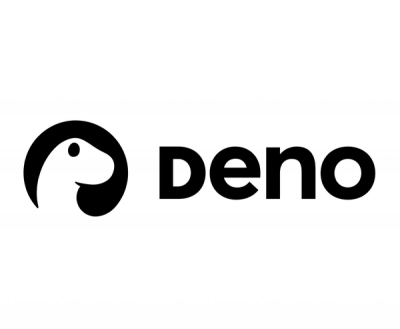
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Plop is a micro-generator framework that helps you create consistent and repeatable code snippets, files, or projects. It allows you to define templates and automate the creation of boilerplate code, making it easier to maintain consistency across your codebase.
File Generation
This feature allows you to generate files based on templates. In this example, a new component file is created using a Handlebars template. The user is prompted for the component name, and the file is generated in the specified path.
module.exports = function (plop) {
plop.setGenerator('component', {
description: 'Create a new component',
prompts: [
{
type: 'input',
name: 'name',
message: 'Component name?'
}
],
actions: [
{
type: 'add',
path: 'src/components/{{pascalCase name}}/{{pascalCase name}}.js',
templateFile: 'plop-templates/component.hbs'
}
]
});
};
Custom Actions
Plop allows you to define custom actions that can be executed as part of the generation process. In this example, a custom action type is defined and used in a generator.
module.exports = function (plop) {
plop.setActionType('customAction', function (answers, config, plop) {
// Custom action logic here
return 'Custom action executed';
});
plop.setGenerator('custom', {
description: 'Run a custom action',
prompts: [],
actions: [
{
type: 'customAction'
}
]
});
};
Template Rendering
This feature allows you to render templates with dynamic data. In this example, a README file is generated using a Handlebars template, with the project name provided by the user.
module.exports = function (plop) {
plop.setGenerator('readme', {
description: 'Create a README file',
prompts: [
{
type: 'input',
name: 'projectName',
message: 'Project name?'
}
],
actions: [
{
type: 'add',
path: 'README.md',
templateFile: 'plop-templates/readme.hbs'
}
]
});
};
Yeoman Generator is a scaffolding tool that allows you to create custom generators for project templates. It is more feature-rich and has a larger ecosystem compared to Plop, but it can be more complex to set up and use.
Hygen is a fast, scalable, and developer-friendly code generator that uses plain text templates. It is similar to Plop in terms of simplicity and ease of use, but it focuses more on speed and scalability.
Slush is a scaffolding tool that uses Gulp for code generation. It is similar to Plop in that it allows you to create custom generators, but it leverages the Gulp ecosystem for more advanced build and task automation capabilities.
Micro-generator script for doing project specific scaffolding of code structures
FAQs
Micro-generator framework that makes it easy for an entire team to create files with a level of uniformity
The npm package plop receives a total of 631,908 weekly downloads. As such, plop popularity was classified as popular.
We found that plop demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.