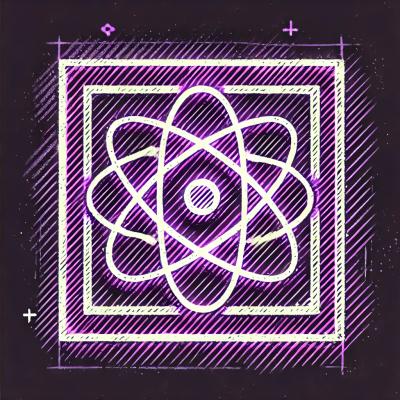
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
payture-official
Advanced tools
This is Offical Payture API for NodeJS. To get started you will need a Merchant account, please contact our support to get one. Here you can explore how to use our API functions! GO!
Simple to install:
npm install payture-official
And include to your project:
var payture = require('payture-official');
You can use one or all of follows API:
Every API function received callback function as second parameter. You need to provide callback for taking required action after receiving a response from the server. The callback accepts 4 parameters:
var callbackFunc = function(error, response, body, responseObject){
// do some work with received arguments....
};
For create instanse of required API you need provide the name of Host and params of your Merchant account (Key and Password).
HOST
Pass the 'https://sandbox.payture.com' for test as the name of Host (first parameter).
Merchant
Params of Merchant account provide as the second parameter for constructor function in simple object, like: { Key : 'YourMerchantAccount', Password : 'YourPassword' };
Please note, that { Key : 'YourMerchantAccount', Password : 'YourPassword' } - fake account, our support help you to get one! Examples you can see below.
For use this you need create instanse of PaytureAPI object. Example:
var api = new payture.Api('https://sandbox.payture.com', { Key : 'YourMerchantAccount', Password : 'YourPassword' });
Please note, that { Key : 'YourMerchantAccount', Password : 'YourPassword' } - fake account, our support help you to get one!
All functions taking 2 parameters: first - required for request data as js object, second - callback function that you need specify. The functions list and examples of usage below. Let's go to explore!
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
PAN : '4111111111111112',
EMonth : 12,
EYear : 20,
CardHolder : 'Vasya Petrov',
SecureCode : 123,
CustomerKey : 'testCustomer',
CustomFields : {},
};
api.pay(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
PAN | Card's number. |
EMonth | The expiry month of card. |
EYear | The expiry year of card. |
CardHolder | Card's holder name. |
SecureCode | CVC2/CVV2. |
CustomerKey | Customer identifier in Payture AntiFraud system. |
CustomFields | Addition fields for processing (especially for AntiFraud system). |
The data and description for params as the same as for pay function.
api.block(data, callbackFunc);
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000
};
api.charge(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
The data and description for params as the same as for charge function.
api.refund(data, callbackFunc);
The data and description for params as the same as for charge function.
api.unblock(data, callbackFunc);
var data = {
Key : 'YourMerchantAccount',
OrderId : 'ORD00000000000000001'
};
api.getState(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
Key | YourMerchantAccount |
OrderId | Payment identifier in your service system. |
For use this API just create instanse of PaytureInPay object:
var inpay = new payture.InPay('https://sandbox.payture.com', { Key : 'YourMerchantAccount', Password : 'YourPassword' });
Please note, that { Key : 'YourMerchantAccount', Password : 'YourPassword' } - fake account, our support help you to get one!
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
IP : '127.0.0.1',
SessionType : 'Pay',
Url : 'payture.com',
TemplateTag : '',
Language : 'RU',
Total : 1,
Product : 'Something'
};
inpay.init(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
IP | Customer's IP. |
SessionType | 'Pay' - for 1-stage payment, 'Block' - for 2-stage payment. |
Url | The url for return customer after completion of payment. |
TemplateTag | Used template. |
Language | Template language. |
var sessionId = 'e5c43d9f-2646-42bc-aeec-0b9005ceb972'; //retrived from init response
inpay.pay(sessionId, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
sessionId | Payment identifier. Given by init response |
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
};
inpay.charge(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
The data and description for params as the same as for charge function.
api.refund(data, callbackFunc);
The data and description for params as the same as for charge function.
api.unblock(data, callbackFunc);
var orderId : 'ORD00000000000000001';
inpay.payStatus(orderId, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
For use this API just create instanse of PaytureEWallet object:
var ew = new payture.EWallet('https://sandbox.payture.com', { Key : 'YourMerchantAccount', Password : 'YourPassword' });
Please note, that { Key : 'YourMerchantAccount', Password : 'YourPassword' } - fake account, our support help you to get one!
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
IP : '127.0.0.1',
SessionType : 'Pay',
CardId : '40252318-de07-4853-b43d-4b67f2cd2077',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
PhoneNumber : '79001234567',
TemplateTag : '',
Language : 'RU',
};
ew.init(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
IP | Customer's IP. |
SessionType | 'Pay' - for 1-stage payment, 'Block' - for 2-stage payment, 'Add' - for register card. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
PhoneNumber | Customer's phone number. |
CardId | Card's identifier. |
TemplateTag | Used template. |
Language | Template language. |
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
IP : '127.0.0.1',
SecureCode : 123,
CardId : '40252318-de07-4853-b43d-4b67f2cd2077',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
};
ew.merchantPayRegCard(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
IP | Customer's IP. |
SecureCode | CVC2/CVV2. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
CardId | Card's identifier. |
ConfirmCode | Confirm code from SMS - if verification code was requested. |
CustomFields | Addition information about customer. |
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
IP : '127.0.0.1',
SecureCode : 123,
CardId : 'FreePay',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
CardHolder : 'Vasya Petrov',
CardNumber : '4111111111111112',
EMonth : 10,
EYear : 20,
};
ew.merchantPayNoRegCard(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
IP | Customer's IP. |
SecureCode | CVC2/CVV2. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
CardNumber | Card's number. |
EMonth | The expiry month of card. |
EYear | The expiry year of card. |
CardHolder | Card's holder name. |
CardId | Card's identifier. |
ConfirmCode | Confirm code from SMS - if verification code was requested. |
CustomFields | Addition information about customer. |
var sessionId = 'e5c43d9f-2646-42bc-aeec-0b9005ceb972';
ew.payturePay(sessionId, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
sessionId | Value extracted from init response (where SessionType=Pay(Block)) |
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
};
ew.charge(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
The data and description for params as the same as for charge function.
ew.refund(data, callbackFunc);
The data and description for params as the same as for charge function.
ew.unblock(data, callbackFunc);
var data = {
OrderId : 'ORD00000000000000001'
};
ew.payStatus(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
var data = {
OrderId : 'ORD00000000000000001',
Amount : 10000,
CardId : '40252318-de07-4853-b43d-4b67f2cd2077',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363'
};
ew.sendCode(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
OrderId | Payment identifier in your service system. |
Amount | Amount of payment kopec. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
CardId | Card's identifier. |
var data = {
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
PhoneNumber : '79156783333'
};
ew.registerCustomer(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
PhoneNumber | Customer's phone number. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
Customer's email. |
var data = {
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
PhoneNumber : '79156783333'
};
ew.deleteCustomer(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
The description for params as the same as for registerCustomer function.
var data = {
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
PhoneNumber : '79156783333'
Email : 'testCustomer@test.com'
};
ew.updateCustomer(data, callbackFunc);
var data = {
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
};
ew.checkCustomer(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
var data = {
SecureCode : 123,
PhoneNumber : '79001234567',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
CardHolder : 'Vasya Petrov',
CardNumber : '4111111111111112',
EMonth : 10,
EYear : 20,
};
ew.merchantAddCard(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
SecureCode | CVC2/CVV2. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
CardNumber | Card's number. |
EMonth | The expiry month of card. |
EYear | The expiry year of card. |
CardHolder | Card's holder name. |
PhoneNumber | Card's identifier. |
var sessionId = 'e5c43d9f-2646-42bc-aeec-0b9005ceb972';
ew.paytureAddCard(sessionId, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
sessionId | Value extracted from init response (where SessionType=Add). |
var data = {
Amount : 101,
CardId : '40252318-de07-4853-b43d-4b67f2cd2077',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
};
ew.activateCard(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
Amount | Amount of payment kopec. |
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
CardId | Card's identifier. |
var data = {
CardId : '40252318-de07-4853-b43d-4b67f2cd2077',
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
};
ew.removeCard(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
CardId | Card's identifier. |
var data = {
VWUserLgn : '123@ya.ru',
VWUserPsw : '2645363',
};
ew.getCardList(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
VWUserLgn | Customer's identifier in Payture system. (Email is recommended). |
VWUserPsw | Customer's password in Payture system. |
For use this API just create instanse of PaytureAppleApi object:
var apple = new payture.ApplePay('https://sandbox.payture.com', { Key : 'YourMerchantAccount', Password : 'YourPassword' });
Please note, that { Key : 'YourMerchantAccount', Password : 'YourPassword' } - fake account, our support help you to get one!
var data = {
PayToken : 'abcdefg',
OrderId : 'ORD00000000000000001',,
};
apple.pay(data, callbackFunc);
Description of provided params.
Parameter's name | Definition |
---|---|
PayToken | PaymentData from PayToken for current transaction |
OrderId | Payment identifier in your service system |
The data and description for params as the same as for pay function.
apple.block(data, callbackFunc);
Visit our site for more information. You can find our contact here.
FAQs
Official Payture API for NodeJS
The npm package payture-official receives a total of 7 weekly downloads. As such, payture-official popularity was classified as not popular.
We found that payture-official demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.