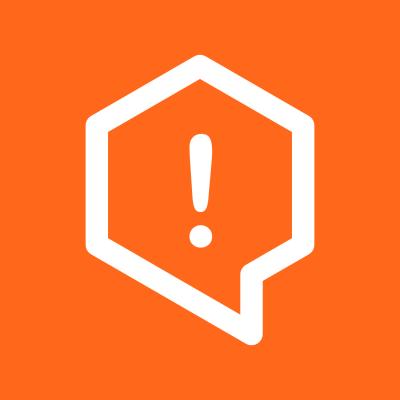
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Extends the functionality of Arrays with several useful methods
npm install pro-array --save
require('pro-array');
Requires browserify to work in the browser.
The native Array object.
See: MDN JavaScript Array Reference
Array
Array
Array
Array
Array
boolean
*
Array
Array
Array
Array
Array
Array
Array
Array
Array
Creates an array of elements split into groups the length of size
. If the array
can't be split evenly, the final chunk will be the remaining elements.
Param | Type | Default | Description |
---|---|---|---|
[size] | number | 1 | The length of each chunk. |
Returns: Array
- An array containing the chunks.
Example
[1, 2, 3, 4].chunk(2);
// -> [[1, 2], [3, 4]]
[1, 2, 3, 4].chunk(3);
// -> [[1, 2, 3], [4]]
Removes all elements from the array.
Example
var array = [1, 2, 3];
array.clear();
console.log(array);
// -> []
Array
Creates a shallow copy of the array.
Returns: Array
- A clone of the array.
Example
var a = [1, 2, 3];
var b = a.clone();
console.log(b, b === a);
// -> [1, 2, 3] false
Array
Returns a new array with all falsey values removed. Falsey values
are false
, 0
, ""
, null
, undefined
, and NaN
.
Returns: Array
- A new array containing only the truthy values of the original array.
Example
[0, 1, false, 2, '', 3].compact();
// -> [1, 2, 3]
Alias of difference.
See: difference
Array
Returns a new array with all of the values of this array that are not in any of the input arrays (performs a set difference).
Param | Type | Description |
---|---|---|
*arrays | ...Array | A variable number of arrays. |
Example
[1, 2, 3, 4, 5].difference([5, 2, 10]);
// -> [1, 3, 4]
Array
Invokes a callback function on each element in the array.
A generic iterator method similar to Array#forEach()
but with the following differences:
this
always refers to the current element in the iteration (the value
argument to the callback).false
in the callback will cancel the iteration (similar to a break
statement).safeIteration
is true
.Param | Type | Default | Description |
---|---|---|---|
callback(value,index,array) | function | A function to be executed on each element in the array. | |
[safeIteration] | boolean | false | When true , the callback will not be invoked for indexes that have been deleted or elided. |
Returns: Array
- this
Example
['a', 'b', 'c'].each(console.log.bind(console));
// -> 'a' 0 ['a', 'b', 'c']
// -> 'b' 1 ['a', 'b', 'c']
// -> 'c' 2 ['a', 'b', 'c']
// -> ['a', 'b', 'c']
['a', 'b', 'c'].each(function(value, index) {
console.log(value);
if (index === 1) return false;
});
// -> 'a'
// -> 'b'
// -> ['a', 'b', 'c']
[[1, 2], [3, 4, 5]].each(Array.prototype.pop);
// -> [[1], [3, 4]]
new Array(1).each(console.log.bind(console));
// -> undefined 0 ['a', 'b', 'c']
// -> [undefined]
new Array(1).each(console.log.bind(console), true);
// -> [undefined]
boolean
Determines if the arrays are equal by doing a shallow comparison of their elements using strict equality.
Note: The order of elements in the arrays DOES matter. The elements must be found in the same order for the arrays to be considered equal.
Param | Type | Description |
---|---|---|
array | Array | An array to compare for equality. |
Returns: boolean
- true
if the arrays are equal, false
otherwise.
Throws:
TypeError
Throws an error if the input value is null
or undefined
.Example
var array = [1, 2, 3];
array.equals(array);
// -> true
array.equals([1, 2, 3]);
// -> true
array.equals([3, 2, 1]);
// -> false
*
Retrieve an element in the array.
Param | Type | Description |
---|---|---|
index | number | A zero-based integer indicating which element to retrieve. |
Returns: *
- The element at the specified index.
Example
var array = [1, 2, 3];
array.get(0);
// -> 1
array.get(1);
// -> 2
array.get(-1);
// -> 3
array.get(-2);
// -> 2
array.get(5);
// -> undefined
Array
Performs a set intersection on this array and the input array(s).
Param | Type | Description |
---|---|---|
*arrays | ...Array | A variable number of arrays. |
Returns: Array
- An array that is the intersection of this array and the input array(s).
Example
[1, 2, 3].intersect([2, 3, 4]);
// -> [2, 3]
[1, 2, 3].intersect([101, 2, 50, 1], [2, 1]);
// -> [1, 2]
Array
Sorts an array in place using a natural string comparison algorithm and returns the array.
Param | Type | Default | Description |
---|---|---|---|
[caseInsensitive] | boolean | false | Set this to true to ignore letter casing when sorting. |
Returns: Array
- The array.
Example
var files = ['a.txt', 'a10.txt', 'a2.txt', 'a1.txt'];
files.natsort();
console.log(files);
// -> ['a.txt', 'a1.txt', 'a2.txt', 'a10.txt']
Array
Sorts an array in place using a numerical comparison algorithm (sorts numbers from lowest to highest) and returns the array.
Returns: Array
- The array.
Example
var files = [10, 0, 2, 1];
files.numsort();
console.log(files);
// -> [0, 1, 2, 3]
Array
Removes all occurrences of the passed in items from the array if they exist in the array.
Param | Type | Description |
---|---|---|
*items | ...* | Items to remove from the array. |
Returns: Array
- A reference to the array (so it's chainable).
Example
var array = [1, 2, 3, 3, 4, 3, 5];
array.remove(1);
// -> [2, 3, 3, 4, 3, 5]
array.remove(3);
// -> [2, 4, 5]
array.remove(2, 5);
// -> [4]
Array
Sorts an array in place using a reverse numerical comparison algorithm (sorts numbers from highest to lowest) and returns the array.
Returns: Array
- The array.
Example
var files = [10, 0, 2, 1];
files.rnumsort();
console.log(files);
// -> [3, 2, 1, 0]
Array
Returns an array containing every distinct element that is in either this array or the input array(s).
Param | Type | Description |
---|---|---|
*arrays | ...Array | A variable number of arrays. |
Returns: Array
- An array that is the union of this array and the input array(s).
Example
[1, 2, 3].union([2, 3, 4, 5]);
// -> [1, 2, 3, 4, 5]
Alias of unique.
See: unique
Array
Returns a duplicate-free clone of the array.
Param | Type | Default | Description |
---|---|---|---|
[isSorted] | boolean | false | If the input array's contents are sorted and this is set to true , a faster algorithm will be used to create the unique array. |
Example
// Unsorted
[4, 2, 3, 2, 1, 4].unique();
// -> [4, 2, 3, 1]
// Sorted
[1, 2, 2, 3, 4, 4].unique();
// -> [1, 2, 3, 4]
[1, 2, 2, 3, 4, 4].unique(true);
// -> [1, 2, 3, 4] (but faster than the previous example)
Array
Returns a copy of the current array without any elements from the input parameters.
Param | Type | Description |
---|---|---|
*items | ...* | Items to leave out of the returned array. |
Example
[1, 2, 3, 4].without(2, 4);
// -> [1, 3]
FAQs
Extends Arrays with useful methods of unparalleled performance
The npm package pro-array receives a total of 14 weekly downloads. As such, pro-array popularity was classified as not popular.
We found that pro-array demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.