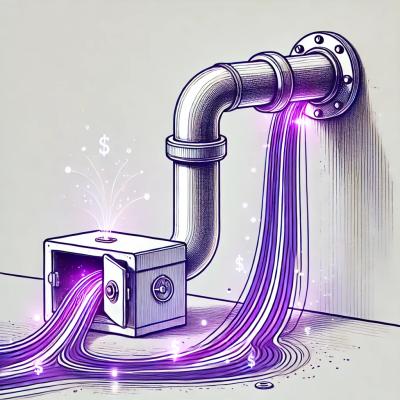
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
promise-thunk
Advanced tools
ES6 Promise + Thunk = PromiseThunk!
promise-thunk
is standard ES6 Promise implementation + thunk.
it supports node.js/io.js.
it throws unhandled rejection error.
for node.js or io.js
$ npm install promise-thunk --save
or
for browsers
https://lightspeedworks.github.io/promise-thunk/promise-thunk.js
<script src="https://lightspeedworks.github.io/promise-thunk/promise-thunk.js"></script>
you can use PromiseThunk.
(function (PromiseThunk) {
'use strict';
// you can use PromiseThunk
})(this.PromiseThunk || require('promise-thunk'));
or
var PromiseThunk = this.PromiseThunk || require('promise-thunk');
var PromiseThunk = require('promise-thunk');
function sleepNodeStyle(msec, val, cb) {
console.log(val + ' timer start: ' + msec);
setTimeout(function () {
console.log(val + ' timer end : ' + msec);
cb(null, val);
}, msec);
}
var sleepPromiseThunk = PromiseThunk.wrap(sleepNodeStyle);
// promise type
sleepPromiseThunk(1000, 'a1')
.then(
function (val) {
console.log('a2 val: ' + val);
return sleepPromiseThunk(1000, 'a2'); },
function (err) { console.log('err:' + err); })
.catch(
function (err) { console.log('err:' + err); }
);
// thunk type
sleepPromiseThunk(1000, 'b1')
(function (err, val) {
console.log('b2 val: ' + val + (err ? ' err: ' + err : ''));
return sleepPromiseThunk(1000, 'b2'); })
(function (err, val) {
console.log('b3 val: ' + val + (err ? ' err: ' + err : '')); });
// mixed promise and thunk type
sleepPromiseThunk(1000, 'c1')
(function (err, val) {
console.log('c2 val: ' + val + (err ? ' err: ' + err : ''));
return sleepPromiseThunk(1000, 'c2'); })
.then(
function (val) {
console.log('c3 val: ' + val);
return sleepPromiseThunk(1000, 'c3'); },
function (err) { console.log('err:' + err); });
//output:
// a1 timer start: 1000
// b1 timer start: 1000
// c1 timer start: 1000
// a1 timer end : 1000
// a2 val: a1
// a2 timer start: 1000
// b1 timer end : 1000
// b2 val: b1
// b2 timer start: 1000
// c1 timer end : 1000
// c2 val: c1
// c2 timer start: 1000
// a2 timer end : 1000
// b2 timer end : 1000
// b3 val: b2
// c2 timer end : 1000
// c3 val: c2
// c3 timer start: 1000
// c3 timer end : 1000
how to make promise.
p = new PromiseThunk(
function setup(resolve, reject) {
// async process -> resolve(value) or reject(error)
try { resolve('value'); }
catch (error) { reject(error); }
}
);
// setup(
// function resolve(value) {},
// function reject(error) {})
example
var p = new PromiseThunk(
function setup(resolve, reject) {
setTimeout(function () {
if (Math.random() < 0.5) resolve('value');
else reject(new Error('error'));
}, 100);
}
);
// promise
p.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); });
// thunk
p(function (err, val) { console.info('val:', val, 'err:', err); });
// mixed chanable
p(function (err, val) { console.info('val:', val, 'err:', err); })
(function (err, val) { console.info('val:', val, 'err:', err); })
(function (err, val) { console.info('val:', val, 'err:', err); })
.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); })
.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); })
(function (err, val) { console.info('val:', val, 'err:', err); })
.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); });
convert standard promise to promise thunk.
also thenable, yieldable, callable. same as thunkify.
also thenable, yieldable, callable. same as wrap.
pg
example:var pg = require('pg');
var pg_connect = thunkify.call(pg, pg.connect);
var client_query = thunkify.call(client, client.query);
how to use promise.
p = p.then(
function resolved(value) {},
function rejected(error) {});
example
p = p.then(
function resolved(value) {
console.info(value);
},
function rejected(error) {
console.error(error);
});
how to catch error from promise.
p = p.catch(
function rejected(error) {});
or
when you use old browser
p = p['catch'](
function rejected(error) {});
wait for all promises.
p = PromiseThunk.all([promise, ...]);
get value or error of first finished promise.
p = PromiseThunk.race([promise, ...]);
get resolved promise.
p = PromiseThunk.resolve(value or promise);
get rejected promise.
p = PromiseThunk.reject(error);
get resolved (accepted) promise.
p = PromiseThunk.accept(value);
make deferred object with promise.
dfd = PromiseThunk.defer();
// -> {promise, resolve, reject}
npm promise-light
npm aa
npm co
MIT
FAQs
Promise and thunk
We found that promise-thunk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.