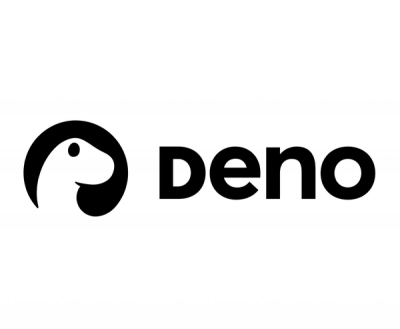
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Better control of Promises
Pendings is a wrapping library over Promises providing flexible control of promise lifecycle.
It is useful for event-based code where you need to manually store resolve
/ reject
callbacks for later fulfillment.
It reduces boilerplate code and allows to split business logic from promise manipulation.
npm install pendings --save
resolve
/ reject
callbacks for later fulfillmenttimeout
waitAll()
methodTypical situation with promises in event-based code:
class Foo {
constructor() {
this.promise = null;
this.resolve = null;
this.reject = null;
}
asyncRequest() {
if (this.promise) { // if promise already exists - return it
return this.promise;
}
this.promise = new Promise((resolve, reject) => {
this.resolve = resolve;
this.reject = reject;
this.send();
});
return this.promise;
}
onSuccess(data) {
this.resolve(data);
}
}
Pending class allows to do it simpler:
const Pending = require('pendings').Pending;
class Foo {
constructor() {
this.pending = new Pending();
}
asyncRequest() {
return this.pending.call(() => this.send());
}
onSuccess(data) {
this.pending.resolve(data);
}
}
Pendings class is useful for dynamic list of promises.
Each promise gets unique id
(manually or auto-generated) and can be resolved later by that id.
const Pendings = require('pendings');
class Foo {
constructor() {
this.pendings = new Pendings();
}
asyncRequest() {
return this.pendings.add(id => {
this.send({id, foo: 'bar'}); // mark request with unique generated `id`
});
}
onSuccess(data) {
this.pendings.resolve(data.id, data); // resolve by `id`
}
onError(data) {
this.pendings.reject(data.id, data); // reject by `id`
}
}
Kind: global class
Promise
*
Boolean
Boolean
Boolean
Boolean
Promise
Creates instance of single pending promise. It holds resolve / reject
callbacks for future fulfillment.
Param | Type | Default | Description |
---|---|---|---|
[options] | Object | ||
[options.timeout] | Number | 0 | |
[options.autoReset] | String | 'never' | automatically reset pending to initial state. Possible values are: never , fufilled , resolved , rejected . |
Promise
Returns promise itself.
Kind: instance property of Pending
*
Returns value with that promise was fulfilled (resolved or rejected).
Kind: instance property of Pending
Boolean
Returns true if promise resolved.
Kind: instance property of Pending
Boolean
Returns true if promise rejected.
Kind: instance property of Pending
Boolean
Returns true if promise fulfilled (resolved or rejected).
Kind: instance property of Pending
Boolean
Returns true if promise is pending.
Kind: instance property of Pending
Callback called when promise is fulfilled (resolved or rejected).
Kind: instance property of Pending
Param | Type |
---|---|
fn | function |
Promise
For the first time this method calls fn
and returns new promise. Also holds resolve
/ reject
callbacks
to allow fulfill promise via pending.resolve()
and pending.reject()
. All subsequent calls of .call(fn)
will return the same promise, which can be still pending or already fulfilled.
To reset this behavior use .reset()
. If options.timeout
is specified, the promise will be automatically
rejected after timeout
milliseconds with TimeoutError
.
Kind: instance method of Pending
Param | Type | Default | Description |
---|---|---|---|
fn | function | ||
[options] | Object | ||
[options.timeout] | Number | 0 | timeout after which promise will be automatically rejected |
Resolves pending promise with specified value
.
Kind: instance method of Pending
Param | Type |
---|---|
[value] | * |
Rejects pending promise with specified value
.
Kind: instance method of Pending
Param | Type |
---|---|
[value] | * |
Helper method: rejects if rejectValue
is truthy, otherwise resolves with resolveValue
.
Kind: instance method of Pending
Param | Type |
---|---|
[resolveValue] | * |
[rejectValue] | * |
Resets to initial state.
Kind: instance method of Pending
Param | Type | Description |
---|---|---|
[error] | Error | custom rejection error if promise is in pending state. |
Kind: global class
Number
Promise
Promise
Boolean
Promise
String
Manipulation of list of promises.
Param | Type | Default | Description |
---|---|---|---|
[options] | Object | ||
[options.autoRemove] | Number | false | automatically remove fulfilled promises from list |
[options.timeout] | Number | 0 | default timeout for all promises |
[options.idPrefix] | String | '' | prefix for generated promise IDs |
Number
Returns count of pending / fulfilled promises in the list.
Kind: instance property of Pendings
Promise
Calls fn
and returns new promise. fn
gets generated unique id
as parameter.
Kind: instance method of Pendings
Param | Type | Description |
---|---|---|
fn | function | |
[options] | Object | |
[options.timeout] | Number | custom timeout for particular promise |
Promise
Calls fn
and returns new promise with specified id
.
If promise with such id
already pending - it will be returned.
Kind: instance method of Pendings
Param | Type | Default | Description |
---|---|---|---|
id | String | ||
fn | function | ||
[options] | Object | ||
[options.timeout] | Number | 0 | custom timeout for particular promise |
Boolean
Checks if promise with specified id
is exists in the list.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
Resolves pending promise by id
with specified value
.
Throws if promise does not exist or is already fulfilled.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
[value] | * |
Rejects pending promise by id
with specified value
.
Throws if promise does not exist or is already fulfilled.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
[value] | * |
Rejects pending promise by id
if reason
is truthy, otherwise resolves with value
.
Throws if promise does not exist or is already fulfilled.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
[resolveValue] | * |
[rejectValue] | * |
Resolves pending promise by id
with specified value
if it exists.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
[value] | * |
Rejects pending promise by id
with specified value
if it exists.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
[value] | * |
Rejects pending promise by id
if reason
is truthy, otherwise resolves with value
.
Kind: instance method of Pendings
Param | Type |
---|---|
id | String |
[resolveValue] | * |
[rejectValue] | * |
Rejects all pending promises with specified value
. Useful for cleanup.
Kind: instance method of Pendings
Param | Type |
---|---|
[value] | * |
Promise
Waits for all promises to fulfill and returns object with resolved/rejected values.
Kind: instance method of Pendings
Returns: Promise
- promise resolved with object {resolved: {id: value, ...}, rejected: {id: value, ...}}
Removes all items from list. If there is waitAll promise - it will be resolved with empty results.
Kind: instance method of Pendings
String
Generates unique ID. Can be overwritten.
Kind: instance method of Pendings
Timeout error for pending promise.
Param | Type |
---|---|
timeout | Number |
MIT @ Vitaliy Potapov
FAQs
Better control of promises
The npm package pendings receives a total of 4 weekly downloads. As such, pendings popularity was classified as not popular.
We found that pendings demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.