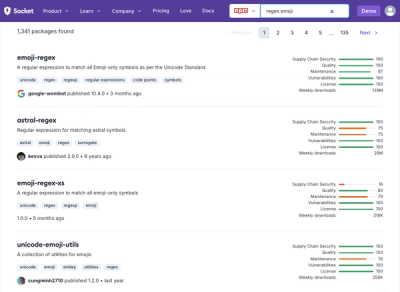
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
The pidusage npm package is a cross-platform process and system monitoring library. It provides statistics such as CPU usage, memory usage, running time, and more for a given process ID (PID). It's particularly useful for monitoring the resource consumption of processes in Node.js applications.
Process Statistics
This feature allows you to monitor the resource usage of a specific process. The code sample demonstrates how to get statistics such as CPU and memory usage for the current process.
const pidusage = require('pidusage');
pidusage(process.pid, function (err, stats) {
console.log(stats);
});
Monitoring Multiple PIDs
Pidusage also supports monitoring multiple processes at once. This code sample shows how to get statistics for multiple PIDs simultaneously.
const pidusage = require('pidusage');
pidusage([1234, 5678], function (err, stats) {
console.log(stats);
});
A library for looking up processes running on the system. While ps-node focuses more on finding and interacting with processes, pidusage specializes in monitoring their resource usage.
This package provides a broad set of system and OS metrics, including process monitoring. Compared to pidusage, systeminformation offers a wider range of system-related information but might be more complex to use for simple process monitoring tasks.
Cross-platform process cpu % and memory usage of a PID.
Ideas from https://github.com/arunoda/node-usage but with no C-bindings.
Please note that if you need to check a Node.JS script process cpu and memory usage, you can use process.cpuUsage
and process.memoryUsage
since node v6.1.0. This script remain useful when you have no control over the remote script, or if the process is not a Node.JS process.
var pidusage = require('pidusage')
pidusage(process.pid, function (err, stats) {
console.log(stats)
// => {
// cpu: 10.0, // percentage (from 0 to 100*vcore)
// memory: 357306368, // bytes
// ppid: 312, // PPID
// pid: 727, // PID
// ctime: 867000, // ms user + system time
// elapsed: 6650000, // ms since the start of the process
// timestamp: 864000000 // ms since epoch
// }
cb()
})
// It supports also multiple pids
pidusage([727, 1234], function (err, stats) {
console.log(stats)
// => {
// 727: {
// cpu: 10.0, // percentage (from 0 to 100*vcore)
// memory: 357306368, // bytes
// ppid: 312, // PPID
// pid: 727, // PID
// ctime: 867000, // ms user + system time
// elapsed: 6650000, // ms since the start of the process
// timestamp: 864000000 // ms since epoch
// },
// 1234: {
// cpu: 0.1, // percentage (from 0 to 100*vcore)
// memory: 3846144, // bytes
// ppid: 727, // PPID
// pid: 1234, // PID
// ctime: 0, // ms user + system time
// elapsed: 20000, // ms since the start of the process
// timestamp: 864000000 // ms since epoch
// }
// }
})
// If no callback is given it returns a promise instead
const stats = await pidusage(process.pid)
console.log(stats)
// => {
// cpu: 10.0, // percentage (from 0 to 100*vcore)
// memory: 357306368, // bytes
// ppid: 312, // PPID
// pid: 727, // PID
// ctime: 867000, // ms user + system time
// elapsed: 6650000, // ms since the start of the process
// timestamp: 864000000 // ms since epoch
// }
// Avoid using setInterval as they could overlap with asynchronous processing
function compute(cb) {
pidusage(process.pid, function (err, stats) {
console.log(stats)
// => {
// cpu: 10.0, // percentage (from 0 to 100*vcore)
// memory: 357306368, // bytes
// ppid: 312, // PPID
// pid: 727, // PID
// ctime: 867000, // ms user + system time
// elapsed: 6650000, // ms since the start of the process
// timestamp: 864000000 // ms since epoch
// }
cb()
})
}
function interval(time) {
setTimeout(function() {
compute(function() {
interval(time)
})
}, time)
}
// Compute statistics every second:
interval(1000)
// Above example using async/await
const compute = async () => {
const stats = await pidusage(process.pid)
// do something
}
// Compute statistics every second:
const interval = async (time) => {
setTimeout(async () => {
await compute()
interval(time)
}, time)
}
interval(1000)
Property | Linux | FreeBSD | NetBSD | SunOS | macOS | Win | AIX | Alpine |
---|---|---|---|---|---|---|---|---|
cpu | ✅ | ❓ | ❓ | ❓ | ✅ | ℹ️ | ❓ | ✅ |
memory | ✅ | ❓ | ❓ | ❓ | ✅ | ✅ | ❓ | ✅ |
pid | ✅ | ❓ | ❓ | ❓ | ✅ | ✅ | ❓ | ✅ |
ctime | ✅ | ❓ | ❓ | ❓ | ✅ | ✅ | ❓ | ✅ |
elapsed | ✅ | ❓ | ❓ | ❓ | ✅ | ✅ | ❓ | ✅ |
timestamp | ✅ | ❓ | ❓ | ❓ | ✅ | ✅ | ❓ | ✅ |
✅ = Working ℹ️ = Not Accurate ❓ = Should Work ❌ = Not Working
Please if your platform is not supported or if you have reported wrong readings file an issue.
By default, pidusage will use procfile
parsing on most unix systems. If you want to use ps
instead use the usePs
option:
pidusage(pid, {usePs: true})
[Promise.<Object>]
Get pid informations.
Kind: global function
Returns: Promise.<Object>
- Only when the callback is not provided.
Access: public
Param | Type | Description |
---|---|---|
pids | Number | Array.<Number> | String | Array.<String> | A pid or a list of pids. |
[options] | object | Options object. See the table below. |
[callback] | function | Called when the statistics are ready. If not provided a promise is returned instead. |
Setting the options programatically will override environment variables
Param | Type | Environment variable | Default | Description |
---|---|---|---|---|
[usePs] | boolean | PIDUSAGE_USE_PS | false | When true uses ps instead of proc files to fetch process information |
[maxage] | number | PIDUSAGE_MAXAGE | 60000 | Max age of a process on history. |
PIDUSAGE_SILENT=1
can be used to remove every console message triggered by pidusage.
If needed this function can be used to delete all in-memory metrics and clear the event loop. This is not necessary before exiting as the interval we're registring does not hold up the event loop.
See also the list of contributors who participated in this project.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Cross-platform process cpu % and memory usage of a PID
We found that pidusage demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.