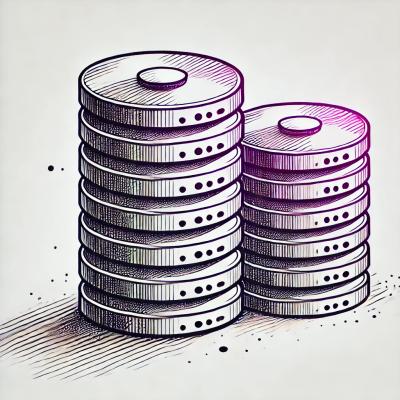
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
proto3-json-serializer
Advanced tools
Support for proto3 JSON serialiazation/deserialization for protobuf.js
The proto3-json-serializer npm package is designed to facilitate the serialization and deserialization of Protocol Buffers (protobuf) messages to and from JSON format, specifically tailored for Protocol Buffers version 3 (proto3). This package is particularly useful when integrating systems that use protobufs with those that operate with JSON, ensuring data compatibility and ease of data handling between different systems or components.
Serialization
This feature allows you to serialize a protobuf message into JSON. The code sample demonstrates how to load a protobuf schema, create a message, and serialize it into JSON.
const { serialize } = require('proto3-json-serializer');
const root = protobuf.loadSync('awesome.proto');
const AwesomeMessage = root.lookupType('awesomepackage.AwesomeMessage');
const message = AwesomeMessage.create({ awesomeField: 'Hello, World!' });
const json = serialize(message);
console.log(json);
Deserialization
This feature enables the deserialization of JSON back into a protobuf message. The code sample shows how to take a JSON object and convert it back into a protobuf message using the defined schema.
const { deserialize } = require('proto3-json-serializer');
const root = protobuf.loadSync('awesome.proto');
const AwesomeMessage = root.lookupType('awesomepackage.AwesomeMessage');
const json = { awesomeField: 'Hello, World!' };
const message = deserialize(AwesomeMessage, json);
console.log(message);
protobufjs is a comprehensive library for encoding, decoding, and manipulating protobuf messages in JavaScript. It supports both proto2 and proto3 versions. Compared to proto3-json-serializer, protobufjs offers a broader scope of functionalities including reflection, custom wrappers, and utilities for working with protobuf files directly.
google-protobuf is the official Google library for working with Protocol Buffers in JavaScript. It provides robust support for encoding and decoding protobuf messages. Unlike proto3-json-serializer, which focuses on JSON serialization, google-protobuf handles binary serialization, which is more efficient but not as human-readable or as easily integrated with web APIs.
This library implements proto3 JSON serialization and deserialization for protobuf.js protobuf objects according to the spec.
Note that the spec requires special representation of some google.protobuf.*
types
(Value
, Struct
, Timestamp
, Duration
, etc.), so you cannot just use .toObject()
since the result won't be understood by protobuf in other languages. Hence this module.
JavaScript:
const serializer = require('proto3-json-serializer');
TypeScript:
import * as serializer from 'proto3-json-serializer';
const root = protobuf.loadSync('test.proto');
const Type = root.lookupType('test.Message');
const message = Type.fromObject({...});
const serialized = serializer.toProto3JSON(message);
Serialization works with any object created by calling .create()
, .decode()
, or .fromObject()
for a loaded protobuf type. It relies on the $type
field so it will not work with a static object.
To deserialize an object from proto3 JSON, we must know its type (as returned by root.lookupType('...')
).
Pass this type as the first parameter to .fromProto3JSON
:
const root = protobuf.loadSync('test.proto');
const Type = root.lookupType('test.Message');
const json = {...};
const deserialized = serializer.fromProto3JSON(Type, json);
const assert = require('assert');
const path = require('path');
const protobuf = require('protobufjs');
const serializer = require('proto3-json-serializer');
// We'll take sample protos from google-proto-files but the code will work with any protos
const protos = require('google-proto-files');
// Load some proto file
const rpcProtos = protos.getProtoPath('rpc');
const root = protobuf.loadSync([
path.join(rpcProtos, 'status.proto'),
path.join(rpcProtos, 'error_details.proto'),
]);
const Status = root.lookupType('google.rpc.Status');
// If you have a protobuf object that follows proto3 JSON syntax
// https://developers.google.com/protocol-buffers/docs/proto3#json
// (this is an example of google.rpc.Status message in JSON)
const json = {
code: 3,
message: 'Test error message',
details: [
{
'@type': 'google.rpc.BadRequest',
fieldViolations: [
{
field: 'field',
description: 'must not be null',
},
],
},
],
};
// You can deserialize it into a protobuf.js object:
const deserialized = serializer.fromProto3JSON(Status, json);
console.log(deserialized);
// And serialize it back
const serialized = serializer.toProto3JSON(deserialized);
assert.deepStrictEqual(serialized, json);
This is not an officially supported Google project.
FAQs
Support for proto3 JSON serialiazation/deserialization for protobuf.js
The npm package proto3-json-serializer receives a total of 5,429,092 weekly downloads. As such, proto3-json-serializer popularity was classified as popular.
We found that proto3-json-serializer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.