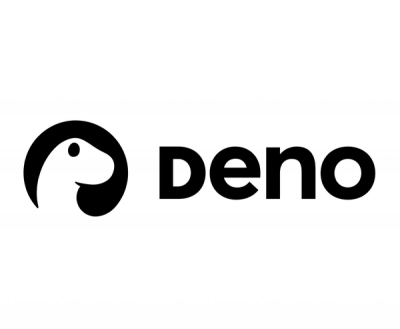
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Generator-based CLI parser.
Install | Synopsis | Usage | Example | Hacking | About
npm install parsec
Parsec is a generator-based CLI options parser written in ES6.
// $ ./app.js --msg=hi
Parsec
.options("msg")
.options("verbose", { default: false })
.parse(process.argv)
{
"message": "hi",
"m": "hi",
"v": true,
"verbose": true
}
Parsec
.options("option string")
.options([aliases], { default: "value" })
.parse(argv, {
sliceIndex: 2,
operandsKey: "_",
noFlags: true,
strictMode: false
})
The first letter of an option string is used as a short alias by default.
// $ ./app.js -tla
Parsec
.options("three")
.options("letter")
.options("abbreviation")
.parse(process.argv)
{
"t": true,
"l": true,
"a": true,
"three": true,
"letter": true,
"abbreviation": true
}
Use an array to specify multiple aliases, and default values via { default: value }
// $ ./app.js -G
Parsec
.options(["G", "g", "great"])
.options(["r", "R"], { default: 8 })
.parse(process.argv)
{
"G":true,
"g":true,
"great":true,
"r":8,
"R":8
}
Options with --no-
prefix before are parsed out of the box.
Use
{ noFlags: false }
to disable this behavior.
// $ ./app.js --no-woman-no-cry --no-wonder=false
Parsec.parse(process.argv)
{
"woman-no-cry": false,
"wonder": true
}
Parsec automatically slices arguments starting from index 2
. To specify a different slice index:
Parsec.parse(["--how", "--nice"], { sliceIndex: 0 })
Parsec.options (aliases, { default: value })
aliases
Use a string to define a single long alias. The first character of the string will be used as the short alias by default.
To specify a custom list of aliases pass an array of strings.
Use the optional, { default: value }
to specify a default value for the option.
Parsec.parse (argv, options)
argv
Array with cli arguments. Usually process.argv
.
options
operandsKey
Key name for array of operands. _ by default.
noFlags
Given an option A, create another option no-A==!A.
Parsec.parse(["--no-love=false", "--no-war", "--no-no", "ok"],
{ sliceIndex: 0 })
{
"love": true,
"war": false,
"no-no": "ok"
}
sliceIndex
Slice index for arguments array.
strictMode
Throw a runtime error if unknown flags are found.
If your app receives the arguments ./app --foo bar -t now -xzy
:
Parsec.parse(process.argv)
will produce the following object
{
"foo": "bar",
"t": "now",
"x": true,
"z": true,
"y": true
}
To customize how the options shall be parsed, use Parsec.options
:
Parsec
.options("foo", { default: "hoge" })
.options("time", { default: 24 })
.options("xoxo")
.options("yoyo")
.options("zorg")
.parse(process.argv)
{
"f": "bar",
"foo": "bar",
"t": "now",
"time": "now",
"x": true,
"xoxo": true,
"z": true,
"zorg": true,
"y": true,
"yoyo": true
}
Why? I wanted a CLI parser with a better feature:bloat ratio and a modern algorithm relying in generators instead of the traditional strategies found in minimist
and nopt
with a simple API to customize options and a small code base.
If Parsec didn't meet your requirements, consider:
git clone https://github.com/bucaran/parsec
cd parsec
npm install
npm run build
MIT © Jorge Bucaran et al :heart:
FAQs
A duration parser and formatter to turn random garbage into program usable times.
The npm package parsec receives a total of 146 weekly downloads. As such, parsec popularity was classified as not popular.
We found that parsec demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.