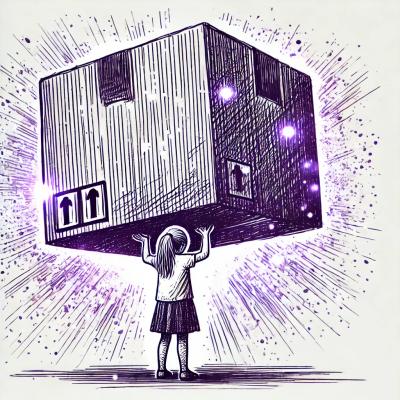
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Popsicle is a versatile HTTP request library for Node.js and the browser. It provides a simple and consistent API for making HTTP requests, handling responses, and managing various aspects of HTTP communication such as headers, query parameters, and request/response bodies.
Making HTTP Requests
This feature allows you to make HTTP requests to a specified URL. The example demonstrates a GET request to a JSON placeholder API, logging the status and body of the response.
const { request } = require('popsicle');
request('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Handling Query Parameters
This feature allows you to include query parameters in your HTTP requests. The example demonstrates a GET request with a query parameter to filter posts by userId.
const { request } = require('popsicle');
request({
url: 'https://jsonplaceholder.typicode.com/posts',
query: { userId: 1 }
})
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Setting Headers
This feature allows you to set custom headers for your HTTP requests. The example demonstrates setting the 'Content-Type' header to 'application/json'.
const { request } = require('popsicle');
request({
url: 'https://jsonplaceholder.typicode.com/posts',
headers: { 'Content-Type': 'application/json' }
})
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Handling Request and Response Bodies
This feature allows you to handle request and response bodies. The example demonstrates a POST request with a JSON body to create a new post.
const { request } = require('popsicle');
request({
method: 'POST',
url: 'https://jsonplaceholder.typicode.com/posts',
body: { title: 'foo', body: 'bar', userId: 1 },
headers: { 'Content-Type': 'application/json' }
})
.then(response => {
console.log(response.status);
console.log(response.body);
})
.catch(error => {
console.error(error);
});
Axios is a popular promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and handling responses, similar to Popsicle. Axios is known for its ease of use and wide adoption in the JavaScript community.
Node-fetch is a lightweight module that brings `window.fetch` to Node.js. It is a minimalistic library that provides a simple API for making HTTP requests, similar to the Fetch API in the browser. Node-fetch is often used for its simplicity and compatibility with the Fetch API standard.
Superagent is a small progressive client-side HTTP request library, and Node.js module with a similar API. It provides a flexible and powerful API for making HTTP requests and handling responses. Superagent is known for its extensive feature set and ease of use.
Advanced HTTP requests in node.js and browsers, using Servie.
npm install popsicle --save
import { transport, request } from 'popsicle'
const req = request('http://example.com') // Creates a `Request` instance.
const res = await transport()(req) // Transports `Request` and returns `Response` instance.
Thin wrapper to transport Servie HTTP request interfaces.
P.S. The default export from popsicle
is universal.js
. In TypeScript, this can cause trouble with types. Use specific imports such as popsicle/dist/{browser,node,universal}
instead, depending on preference.
Normalizes some behavior that happens automatically in browsers (each normalization can be disabled).
User-Agent
insertiongzip
and deflate
encodingtrue
)true
)jar()
from node.js
import)5
)307
and 308
redirects (default: () => false
)true
)http.request
agentBuffer
or array of strings or Buffers
of trusted certificates in PEM formattext
)false
)Transports can return an error. Errors have a request
property set to the request object and a code
string. The built-in codes are documented below:
Coming back soon.
throat
- Throttle promise-based functions with concurrency supportis-browser
- Check if your in a browser environment (E.g. Browserify, Webpack)parse-link-header
- Handy for parsing HTTP link headersSee Throwback for more information:
type Plugin = (req: Request, next: () => Promise<Response>) => Promise<Response>
See Servie for more information:
type Transport = (req: Request) => Promise<Response>
This project is written using TypeScript and publishes the types to NPM alongside the package.
$http
serviceMIT
FAQs
Advanced HTTP requests in node.js and browsers
The npm package popsicle receives a total of 184,724 weekly downloads. As such, popsicle popularity was classified as popular.
We found that popsicle demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.