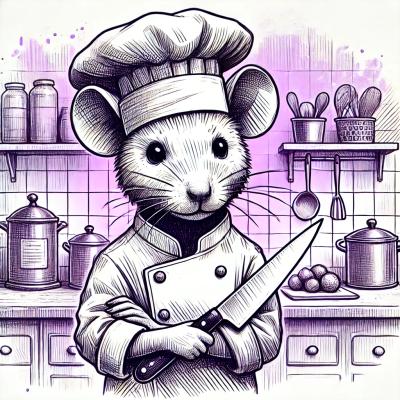
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
parse-github-url
Advanced tools
The parse-github-url npm package is a utility for parsing GitHub repository URLs into their component parts. It helps in extracting information such as the owner, repository name, branch, and file path from a given GitHub URL.
Parse GitHub URL
This feature allows you to parse a GitHub URL and extract its components such as the owner, repository name, and more. The code sample demonstrates how to use the package to parse a GitHub URL and log the parsed components.
const parse = require('parse-github-url');
const parsed = parse('https://github.com/IonicaBizau/parse-github-url');
console.log(parsed);
Extract Owner and Repository
This feature allows you to specifically extract the owner and repository name from a GitHub URL. The code sample shows how to parse the URL and log the owner and repository name.
const parse = require('parse-github-url');
const { owner, name } = parse('https://github.com/IonicaBizau/parse-github-url');
console.log(`Owner: ${owner}, Repository: ${name}`);
Handle Different URL Formats
This feature demonstrates the package's ability to handle different GitHub URL formats, including those with different protocols and extensions. The code sample shows how to parse a URL with the 'git+' protocol and '.git' extension.
const parse = require('parse-github-url');
const parsed = parse('git+https://github.com/IonicaBizau/parse-github-url.git');
console.log(parsed);
The github-url-to-object package converts GitHub URLs to objects containing useful information like owner, name, and branch. It is similar to parse-github-url but also includes additional properties like API URLs and HTTPS URLs.
The parse-repo package parses repository URLs from various hosting services, including GitHub. It provides a more generalized solution compared to parse-github-url, which is specific to GitHub.
The git-url-parse package parses Git URLs and supports multiple Git hosting services, including GitHub, GitLab, and Bitbucket. It offers a broader range of functionality compared to parse-github-url, which is focused solely on GitHub.
Parse a github URL into an object.
Install with npm:
$ npm install parse-github-url --save
HEADS UP! Breaking changes in 0.3.0!!!
See the release history for details.
Why another GitHub URL parser library?
Seems like every lib I've found does too much, like both stringifying and parsing, or converts the URL from one format to another, only returns certain segments of the URL except for what I need, yields inconsistent results or has poor coverage.
var gh = require('parse-github-url');
gh('https://github.com/jonschlinkert/micromatch');
Results in:
{
"owner": "jonschlinkert",
"name": "micromatch",
"repo": "jonschlinkert/micromatch",
"branch": "master"
}
Generated results from test fixtures:
// assemble/verb#1.2.3
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: '1.2.3' }
// assemble/verb#branch
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'branch' }
// assemble/verb
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git+https://github.com/assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git+ssh://github.com/assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git://gh.pages.com/assemble/verb.git
{ host: 'gh.pages.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git://github.assemble.com/assemble/verb.git
{ host: 'github.assemble.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git://github.assemble.two.com/assemble/verb.git
{ host: 'github.assemble.two.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git://github.com/assemble/verb
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git://github.com/assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git@gh.pages.com:assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// git@github.com:assemble/verb.git#1.2.3
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: '1.2.3' }
// git@github.com:assemble/verb.git#v1.2.3
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'v1.2.3' }
// git@github.com:assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// github:assemble/verb
{ host: 'assemble',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// http://github.com/assemble
{ host: 'github.com',
owner: 'assemble',
name: null,
repo: null,
repository: null,
branch: 'master' }
// http://github.com/assemble/verb
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// http://github.com/assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// http://github.com/assemble/verb/tree
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'tree' }
// http://github.com/assemble/verb/tree/master
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// http://github.com/assemble/verb/tree/master/foo/bar
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master/foo/bar' }
// https://assemble.github.com/assemble/verb/somefile.tar.gz
{ host: 'assemble.github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'somefile.tar.gz' }
// https://assemble.github.com/assemble/verb/somefile.zip
{ host: 'assemble.github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'somefile.zip' }
// https://assemble@github.com/assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// https://gh.pages.com/assemble/verb.git
{ host: 'gh.pages.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// https://github.com/assemble/verb
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// https://github.com/assemble/verb.git
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// https://github.com/assemble/verb/blob/1.2.3/README.md
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: '1.2.3' }
// https://github.com/assemble/verb/blob/249b21a86400b38969cee3d5df6d2edf8813c137/README.md
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'blob' }
// https://github.com/assemble/verb/blob/master/assemble/index.js
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'master' }
// https://github.com/assemble/verb/tree/1.2.3
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: '1.2.3' }
// https://github.com/assemble/verb/tree/feature/1.2.3
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'feature/1.2.3' }
// https://github.com/repos/assemble/verb/tarball
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'tarball' }
// https://github.com/repos/assemble/verb/zipball
{ host: 'github.com',
owner: 'assemble',
name: 'verb',
repo: 'assemble/verb',
repository: 'assemble/verb',
branch: 'zipball' }
v0.3.0
To be more consistent with node.js/package.json conventions, the following properties were renamed in v0.3.0
:
repo
is now name
(project name)repopath
is now repository
(project repository)user
is now owner
(project owner or org)You might also be interested in these projects:
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Generate readme and API documentation with verb:
$ npm install verb && npm run docs
Or, if verb is installed globally:
$ verb
Install dev dependencies:
$ npm install -d && npm test
Jon Schlinkert
Copyright © 2016, Jon Schlinkert. Released under the MIT license.
This file was generated by verb, v0.9.0, on April 17, 2016.
FAQs
Parse a github URL into an object.
The npm package parse-github-url receives a total of 595,431 weekly downloads. As such, parse-github-url popularity was classified as popular.
We found that parse-github-url demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.