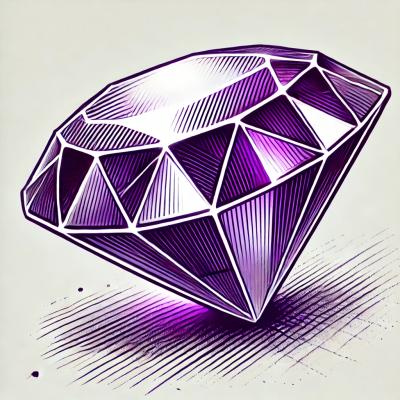
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
proper-lockfile
Advanced tools
A lockfile utility based on fs that works cross process and machine (network file systems)
The proper-lockfile npm package is used to create and manage file locks, ensuring that only one process can access a file at a time. This is particularly useful for preventing race conditions and ensuring data integrity in concurrent environments.
Locking a file
This feature allows you to lock a file to prevent other processes from accessing it. The code sample demonstrates how to lock a file, perform some operations, and then release the lock.
const lockfile = require('proper-lockfile');
async function lockFile() {
try {
const release = await lockfile.lock('somefile');
console.log('File is locked');
// Do some work with the locked file
await release();
console.log('File is released');
} catch (err) {
console.error('Failed to lock file', err);
}
}
lockFile();
Checking if a file is locked
This feature allows you to check if a file is currently locked. The code sample demonstrates how to check the lock status of a file.
const lockfile = require('proper-lockfile');
async function checkLock() {
try {
const isLocked = await lockfile.check('somefile');
console.log(`File is ${isLocked ? 'locked' : 'not locked'}`);
} catch (err) {
console.error('Failed to check lock status', err);
}
}
checkLock();
Unlocking a file
This feature allows you to manually unlock a file. The code sample demonstrates how to unlock a file that was previously locked.
const lockfile = require('proper-lockfile');
async function unlockFile() {
try {
await lockfile.unlock('somefile');
console.log('File is unlocked');
} catch (err) {
console.error('Failed to unlock file', err);
}
}
unlockFile();
The lockfile package provides similar functionality for file locking. It is simpler but less feature-rich compared to proper-lockfile. It allows you to create, check, and remove locks on files.
The async-lock package provides a general-purpose locking mechanism for asynchronous code. While it is not specifically designed for file locking, it can be used to manage access to resources in a concurrent environment.
The rwlockfile package provides read-write locks for files. It allows multiple readers or a single writer to access a file, offering more granular control compared to proper-lockfile.
A lockfile utility based on fs that works cross process and machine (network file systems).
$ npm install proper-lockfile --save
There are various ways to achieve file locking.
This library utilizes the mkdir
strategy which works atomically on any kind of file system, even network based ones.
The lockfile path is based on the file path you are trying to lock by suffixing it with .lock
.
When a lock is successfully acquired, the lockfile's mtime
(modified time) is periodically updated to prevent staleness. This allows to effectively check if a lock is stale by checking its mtime
against a stale threshold. If the update of the mtime fails several times, the lock might be compromised.
This library is similar to lockfile but the later has some drawbacks:
open
with O_EXCL
flag which has problems in network file systems. proper-lockfile
uses mkdir
which doesn't have this issue.O_EXCL is broken on NFS file systems; programs which rely on it for performing locking tasks will contain a race condition.
The lockfile staleness check is done via ctime (creation time) which is unsuitable for long running processes. proper-lockfile
constantly updates lockfiles mtime to do proper staleness check.
It does not check if the lockfile was compromised which can led to undesirable situations. proper-lockfile
checks the lockfile when updating the mtime.
Tries to acquire a lock on file
.
If the lock succeeds, an unlock
function is provided that should be called when you want to release the lock.
If the lock gets compromised, the compromised
function will be called (optionally).
Available options:
stale
: Duration in milliseconds in which the lock is considered stale, defaults to 10000
(minimum value is 2000
)update
: The interval in milliseconds in which the lockfile's mtime will be updated, defaults to 5000
(minimum value is 1000
, maximum value is stale - 1000
)retries
: The number of retries or a retry options object, defaults to 0
resolve
: Resolve to a canonical path to handle relative paths & symlinks properly, defaults to true
fs
: A custom fs to use, defaults to graceful-fs
var lockfile = require('proper-lockfile');
lockfile.lock('some/file', function (err, unlock) {
if (err) {
throw err; // Lock failed
}
// Do something while the file is locked
// Call the provided unlock function when you're done
unlock();
// Note that you can optionally handle unlock errors
// Though it's not really since it will eventually get stale
/*unlock(function (err) {
// At this point the lock was effectively released or an error
// ocurred while removing it
});*/
}, function (err) {
// If we get here, the lock has been compromised
// e.g.: the lock has been manually deleted
});
Removes a lock.
You should NOT call this function to unlock a lockfile that isn't owned by you.
This function is an alternative to the unlock
function (as explained above) and you should ONLY call it if you own the lock.
The callback
is optional because even if the removal of the lock failed, it will eventually get stale since
the lockfile's mtime will no longer be updated.
Available options:
resolve
: Resolve to a canonical path to handle relative paths & symlinks properly, defaults to true
fs
: A custom fs to use, defaults to graceful-fs
var lockfile = require('proper-lockfile');
lockfile.lock('some/file', function (err) {
if (err) {
throw err;
}
// Later..
lockfile.remove('some/file');
});
Simply run the test suite with $ npm test
The test suite is very extensive. There's even a stress test to guarantee exclusiveness of locks.
Released under the MIT License.
FAQs
A inter-process and inter-machine lockfile utility that works on a local or network file system
The npm package proper-lockfile receives a total of 863,881 weekly downloads. As such, proper-lockfile popularity was classified as popular.
We found that proper-lockfile demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.