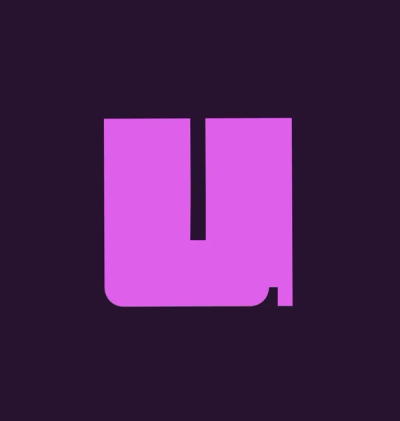
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Parch is a simple RESTful framework combining the power of restify for routing and sequelize ORM for dao access. Stop rewriting your server code and get parched.
** WIP: parch is very much still in beta so use at your own risk **
If you'd like to contribute, take a look at the roadmap
npm install --save parch
For a full list of available options see below
const parch = require("parch");
// define your app
const parch = new parch.Application({
server: {
name: "my-app",
certificate: "/path/to/my.crt",
key: "/path/to/my.key",
middlewares: [
restify.bodyParser(),
restify.queryParser(),
myCustomMiddleware()
]
},
authentication: {
secretKey: "ssshhh",
unauthenticated: [/\/posts[\s\S]*/, "/users/resetPassword"]
},
controllers: {
dir: path.resolve(__dirname, "controllers")
},
database: {
connection: {
username: "postgres",
password: "postgres",
database: "postgres",
host: "localhost",
dialect: "postgres",
logging: true
},
models: {
dir: path.resolve(__dirname, "models")
}
}
});
// wire up your routes
parch.map(function () {
this.resource("user");
this.route("user/resetPassword", {
using: "users:resetPassword", // controller:method
method: "post" // request method
});
});
parch.start(3000).then(() => {
console.log("App listening.")
});
The above will create the following route mapping
POST /users => UserController.create
GET /users => UserController.index
GET /users/:id => UserController.show
PUT /users/:id => UserController.update
DELETE /users/:id => UserController.destroy
POST /users/resetPassword => UserController.resetPassword
const parch = require("parch");
class UserController extends parch.Controller {
constructor(options) {
super(options);
}
index(req, res, next) {
this.findAll(req.query).then(records => {
/**
* {
* users: [{
* ...
* }]
* }
*/
res.send(this.statusCodes.SUCCESS, records);
}).catch(next);
}
show(req, res, next) {
this.findOne(req.params.id).then(record => {
/**
* {
* user: {
* ...
* }
* }
*/
res.send(this.statusCodes.SUCCESS, record);
}).catch(next);
}
create(req, res, next) {
this.createRecord(req.body.user).then(record => {
/**
* {
* user: {
* ...
* }
* }
*/
res.send(this.statusCodes.CREATED, record);
}).catch(next);
}
update(req, res, next) {
this.updateRecord(req.params.id, req.body).then(updatedRecord => {
/**
* {
* user: {
* ...
* }
* }
*/
res.send(this.statusCodes.SUCCESS, record);
}).catch(next);
}
destroy(req, res, next) {
this.destroyRecord(req.params.id).then(() => {
res.send(this.statusCodes.NO_CONTENT);
}).catch(next);
}
resetPassword(req, res, next) {
this.findOne(req.params.id).then(record => {
record.password = req.body.password;
return record.save();
}).then(record => {
res.send(this.statusCodes.SUCCESS);
}).catch(next);
}
}
Models are defined following the sequelize define, pattern.
lib/models/user.js
class UserModel extends parch.Model {
constructor() {
super();
}
define(DataTypes) {
const user = {
email: {
type: DataTypes.STRING,
validate: { isEmail: true }
}
};
return user;
}
}
parch loads associations of a record as an array of ids.
class UserController extends parch.Controller {
constructor() {
super();
}
show(req, res, next) {
this.findOne(req.params.id).then(user => {
/**
* {
* user: {
* firstName: "John",
* posts: [1, 2, 3]
* }
* }
*/
});
}
}
Authentication and authorization is handled using jwt, with more options coming
in the future. To disable auth for specific routes, use the
authentication.unauthenticated
array. Empty by default, you can add an entire controller,
or a controller's method.
const parch = new parch.Application({
authentication: {
unauthenticated: [/\/posts[\s\S]*/, "/users/resetPassword"]
}
});
TODO
TODO
TODO
0.0.5 (2016-06-22)
<a name="0.0.4"></a>
FAQs
Restify + Sequelize
The npm package parch receives a total of 24 weekly downloads. As such, parch popularity was classified as not popular.
We found that parch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.