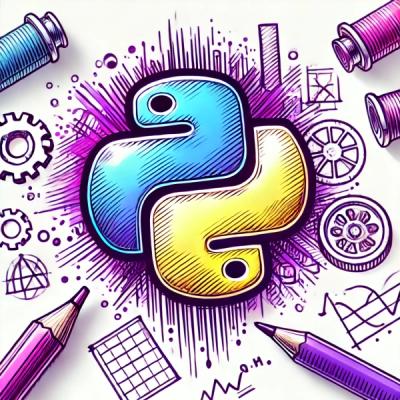
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
peopledatalabs
Advanced tools
A tiny, universal JS client for the People Data Labs API.
This is a simple JavaScript client library to access the various API endpoints provided by People Data Labs.
This library bundles up PDL API requests into simple function calls, making it easy to integrate into your projects. You can use the various API endpoints to access up-to-date, real-world data from our massive Person and Company Datasets.
yarn add peopledatalabs
or
npm i peopledatalabs
First, create the PDLJS client:
import PDLJS from 'peopledatalabs';
const PDLJSClient = new PDLJS({apiKey: "YOUR API KEY"})
Then, send requests to any PDL API Endpoint:
Getting Person Data
// By Enrichment
PDLJSClient.person.enrichment({ phone: '4155688415' }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
// By Search (SQL)
const sqlQuery = "SELECT * FROM person WHERE location_country='mexico' AND job_title_role='health'AND phone_numbers IS NOT NULL;"
PDLJSClient.person.search.sql({ searchQuery: sqlQuery, size: 10 }).then((data) => {
console.log(data['total']);
}).catch((error) => {
console.log(error);
});
// By Search (Elasticsearch)
const esQuery = {
query: {
bool: {
must:[
{term: {location_country: "mexico"}},
{term: {job_title_role: "health"}},
{exists: {field: "phone_numbers"}}
]
}
}
}
PDLJSClient.person.search.elastic({ searchQuery: esQuery, size: 10 }).then((data) => {
console.log(data['total']);
}).catch((error) => {
console.log(error);
});
// By PDL_ID
PDLJSClient.person.retrieve('qEnOZ5Oh0poWnQ1luFBfVw_0000').then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
// By Fuzzy Enrichment
PDLJSClient.person.identify({ name: 'sean thorne' }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
Getting Company Data
// By Enrichment
PDLJSClient.company.enrichment({ website: 'peopledatalabs.com' }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
// By Search (SQL)
const sqlQuery = "SELECT * FROM company WHERE tags='big data' AND industry='financial services' AND location.country='united states';"
PDLJSClient.company.search.sql({ searchQuery: sqlQuery, size: 10 }).then((data) => {
console.log(data['total']);
}).catch((error) => {
console.log(error);
});
// By Search (Elasticsearch)
const esQuery = {
query: {
bool: {
must:[
{term: {tags: "big data"}},
{term: {industry: "financial services"}},
{term: {location_country: "united states"}}
]
}
}
}
PDLJSClient.company.search.elastic({ searchQuery: esQuery, size: 10 }).then((data) => {
console.log(data['total']);
}).catch((error) => {
console.log(error);
});
Using Supporting APIs
// Get Autocomplete Suggestions
PDLJSClient.autocomplete({ field: 'title', text: 'full', size: 10 }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
// Clean Raw Company Strings
PDLJSClient.company.cleaner({ name: 'peOple DaTa LabS' }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
// Clean Raw Location Strings
PDLJSClient.location.cleaner({ location: '455 Market Street, San Francisco, California 94105, US' }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
// Clean Raw School Strings
PDLJSClient.school.cleaner({ name: 'university of oregon' }).then((data) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
Person Endpoints
API Endpoint | PDLJS Function |
---|---|
Person Enrichment API | PDLJS.person.enrichment(...params) |
Person Search API | SQL: PDLJS.person.search.sql(...params) Elasticsearch: PDLJS.person.search.elastic(...params) |
Person Retrieve API | PDLJS.person.retrieve(...params) |
Person Identify API | PDLJS.person.identify(...params) |
Company Endpoints
API Endpoint | PDLJS Function |
---|---|
Company Enrichment API | PDLJS.company.enrichment(...params) |
Company Search API | SQL: PDLJS.company.search.sql(...params) Elasticsearch: PDLJS.company.search.elastic(...params) |
Supporting Endpoints
API Endpoint | PDLJS Function |
---|---|
Autocomplete API | PDLJS.autocomplete(...params) |
Company Cleaner API | PDLJS.company.cleaner(...params) |
Location Cleaner API | PDLJS.location.cleaner(...params) |
School Cleaner API | PDLJS.school.cleaner(...params) |
All of our API endpoints are documented at: https://docs.peopledatalabs.com/
These docs describe the supported input parameters, output responses and also provide additional technical context.
As illustrated in the Endpoints section above, each of our API endpoints is mapped to a specific method in the PDLJS class. For each of these class methods, all function inputs are mapped as input parameters to the respective API endpoint, meaning that you can use the API documentation linked above to determine the input parameters for each endpoint.
As an example:
The following is valid because name
is a supported input parameter to the Person Identify API:
PDLJS.person.identify({ name: 'sean thorne' })
Conversely, this would be invalid because fake_parameter
is not an input parameter to the Person Identify API:
PDLJS.person.identify({ fake_parameter: 'anything' })
Our Person Search API and Company Search API endpoints both support two types of query syntax: you can construct search queries using either SQL
or Elasticsearch
syntax.
In the PDLJS class, the person and company search functions are broken out into two syntax-specific methods as follows:
Data Type | Search Query Syntax | Function |
---|---|---|
Person | SQL | PDLJS.person.search.sql(...params) |
Person | Elasticsearch | PDLJS.person.search.elastic(...params) |
Company | SQL | PDLJS.company.search.sql(...params) |
Company | Elasticsearch | PDLJS.company.search.elastic(...params) |
You can pass your query to these methods using the special searchQuery
function argument, as shown in the following example:
const sqlQuery = "SELECT * FROM company WHERE website='peopledatalabs.com';"
PDLJSClient.company.search.sql({ searchQuery: sqlQuery, size: 10 }).then((data) => {
console.log(data['total']);
}).catch((error) => {
console.log(error);
});
FAQs
JavaScript client with TypeScript support for the People Data Labs API
The npm package peopledatalabs receives a total of 2,107 weekly downloads. As such, peopledatalabs popularity was classified as popular.
We found that peopledatalabs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.