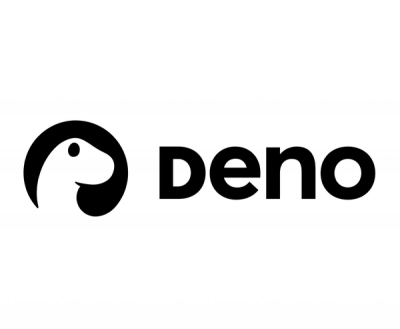
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
popups-file-dialog
Advanced tools
popups file dialog build in c on top of lib called tinyfiledialogs and ported for node
This is a simple file dialog 0 dependencies for windows, linux and macos.
build on library in c language called tinyfiledialogs.
sence it is build in c , so this lib is super fast unlike any library build in python i saw on npm
now listen, sense node is not natively have a file dialog
so i build this lib as a wrapper for the (tinyfiledialogs library build in c).
i made the cli version of the tinyfiledialogs library.
after that i build this lib as a wrapper for the cli version of the tinyfiledialogs library.
i did think of making the tinyfiledialogs library as a node addon, but this is too much work.
so i go for the easy way, and make the cli version of the tinyfiledialogs library.
still have a lot of work to do
i recently build the lib for windows and linux
i am to lazy to download macos and build the lib for macos
so if you have a macos and want to help me build the lib for macos
feel free to contribute to this lib.
compile and test the lib/vendors/src/cli.c for macos
const fileDialog = require("popups-file-dialog");
(async () => {
const result = await fileDialog.openFile({
title: 'Open File',
startPath: './',
filterPatterns: ['*'],
filterPatternsDescription: 'all files',
allowMultipleSelects: true,
})
console.log(result);
})();
windows:
linux:
(async () => {
const result = await fileDialog.openDirectory({
title: "select folder please",
})
console.log(result);
})();
windows:
linux:
npm install popups-file-dialog
yarn add popups-file-dialog
or
git clone https://github.com/native-toolkit/popups-file-dialog.git
cd popups-file-dialog
const fileDialog = require("../file-dialog");
const main = async () => {
console.log(fileDialog.config.vendorPath); // check for the path of the vendor folder if the os is supported
const result = await fileDialog.openFile({
title: "Open File",
startPath: "C:\\Users\\",
filterPatterns: ["*.exe", "*.txt"],
filterPatternsDescription: "exe files,txt files",
allowMultipleSelects: true,
});
console.log(result);
const result2 = await fileDialog.messageBox({
title: "Message Box",
message: "Hello World",
dialogType: "yesNoCancel",
iconType: "info",
defaultSelected: "yes",
});
console.log(result2);
};
main();
super important, and big object, that contain all the config of the lib all the flags with the commands and the path of the vendor folder part of it:
config = {
vendorPath: path.join(
__dirname,
"lib",
"vendors",
"bin",
`${process.platform}${process.platform === "win32" ? ".exe" : ".app"}`
),
availableCommand: {
open: {
name: "-open-file",
flags: {
title: {
name: "--title",
defaultValue: "open",
},
startPath: {
name: "--startPath",
defaultValue: path.resolve("./"),
},
filterPatterns: {
name: "--filterPatterns",
defaultValue: "*",
},
filterPatternsDescription: {
name: "--filterPatternsDescription",
defaultValue: "",
},
allowMultipleSelects: {
name: "--allowMultipleSelects",
defaultValue: "0",
},
},
},
},
};
open file dialog menu
multiple select example:
const result = await fileDialog.openFile({
title: "Open File",
startPath: "./",
filterPatterns: ["*"],
filterPatternsDescription: "all files",
allowMultipleSelects: true
})
console.log(result);
image on windows 10:
expected result:
[
'C:\\Users\\pc\\Desktop\\workingdir\\projects\\popups-file-dialog\\.gitignore',
'C:\\Users\\pc\\Desktop\\workingdir\\projects\\popups-file-dialog\\.npmignore',
'C:\\Users\\pc\\Desktop\\workingdir\\projects\\popups-file-dialog\\file-dialog.js'
]
option | type | default | description | example |
---|---|---|---|---|
title | string | "open" | title of the dialog | "Open File" |
startPath | string | path.resolve("./") | start path of the dialog | "C:\Users\" |
filterPatterns | string[] | ["*"] | filter patterns of the dialog | ["*.exe", "*.txt"] |
filterPatternsDescription | string | "" | filter patterns description of the dialog | "exe files,txt files" |
allowMultipleSelects | boolean | false | allow multiple selects of the dialog | true |
save file dialog menu
const result5 = await fileDialog.saveFile({
title: 'Save File',
startPath: 'C:\\Users\\pc\\Desktop\\New folder (36)\\test.txt',
filterPatterns: ['*'],
filterPatternsDescription: "all",
})
console.log(result5)
image on windows 10:
expected result:
"C:\\Users\\pc\\Desktop\\New folder (36)\\test.txt"
option | type | default | description | example |
---|---|---|---|---|
title | string | "save" | title of the dialog | "Save File" |
startPath | string | path.resolve("./default.txt") | start path and file name | "C:\Users\someFile.txt" |
filterPatterns | string[] | ["*"] | filter patterns of the dialog | ["*.exe", "*.txt"] |
filterPatternsDescription | string | "" | filter patterns description of the dialog | "exe files,txt files" |
open folder dialog menu
const result = await fileDialog.openDirectory({
title: "Message Box",
})
console.log(result);
image on windows 10:
expected result:
"C:\\Users\\pc\\Documents\\Adobe"
option | type | default | description | example |
---|---|---|---|---|
title | string | "open" | title of the dialog | "Open Folder" |
promote message box
const result = await fileDialog.messageBox({
title: "Message Box",
message: "Hello World",
dialogType: "yesNoCancel",
iconType: "info",
defaultSelected: "yes"
})
console.log(result);
image on windows 10:
expected result:
yes -> 1
no -> 2
cancel -> 0
const result = await fileDialog.messageBox({
title: "Message Box",
message: "Hello World",
dialogType: "okCancel",
iconType: "info",
defaultSelected: "ok"
})
console.log(result);
expected result:
ok -> 1
cancel -> 0
const result = await fileDialog.messageBox({
title: "Message Box",
message: "Hello World",
dialogType: "yesNo",
iconType: "info",
defaultSelected: "ok"
})
console.log(result);
expected result:
yes -> 1
no -> 0
option | type | default | description | example | available options |
---|---|---|---|---|---|
title | string | "message" | title of the dialog | "Message Box" | |
message | string | "message" | message of the dialog | "Hello World" | |
dialogType | string | "ok" | dialog type of the dialog | "okCancel" | "ok", "okCancel", "yesNo", "yesNoCancel" |
iconType | string | "info" | icon type of the dialog | "info" | "info", "warning", "error","question" |
defaultSelected | string | "ok" | default selected of the dialog | "ok" | "ok", "cancel", "yes", "no" |
FAQs
popups file dialog build in c on top of lib called tinyfiledialogs and ported for node
The npm package popups-file-dialog receives a total of 1 weekly downloads. As such, popups-file-dialog popularity was classified as not popular.
We found that popups-file-dialog demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.