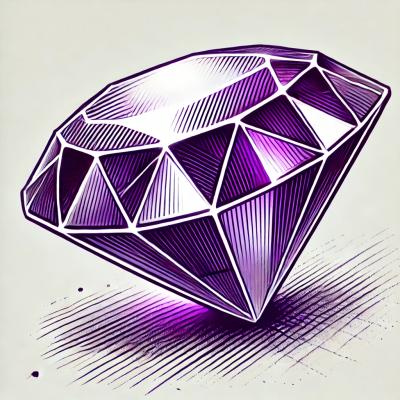
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Popsicle is a versatile HTTP request library for Node.js and the browser. It provides a simple and consistent API for making HTTP requests, handling responses, and managing various aspects of HTTP communication such as headers, query parameters, and request/response bodies.
Making HTTP Requests
This feature allows you to make HTTP requests to a specified URL. The example demonstrates a GET request to a JSON placeholder API, logging the status and body of the response.
const { request } = require('popsicle');
request('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Handling Query Parameters
This feature allows you to include query parameters in your HTTP requests. The example demonstrates a GET request with a query parameter to filter posts by userId.
const { request } = require('popsicle');
request({
url: 'https://jsonplaceholder.typicode.com/posts',
query: { userId: 1 }
})
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Setting Headers
This feature allows you to set custom headers for your HTTP requests. The example demonstrates setting the 'Content-Type' header to 'application/json'.
const { request } = require('popsicle');
request({
url: 'https://jsonplaceholder.typicode.com/posts',
headers: { 'Content-Type': 'application/json' }
})
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Handling Request and Response Bodies
This feature allows you to handle request and response bodies. The example demonstrates a POST request with a JSON body to create a new post.
const { request } = require('popsicle');
request({
method: 'POST',
url: 'https://jsonplaceholder.typicode.com/posts',
body: { title: 'foo', body: 'bar', userId: 1 },
headers: { 'Content-Type': 'application/json' }
})
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Axios is a popular promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and handling responses, similar to Popsicle. Axios is known for its ease of use and wide adoption in the JavaScript community.
Node-fetch is a lightweight module that brings `window.fetch` to Node.js. It is a minimalistic library that provides a simple API for making HTTP requests, similar to the Fetch API in the browser. Node-fetch is often used for its simplicity and compatibility with the Fetch API standard.
Superagent is a small progressive client-side HTTP request library, and Node.js module with a similar API. It provides a flexible and powerful API for making HTTP requests and handling responses. Superagent is known for its extensive feature set and ease of use.
Popsicle is designed to be easiest way for making HTTP requests by offering a consistent, intuitive and light-weight API that works with both node and the browser.
popsicle('/users.json')
.then(function (res) {
console.log(res.status) //=> 200
console.log(res.body) //=> { ... }
console.log(res.headers) //=> { ... }
})
npm install popsicle --save
bower install popsicle --save
var popsicle = require('popsicle')
// var popsicle = window.popsicle
popsicle({
method: 'POST',
url: 'http://example.com/api/users',
body: {
username: 'blakeembrey',
password: 'hunter2'
},
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
})
.then(function (res) {
console.log(res.status) // => 200
console.log(res.body) //=> { ... }
console.log(res.get('Content-Type')) //=> 'application/json'
})
"GET"
){}
)Infinity
)[stringify, headers, parse]
){}
)http.request/https.request
(node), XMLHttpRequest
(brower))Options using node transport
The default plugins under node are [stringify, headers, cookieJar, unzip, concatStream('string'), parse]
, since the extra options aren't customizable for the browser.
popsicle.jar()
) (default: null
)5
)true
)307
/308
redirects (default: true
)Options using browser transport
false
)responseType
(default: undefined
)Every method has a short hand exposed under the main Popsicle function.
popsicle.post('http://example.com/api/users')
Create a new Popsicle function with defaults set. Handy for a consistent cookie jar or transport to be used.
var cookiePopsicle = popsicle.defaults({ options: { jar: popsicle.jar() } })
Popsicle can automatically serialize the request body with the built-in stringify
plugin. If an object is supplied, it will automatically be stringified as JSON unless the Content-Type
was set otherwise. If the Content-Type
is multipart/form-data
or application/x-www-form-urlencoded
, it will be automatically serialized.
popsicle({
url: 'http://example.com/api/users',
body: {
username: 'blakeembrey'
},
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
})
You can manually create form data by calling popsicle.form
. When you pass a form data instance as the body, it'll automatically set the correct Content-Type
- complete with boundary.
var form = popsicle.form({
username: 'blakeembrey',
profileImage: fs.createReadStream('image.png')
})
popsicle.post({
url: '/users',
body: form
})
All requests can be aborted before or during execution by calling Request#abort
.
var request = popsicle('http://example.com')
setTimeout(function () {
request.abort()
}, 100)
request.catch(function (err) {
console.log(err) //=> { message: 'Request aborted', type: 'EABORTED' }
})
The request object can be used to check progress at any time.
All percentage properties (request.uploaded
, request.downloaded
, request.completed
) are a number between 0
and 1
. Aborting the request will emit a progress event, if the request had started.
var request = popsicle('http://example.com')
request.uploaded //=> 0
request.downloaded //=> 0
request.progress(function () {
console.log(request) //=> { uploaded: 1, downloaded: 0, completed: 0.5, aborted: false }
})
request.then(function (response) {
console.log(request.downloaded) //=> 1
})
The default plugins are exposed under popsicle.plugins
, which allows you to mix, match and omit some plugins for maximum usability with any use-case.
{
headers: [Function: headers],
stringify: [Function: stringify],
parse: [Function: parse],
cookieJar: [Function: cookieJar],
unzip: [Function: unzip],
concatStream: [Function: concatStream],
defaults: [
[Function: stringify],
[Function: headers],
[Function: cookieJar],
[Function: unzip],
[Function: concatStream],
[Function: parse]
]
}
User-Agent
, Accept
, Content-Length
(Highly recommended)string
(default), buffer
, array
, uint8array
, object
) (Node only)You can create a reusable cookie jar instance for requests by calling popsicle.jar
.
var jar = request.jar()
popsicle({
method: 'POST',
url: '/users',
options: {
jar: jar
}
})
Promises and node-style callbacks are both supported.
Promises are the most expressive interface. Just chain using Request#then
or Request#catch
and continue.
popsicle('/users')
.then(function (res) {
// Success!
})
.catch(function (err) {
// Something broke.
})
If you live on the edge, try using it with generators (with co) or ES7 async
.
co(function * () {
yield popsicle('/users')
})
For tooling that still expects node-style callbacks, you can use Request#exec
. This accepts a single function to call when the response is complete.
popsicle('/users')
.exec(function (err, res) {
if (err) {
// Something broke.
}
// Success!
})
Every Popsicle response will give a Response
object on success. The object provides an intuitive interface for requesting common properties.
responseURL
)200 -> 2
)application/json
)All response handling methods can return an error. Errors have a popsicle
property set to the request object and a type
string. The built-in types are documented below, but custom errors can be created using request.error(message, code, originalError)
.
Plugins can be passed in as an array with the initial options (which overrides default plugins), or they can be used via the chained method Request#use
.
Plugins must be a function that accepts configuration and returns another function. For example, here's a basic URL prefix plugin.
function prefix (url) {
return function (request) {
request.url = url + req.url
}
}
popsicle('/user')
.use(prefix('http://example.com'))
.then(function (response) {
console.log(response.url) //=> "http://example.com/user"
})
Popsicle also has a way modify the request and response lifecycle, if needed. Any registered function can return a promise to defer the request or response resolution. This makes plugins such as rate-limiting and response body concatenation possible.
resolve
or reject
popsicle.browser //=> true
Creating a custom transportation layer is just a matter creating an object with open
, abort
and use
options set. The open method should set any request information required between called as request.raw
. Abort must abort the current request instance, while open
must always resolve the promise. You can set use
to an empty array if no plugins should be used by default. However, it's recommended you keep use
set to the defaults, or as close as possible using your transport layer.
This project is written using TypeScript and has an accompanying .d.ts
file.
Install dependencies and run the test runners (node and PhantomJS using Tap).
npm install && npm test
MIT
FAQs
Advanced HTTP requests in node.js and browsers
The npm package popsicle receives a total of 78,631 weekly downloads. As such, popsicle popularity was classified as popular.
We found that popsicle demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.