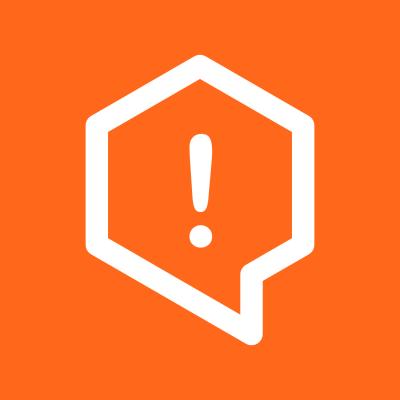
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
plivo-browser-sdk
Advanced tools
A pure javascript webRTC-SIP library
npm install
npm start
This builds the SDK file and attaches it to the port 9000 - http://localhost:9000/plivowebsdk.js
This uses webpack-dev-server so, when there is a change in any file, the build file is auto build, When this is used in plivo-websdk-2.0-example, the app will auto reload with the build with latest changes
npm run build
Minified, production-ready build is created at dist/plivowebsdk.min.js
npm run build:nominfy
A non-minified version of the build file is created dist/plivowebsdk.js
Follow this Git branching Strategy
Use your Plivo's google account to view that document.
To deploy to production (CDN77 and CloudFront), just push to master, the upload is handled by CircleCI.
git checkout master
git pull origin master
git reset --hard <last-stable-tag>
replace <last-stable-tag>
with last stable release tag
git push -f origin master
this force pushes the tag to master and the CI will kick in and deploy the last stable release to production.
Refer example app
Add the javascript file to the example app or to the application that you are build to use the SDK.
To use the development build:
<script type="text/javascript" src="http://localhost:9000/plivowebsdk.js"></script>
To use the production build:
<script type="text/javascript" src="https://cdn.plivo.com/sdk/browser/v2/plivo.min.js"></script>
You may specify a set of configuration parameters to initialise the object. The details are covered in the [configuration section]
var options = {"debug":"DEBUG","permOnClick":true,"codecs":["OPUS","PCMU"],"enableIPV6":false,"audioConstraints":{"optional":[{"googAutoGainControl":false}]},"enableTracking":false,"appId":null};
var plivoWebSdk = new window.Plivo(options);
Pass function references to the events produced from the sdk. This is where your UI manipulation should handle all the different call flows.
plivoWebSdk.client.on('onWebrtcNotSupported', onWebrtcNotSupported);
plivoWebSdk.client.on('onLogin', onLogin);
plivoWebSdk.client.on('onLogout', onLogout);
plivoWebSdk.client.on('onLoginFailed', onLoginFailed);
plivoWebSdk.client.on('onCallRemoteRinging', onCallRemoteRinging);
plivoWebSdk.client.on('onIncomingCallCanceled', onIncomingCallCanceled);
plivoWebSdk.client.on('onCallFailed', onCallFailed);
plivoWebSdk.client.on('onCallAnswered', onCallAnswered);
plivoWebSdk.client.on('onCallTerminated', onCallTerminated);
plivoWebSdk.client.on('onCalling', onCalling);
plivoWebSdk.client.on('onIncomingCall', onIncomingCall);
plivoWebSdk.client.on('onMediaPermission', onMediaPermission);
plivoWebSdk.client.on('mediaMetrics',mediaMetrics);
plivoWebSdk.client.on('audioDeviceChange',audioDeviceChange);
plivoWebSdk.client.on('onConnectionChange',onConnectionChange);
Register using your Plivo Endpoints credentials
var username = “johndoe1234@phone.plivo.com”;
var password = “XXXXXXXX”;
plivoWebSdk.client.login(username, password);
Making a call is among the various methods exposed by the Plivo JS Object. The other methods are described under [Methods]. You can pass extra headers to be sent with the call, but is optional.
var dest = “<jane1234@phone.plivo.com>”;
var extraHeaders = {'X-PH-Test1': 'test1', 'X-PH-Test2': 'test2'};
plivoWebSdk.client.call(dest, extraHeaders);
This is how an incoming call is answered, which is notified by the event onIncomingCall
.
plivoWebSdk.client.answer();
This is how DTMF is sent during a call.
plivoWebSdk.client.sendDtmf('1');
All of these options are optional, if not present their default value will be used.
Option | default | Description |
---|---|---|
debug | DEBUG | Set logging level for sdk, valid values: OFF, ERROR, WARN, INFO, DEBUG, ALL |
permOnClick | false | Set to true if you want to ask for mic permission before call. Otherwise it will be asked on page load |
enableIPV6 | false | Enables or disables IPv6 candidate collection. *Experimental |
codecs | ["OPUS","PCMU"] | Valid array values include OPUS or PCMU . Setting one or the other will force that codec and remove the other. If no codecs is provided it will default to OPUS only |
audioConstraints | {} | Audio constraints object that will be passed to webRTC getUserMedia() . This is a browsers specific audio controls. |
enableTracking | false | set true to collect call stats |
appId | null | if enableTracking is true set a valid callstats.io application id . If you don't have an appId and still wanna track call stats, Just set enableTracking: true and we'll use our default account. |
dscp | false | Buy setting it to true you can enable QoS in voice traffic. Differentiated Services field in the packet headers for all WebRTC traffic. |
registrationDomainSocket | null | if you want to register with specific Plivo websocket server. |
preDetectOwa | false | set it to true if you want to detect one way audio before answering/sending the call. |
These are all the methods supported on the plivoWebSdk.client
Option | params | Description |
---|---|---|
login | username , password | Registering a Plivo endpoint |
logout | Logging out from the registered endpoint | |
call | number , extraHeaders | Call a number or sip address. |
answer | Answer an incoming call | |
hangup | Hangup an ongoing call | |
reject | Reject and incoming call | |
sendDtmf | digit | Send the digits as dtmf |
mute | Mute the mic | |
unmute | Unmute the mic | |
setRingTone | url to audio file | Set the ring tone that plays when calling, default tone is here |
setRingToneBack | url to audio file | Set the ring tone that plays when being called, default tone is here |
setConnectTone | true/false | Dial beep will play till we get 18X response from server. setting false will not play beep tone. |
setDtmfTone | digit , url to audio tone | Set the tone played when sending dtmf. Default tone is located here, where you need to replace digit between dtmf-[digit].mp3 . |
setDebug | INFO | will look for any of [INFO, DEBUG, ERROR, WARN, ALL, OFF] Set the log level of the sdk, DEBUG and ALL will show all logs, OFF will turn off all plivo logs |
sendQualityFeedback | (callUUID ,score , comment ) | score is between 1-5. For score 1-4, comment must be one of the following values ['bad-audio', 'call-dropped', 'wrong-callerid', 'post-dial-delay', 'dtmf-not-captured', 'audio-latency', 'unsolicited-call', 'one-way-audio', 'no-audio', 'never-connected'], only valid if call stats integration setup. If invalid score/comment returns error |
getCallUUID | Returns call UUID if a call is active, else returns null | |
getLastCallUUID | Returns last call UUID, Useful in the cases if you want to send feedback for last call | |
webRTC | Return true if webRTC is supported. false if there is no webrtc support | |
version | It is a variable that returns current version of Plivo SDK |
Methods allowed in audio API plivoWebSdk.client.audio
Name | Param | Description |
---|---|---|
availableDevices {Function} | filter {String} - Optional | Promise based callback! Pass 'input' as filter by Input audio devices,'output' as filter by Output audio devices, null to get all audio devices |
revealAudioDevices {Function} | arg {String} - Optional | Promise based callback! This will force and ask for allow permission, On permisson allowed it will list available devices. Pass 'returnStream' as arg to get local stream in Promise success |
microphoneDevices {Object} | set(deviceId) - deviceId {String} is Manditory, get() ,reset() | set method will set the audioDevice ID as default Microphone device. get method will return the Microphone device ID which is set already!, reset method will remove any Microphone device ID which is already set! |
speakerDevices {Object} | set(deviceId) - deviceId {String} is Manditory, get() ,reset() ,media(source) - source {String} is Manditory | set method will set the audioDevice ID as default Speaker device for DTMF and Callee's/Remote audio. get method will return the Speaker device ID which is set already!, reset method will remove any Speaker device ID which is already set! media will take 'dtmf' or 'ringback' as source name and will return a HTML audio element for the source name you passed |
ringtoneDevices {Object} | set(deviceId) - deviceId {String} is Manditory, get() ,reset() ,media() | set method will set the audioDevice ID as default Ringtone device for incoming ringtone audio. get method will return the Ringtone device ID which is set already!, reset method will remove any Ringtone device ID which is already set! media method will return Ringtone HTML audio element. |
Event | Description |
---|---|
onLogin | Occurs when a login is successful |
onLoginFailed | Occurs when a login has failed |
onLogout | Occurs when a logout is successful |
onCalling | Occurs when a call is initiated |
onCallRemoteRinging | Occurs when the remote end starts ringing during an outbound call |
onCallAnswered | Occurs when the an outbound call is answered |
onCallTerminated | Occurs when the an outbound call has ended |
onIncomingCall | Occurs when there is an incoming call |
onIncomingCallCanceled | Occurs when an incoming call is cancelled by the caller |
onCallFailed(cause) | Occurs when an outbound call fails |
onMediaPermission | Occurs when media permission has been granted |
onWebrtcNotSupported | Occurs when browser does not support web rtc |
audioDeviceChange(deviceObj) | Occurs when there is a change in USB audio device, Device added or removed |
onConnectionChange | Occurs when the connection state is changed such as websocket abruptly closes |
mediaMetrics | Works only for Chrome Occurs with below type high_jitter When the jitter is higher than 30 ms for 3 out of last 5 samples, high_latency When the RTT is higher than 400 ms for 3 out of last 5 samples, high_packetloss When the packet loss is > 10% for OPUS and loss > 20% PCMU, low_mos When sampled mos is < 3 for 3 out of last 5 samples, no_microphone_access When we detect one way audio (<80 bytes sent in 3 seconds), no_audio_received When the user is not able to hear the callee or When audio level is stable for last 3 samples. All the above will have level :warning its group : network/audio related params will have its own value and state active :true/false , ice_timeout will get triggered when ICE gathering takes more than 2 sec either for outgoing call invite or incoming call answer. Example => {active : true, desc : "local_audio", group : "audio", level : "warning", type : "no_audio_received", value : 0} |
These are just behavioural in nature which just tests if a call flows as it should. The SDK code is not unit testable as it has no modularity(all the functions are defined in the callbacks itself).
test/spec.js
Run npm test
in the root directory to start executing tests on firefox and chrome in headless mode
Refer Change log for version changes
FAQs
This library allows you to connect with plivo's voice enviroment from browser
The npm package plivo-browser-sdk receives a total of 915 weekly downloads. As such, plivo-browser-sdk popularity was classified as not popular.
We found that plivo-browser-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.