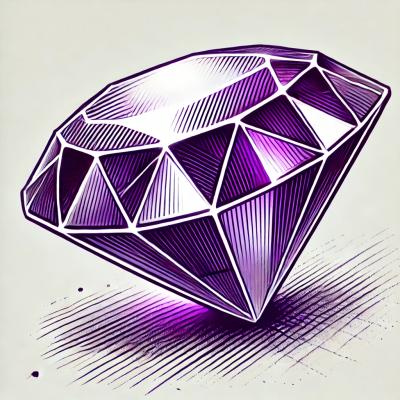
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
A tiny parallax library that updates styles on scroll
Parallax works by listening to every scroll event. Parallaxis is probably the only parallax library that thinks parallax is a shit show.
This leads to Parallaxis being quite opinionated. It allows only a few element styles to be updated on scroll, in the hope of not totally killing performance.
opacity
translateX
translateY
For best performance try to only use Parallaxis on one or two elements per page, and avoid using on mobile completely.
Alternatively, take a look in /examples
.
npm install parallaxis
<h1
class="js-parallaxis"
data-start="0"
data-end="200"
data-opacity-start="1"
data-opacity-end="0"
>
Hello world
</h1>
import parallaxis from 'parallaxis'
parallaxis()
The above example translates to:
window.scrollY
equals 0
then the opacity
of the h1
will be 1
.window.scrollY
equals 200
, or more, then the
opacity
of the h1
will be 0
.window.scrollY
is somewhere between 0
and
200
then the opacity
of the h1
will be
somewhere between 0
and 1
.The parallaxis
function can take an object, that
may include the following properties.
The class name that Parallaxis uses to locate elements.
Defaults to js-parallaxis
.
parallaxis({ className: 'my-special-class' })
Parallaxis uses element data attributes for configuration.
This is the window.scrollY
position that will be the
element's update start point.
<h1
class="js-parallaxis"
data-start="0"
>
Hello world
</h1>
This is the window.scrollY
position that will be the
element's update end point.
<h1
class="js-parallaxis"
data-start="0"
data-end="200"
>
Hello world
</h1>
Defining data-opacity-start
and data-opacity-end
will
result in opacity
style updates.
<h1
class="js-parallaxis"
data-start="0"
data-end="200"
data-opacity-start="1"
data-opacity-end="0"
>
Hello world
</h1>
Defining data-translatex-start
and data-translatex-end
will result in transform: translateX()
style updates.
<h1
class="js-parallaxis"
data-start="0"
data-end="200"
data-translatex-start="0"
data-translatex-end="200"
>
Hello world
</h1>
Defining data-translatey-start
and data-translatey-end
will result in transform: translateY()
style updates.
<h1
class="js-parallaxis"
data-start="0"
data-end="200"
data-translatey-start="0"
data-translatey-end="200"
>
Hello world
</h1>
Parallaxis is packaged with Babel, and
makes use of Array.from
.
If you want Parallaxis to work on browsers that don't support
this method (e.g. IE11), then you will need to
polyfill Array.from
before calling parallaxis
.
FAQs
A tiny parallax library that updates styles on scroll
The npm package parallaxis receives a total of 4 weekly downloads. As such, parallaxis popularity was classified as not popular.
We found that parallaxis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.