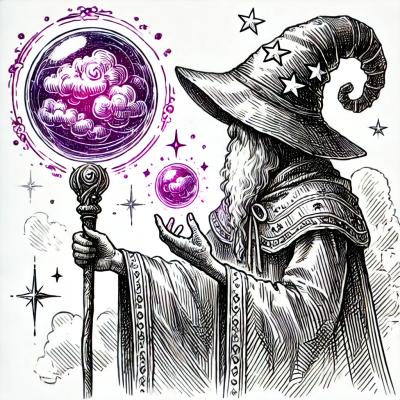
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
promise-polyfill
Advanced tools
The promise-polyfill npm package is a lightweight implementation of the ES6 Promise specification. It is designed to provide a simple and efficient way to use Promises in environments that do not natively support them, such as older browsers.
Basic Promise Usage
This code demonstrates the basic usage of a Promise. It creates a new Promise that resolves with the message 'Success!' after 1 second, and then logs the message to the console.
const Promise = require('promise-polyfill');
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Success!');
}, 1000);
});
promise.then((message) => {
console.log(message); // 'Success!'
});
Chaining Promises
This code demonstrates how to chain multiple .then() calls to handle a sequence of asynchronous operations. Each .then() call receives the resolved value from the previous Promise and returns a new value.
const Promise = require('promise-polyfill');
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve(1);
}, 1000);
});
promise
.then((value) => {
console.log(value); // 1
return value + 1;
})
.then((value) => {
console.log(value); // 2
return value + 1;
})
.then((value) => {
console.log(value); // 3
});
Handling Errors
This code demonstrates how to handle errors in Promises using the .catch() method. If the Promise is rejected, the error message is logged to the console.
const Promise = require('promise-polyfill');
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
reject('Error occurred!');
}, 1000);
});
promise
.then((value) => {
console.log(value);
})
.catch((error) => {
console.error(error); // 'Error occurred!'
});
The es6-promise package is another polyfill for the ES6 Promise specification. It provides a similar API to promise-polyfill and is widely used in environments that lack native Promise support. Compared to promise-polyfill, es6-promise is slightly larger in size but offers more comprehensive test coverage and compatibility.
Bluebird is a fully-featured Promise library that offers a wide range of additional features and optimizations beyond the ES6 Promise specification. It includes utilities for working with collections, cancellation, and more. While it is more powerful and feature-rich than promise-polyfill, it is also significantly larger in size.
Q is a popular Promise library that predates the ES6 Promise specification. It provides a rich set of features for working with asynchronous code, including support for deferred objects and advanced error handling. Q is more feature-rich than promise-polyfill but has a larger footprint and a different API.
Lightweight ES6 Promise polyfill for the browser and node. Adheres closely to the spec. It is a perfect polyfill IE, Firefox or any other browser that does not support native promises.
This implementation is based on then/promise. It has been changed to use the prototype for performance and memory reasons.
For API information about Promises, please check out this article HTML5Rocks article.
It is extremely lightweight. < 1kb Gzipped
IE8+, Chrome, Firefox, IOS 4+, Safari 5+, Opera
npm install promise-polyfill
bower install promise-polyfill
var prom = new Promise(function(resolve, reject) {
// do a thing, possibly async, then…
if (/* everything turned out fine */) {
resolve("Stuff worked!");
} else {
reject(new Error("It broke"));
}
});
// Do something when async done
prom.then(function() {
...
});
By default promise-polyfill uses setImmediate
, but falls back to setTimeout
for executing asynchronously. If a browser does not support setImmediate
(IE/Edge are the only browsers with setImmediate), you may see performance issues.
Use a setImmediate
polyfill to fix this issue. setAsap or setImmediate work well.
If you polyfill window.setImmediate
or use Promise._setImmediateFn(immedateFn)
it will be used instead of window.setTimeout
npm install setasap --save
var Promise = require('promise-polyfill');
var setAsap = require('setasap');
Promise._setImmediateFn(setAsap);
promise-polyfill will warn you about possibly unhandled rejections. It will show a console warning if a Promise is rejected, but no .catch
is used. You can turn off this behavior by setting Promise._setUnhandledRejectionFn(<rejectError>)
.
If you would like to disable unhandled rejections. Use a noop like below.
Promise._setUnhandledRejectionFn(function(rejectError) {});
npm install
npm test
MIT
FAQs
Lightweight promise polyfill. A+ compliant
We found that promise-polyfill demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.