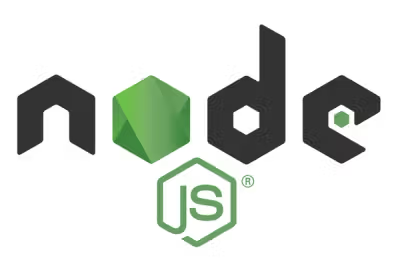
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
port_agent
Advanced tools
A RPC-like facility for making inter-thread function calls.
Port Agent provides a simple and intuitive interface that makes inter-thread function calls easy.
Error
from the other thread back to the caller.Agent.register
) persist until deregistered (i.e., Agent.deregister
) .An instance of an Agent
facilitates communication across threads. The Agent
can be used in order to register a function in one thread and call it from another thread. Calls may be made from the main thread to a worker thread, and conversely from a worker thread to the main thread.
Late binding registrants will be called with previously awaited invocations; thus preventing a race condition. This means that you may await a call to a function that has not yet been registered. Once the function is registered in the other thread it will be called and its return value or Error
will be marshalled back to the caller.
Please see the Examples for variations on the Agent
's usage.
Agent
Class<threads.MessagePort>
or <threads.Worker>
The message port.name <string>
The name of the registered function.
...args <Array<unknown>>
Arguments to be passed to the registered function.
Returns: <Promise<T>>
Errors:
Error
, the Error
will be marshalled back from the other thread to this thread and the Promise
will reject with the Error
as its failure reason.Error
will be marshalled back from the other thread to this thread and the Promise
will reject with the unhandled exception as its failure reason.Error
will be marshalled back from the other thread to this thread and the Promise
will reject with the exit code as its failure reason.name <string>
The name of the registered function.
fn <(...args: Array<any>) => any>
The registered function.
Returns: <void>
name <string>
The name of the registered function.
Returns: <void>
Agent
instance.Agent
by passing a parentPort
or a Worker
instance to the Agent
constructor:In the main thread,
const worker = new Worker(fileURLToPath(import.meta.url));
const agent = new Agent(worker);
or, in a worker thread,
const agent = new Agent(worker_threads.parentPort);
Agent
instance.Agent.register
method:agent.register('hello_world', (value: string): string => `Hello, ${value} world!`);
Agent.call
method:const greeting = await agent.call<string>('hello_world', 'happy');
In this example you will:
Agent
in the main thread.Agent
to call the hello_world
function.Agent
in the worker thread.Agent
in order to register a function to handle calls to the hello_world
function.examples/simple/index.js
import { Worker, isMainThread, parentPort } from 'node:worker_threads';
import { fileURLToPath } from 'node:url';
import { Agent } from 'port_agent';
if (isMainThread) { // This is the main thread.
void (async () => {
const worker = new Worker(fileURLToPath(import.meta.url)); // (1)
const agent = new Agent(worker); // (2)
try {
const greeting = await agent.call('hello_world', 'another'); // (3)
console.log(greeting); // (6)
}
catch (err) {
console.error(err);
}
finally {
worker.terminate();
}
})();
}
else { // This is a worker thread.
if (parentPort) {
const agent = new Agent(parentPort); // (4)
agent.register('hello_world', (value) => `Hello, ${value} world!`); // (5)
}
}
The example should log to the console:
Hello, another world!
Please see the Simple Example for a working implementation.
In this test you will:
Agent
in the main thread.Agent
to call the hello_world
function and await resolution.
hello_world
function has not yet been registered in the worker thread. The function will be called once it is registered.Agent
in the worker thread.Agent
to register the hello_world
function in the worker.Agent
to register the a_reasonable_assertion
function in the worker.Agent
to call a very_late_binding
function in the main thread that is not yet registered.Agent
to call the function registered as hello_world
and await resolution.Agent
to call the function registered as a_reasonable_assertion
and await resolution.nowThrowAnError
function in the worker thread.Agent
to register a very_late_binding
function in the main thread and log the long disposed thread's ID.Please see the comments in the code that specify each of the steps above. The output of the test is printed below.
./tests/test/index.ts
import { Worker, isMainThread, parentPort, threadId } from 'node:worker_threads';
import { fileURLToPath } from 'node:url';
import { strict as assert } from 'node:assert';
import { Agent } from 'port_agent';
if (isMainThread) { // This is the main thread.
void (async () => {
const worker = new Worker(fileURLToPath(import.meta.url)); // (1)
const agent = new Agent(worker); // (2)
worker.on('online', /*(4)*/ async () => {
try {
const greeting = await agent.call<string>('hello_world', 'again, another'); // (9)
console.log(greeting); // (11)
await agent.call('a_reasonable_assertion', 'To err is Human.'); // (12)
}
catch (err) {
console.error(`Now, back in the main thread, we will handle the`, err); // (13)
}
finally {
void worker.terminate(); // (14)
setTimeout(async () => {
try {
await agent.call<string>('hello_world', 'no more...'); // (15)
}
catch (err) {
if (err instanceof Error) {
console.error(err);
}
else if (typeof err == 'number') {
console.log(`Exit code: ${err.toString()}`); // (16)
}
}
agent.register('very_late_binding', (value: number): void => console.log(`The worker's thread ID was ${value}.`)); // (17)
}, 4);
}
});
try {
// This call will be invoked once the `hello_world` function has been bound in the worker.
const greeting = await agent.call<string>('hello_world', 'another'); // (3)
console.log(greeting); // (10)
}
catch (err) {
console.error(err);
}
})();
} else { // This is a worker thread.
function nowThrowAnError(message: string) {
// This seems reasonable...
assert.notEqual(typeof new Object(), typeof null, message);
}
function callAFunction(message: string) {
nowThrowAnError(message);
}
if (parentPort) {
try {
const agent = new Agent(parentPort); // (5)
agent.register('hello_world', (value: string): string => `Hello, ${value} world!`); // (6)
// This will throw in the main thread.
agent.register('a_reasonable_assertion', callAFunction); // (7).
await agent.call<void>('very_late_binding', threadId); // (8)
}
catch(err) {
console.error(err);
}
}
}
This test should log to the console something that looks similar to this:
Hello, another world!
Hello, again, another world!
Now, back in the Main Thread, we will handle the AssertionError [ERR_ASSERTION]: To err is Human.
at nowThrowAnError (file:///port_agent/tests/test/dist/index.js:31:16)
at callAFunction (file:///port_agent/tests/test/dist/index.js:34:9)
at Agent.tryPost (/port_agent/dist/index.js:92:33)
at MessagePort.<anonymous> (/port_agent/dist/index.js:62:36)
at [nodejs.internal.kHybridDispatch] (node:internal/event_target:762:20)
at exports.emitMessage (node:internal/per_context/messageport:23:28) {
generatedMessage: false,
code: 'ERR_ASSERTION',
actual: 'object',
expected: 'object',
operator: 'notStrictEqual'
}
Exit code: 1
The worker's thread ID was 1.
git clone https://github.com/faranalytics/port_agent.git
cd port_agent
npm run test
[1.2.12] - 2023-09-30
FAQs
A RPC-like facility for making inter-thread function calls.
The npm package port_agent receives a total of 0 weekly downloads. As such, port_agent popularity was classified as not popular.
We found that port_agent demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.