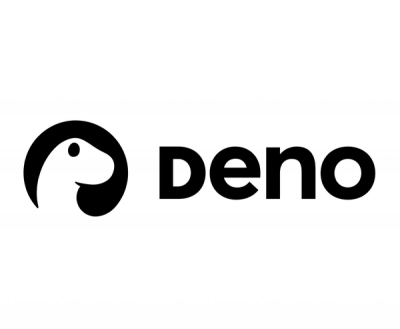
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Lightweight WebRTC browser library that supports video, audio and data channels
Lightweight WebRTC browser library that supports video, audio and data channels.
yarn add peer-lite
Example of two peers connecting to each other locally, see more examples here.
import Peer from 'peer-lite';
const peer1 = new Peer();
const peer2 = new Peer();
peer1.on('signal', async (description) => {
await peer2.signal(description);
})
peer2.on('signal', async (description) => {
await peer1.signal(description);
})
peer1.on('onicecandidates', async (candidates) => {
const promises = candidates.map(async candidate => peer2.addIceCandidate(candidate));
await Promise.all(promises);
});
peer2.on('onicecandidates', async (candidates) => {
const promises = candidates.map(async candidate => peer1.addIceCandidate(candidate));
await Promise.all(promises);
});
peer1.on('streamRemote', (stream) => {
document.querySelector('#video1').srcObject = stream;
});
peer2.on('streamRemote', (stream) => {
document.querySelector('#video2').srcObject = stream;
});
(async () => {
const stream = await Peer.getUserMedia();
peer1.addStream(stream);
peer2.addStream(stream);
peer1.start();
})();
new Peer(Options)
Typescript Options Definition
export interface PeerOptions {
batchCandidates?: boolean;
batchCandidatesTimeout?: number;
enableDataChannels?: boolean;
name?: string;
config?: RTCConfiguration;
constraints?: MediaStreamConstraints;
offerOptions?: RTCOfferOptions;
answerOptions?: RTCAnswerOptions;
channelName?: string;
channelOptions?: RTCDataChannelInit;
sdpTransform?: (sdp: string) => string;
}
The tests run inside a headless Chrome with Playwright using @playwright/test with Jest. These run quickly and allow testing of real WebRTC APIs in a real browser.
yarn test
FAQs
Lightweight WebRTC browser library that supports video, audio and data channels
The npm package peer-lite receives a total of 159 weekly downloads. As such, peer-lite popularity was classified as not popular.
We found that peer-lite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.