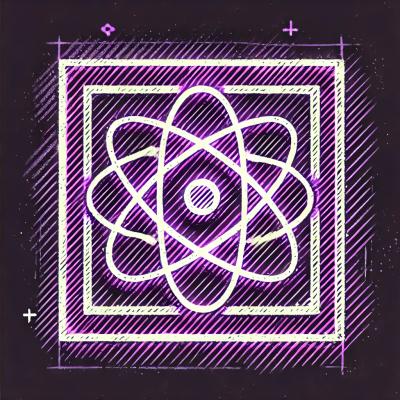
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
The 'promptly' npm package is a simple utility for prompting user input in Node.js applications. It provides a straightforward way to ask for user input from the command line, with support for validation, default values, and hidden input for sensitive information.
Basic Prompt
This feature allows you to prompt the user for input and then use that input in your application. The example code asks the user for their name and then greets them.
const promptly = require('promptly');
(async () => {
const name = await promptly.prompt('Enter your name: ');
console.log(`Hello, ${name}!`);
})();
Prompt with Default Value
This feature allows you to provide a default value for the prompt. If the user does not enter anything, the default value will be used. The example code sets 'Anonymous' as the default name.
const promptly = require('promptly');
(async () => {
const name = await promptly.prompt('Enter your name: ', { default: 'Anonymous' });
console.log(`Hello, ${name}!`);
})();
Hidden Input
This feature allows you to prompt the user for input without displaying what they type on the screen. This is useful for sensitive information like passwords. The example code asks for a password and hides the input.
const promptly = require('promptly');
(async () => {
const password = await promptly.prompt('Enter your password: ', { silent: true });
console.log('Password received.');
})();
Input Validation
This feature allows you to validate the user's input before accepting it. The example code validates that the input is a positive integer representing the user's age.
const promptly = require('promptly');
(async () => {
const age = await promptly.prompt('Enter your age: ', {
validator: (value) => {
const age = parseInt(value, 10);
if (isNaN(age) || age <= 0) {
throw new Error('Invalid age');
}
return age;
}
});
console.log(`You are ${age} years old.`);
})();
Inquirer.js is a powerful and flexible library for creating interactive command-line interfaces. It supports a wide range of prompt types, including input, confirm, list, rawlist, expand, checkbox, password, and editor. Compared to promptly, Inquirer.js offers more advanced features and customization options.
Readline-sync is a synchronous library for reading user input from the command line. It provides basic functionality for prompting user input, similar to promptly, but operates synchronously, making it simpler to use in some cases. However, it lacks some of the advanced features and flexibility of promptly.
The 'prompt' package is another library for prompting user input in Node.js. It supports various input types, validation, and default values. While it offers similar functionality to promptly, it has a more complex API and additional features like schema-based prompts.
Simple command line prompting utility.
$ npm install promptly
Prompts for a value, printing the message
and waiting for the input.
Returns a promise that resolves with the input.
Available options:
Name | Description | Type | Default |
---|---|---|---|
default | The default value to use if the user provided an empty input | string | undefined |
trim | Trims the user input | boolean | true |
validator | A validator or an array of validators | function/array | undefined |
retry | Retry if any of the validators fail | boolean | true |
silent | Do not print what the user types | boolean | false |
replace | Replace each character with the specified string when silent is true | string | '' |
input | Input stream to read from | Stream | process.stdin |
output | Output stream to write to | Stream | process.stdout |
timeout | Timeout in ms | number | 0 |
useDefaultOnTimeout | Return default value if timed out | boolean | false |
The same options are available to all functions but with different default values.
Ask for a name:
const promptly = require('promptly');
(async () => {
const name = await promptly.prompt('Name: ');
console.log(name);
})();
Ask for a name with a constraint (non-empty value and length > 2):
const promptly = require('promptly');
const validator = function (value) {
if (value.length < 2) {
throw new Error('Min length of 2');
}
return value;
};
(async () => {
const name = await promptly.prompt('Name: ', { validator });
// Since retry is true by default, promptly will keep asking for a name until it is valid
// Between each prompt, the error message from the validator will be printed
console.log('Name is:', name);
})();
Same as above but do not retry automatically:
const promptly = require('promptly');
const validator = function (value) {
if (value.length < 2) {
throw new Error('Min length of 2');
}
return value;
};
(async () => {
try {
const name = await promptly.prompt('Name: ', { validator, retry: false });
console.log('Name is:', name);
} catch (err) {
console.error('Invalid name:')
console.error(`- ${err.message}`);
}
})();
Ask for a name with timeout:
const promptly = require('promptly');
(async () => {
const name = await promptly.prompt('Name: ', { timeout: 3000 });
console.log(name);
})();
It throws an Error("timed out")
if timeout is reached and no default value is provided
The validators have two purposes: to check and transform input. They can be asynchronous or synchronous
const validator = (value) => {
// Validation example, throwing an error when invalid
if (value.length !== 2) {
throw new Error('Length must be 2');
}
// Parse the value, modifying it
return value.replace('aa', 'bb');
}
const asyncValidator = async (value) => {
await myfunc();
return value;
}
Ask the user for confirmation, printing the message
and waiting for the input.
Returns a promise that resolves with the answer.
Truthy values are: y
, yes
and 1
. Falsy values are n
, no
, and 0
.
Comparison is made in a case insensitive way.
The options are the same as prompt, except that trim
defaults to false
.
Ask to confirm something important:
const promptly = require('promptly');
(async () => {
const answer = await promptly.confirm('Are you really sure? ');
console.log('Answer:', answer);
})();
Ask the user to choose between multiple choices
(array of choices), printing the message
and waiting for the input.
Returns a promise that resolves with the choice.
The options are the same as prompt, except that trim
defaults to false
.
Ask to choose between:
const promptly = require('promptly');
(async () => {
const choice = await promptly.choose('Do you want an apple or an orange? ', ['apple', 'orange']);
console.log('Choice:', choice);
})();
Prompts for a password, printing the message
and waiting for the input.
Returns a promise that resolves with the password.
The options are the same as prompt, except that trim
and silent
default to false
and default
is an empty string (to allow empty passwords).
Ask for a password:
const promptly = require('promptly');
(async () => {
const password = await promptly.password('Type a password: ');
console.log('Password:', password);
})();
Ask for a password but mask the input with *
:
const promptly = require('promptly');
(async () => {
const password = await promptly.password('Type a password: ', { replace: '*' });
console.log('Password:', password);
})();
$ npm test
$ npm test -- --watch
during development
Released under the MIT License.
FAQs
Simple command line prompting utility
The npm package promptly receives a total of 1,391,940 weekly downloads. As such, promptly popularity was classified as popular.
We found that promptly demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.