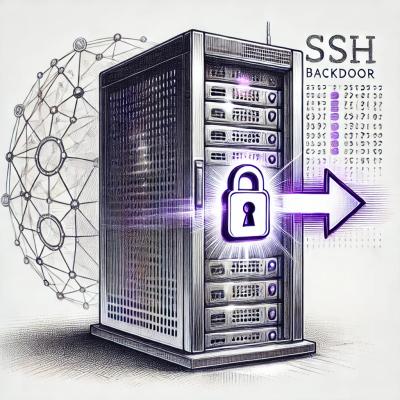
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
prunk is a mocking utility for node.js require()
. It allows you to
mock or suppress imports based on their name, regular expressions or
custom test functions.
Given you have a React component you want to test. However, since this component imports a SCSS file you cannot the import component directly. At this point, you either can introduce a pre-compiler, loader or whatever your test framework supports or you can simply mock the import.
// MyComp.js
import { Component } from 'react';
import 'style.scss';
export default class Mycomp extends Component {
// ...
}
// MyComp.spec.js
const prunk = require('prunk');
prunk.mock('style.scss', 'no scss, dude.');
// or
prunk.mock( function(req) { return 'style.scss' === req; }, 'no scss, dude');
// or better
prunk.mock( /\.(css|scss|sass)$/, 'no styles, dude');
const MyComp = require('./MyComp');
In the test require()
is used instead of import
because some pre-compilers
move all imports to the top of the file and that would make the mocking impossible.
If you use mocha you can use compiler for that.
If you just and to make sure some things don't get imported you can suppress them.
Then, they will always return undefined
;
// MyComp.spec.js
const prunk = require('prunk');
prunk.suppress('style.scss');
// or
prunk.suppress( function(req) { return 'style.scss' === req; } );
// or better
prunk.suppress( /\.(css|scss|sass)$/ );
const MyComp = require('./MyComp');
Mocks the given import with the given value.
test
can be a function that is used to compare
whatever is required and returns true
or false
.
If the return value is truthy the import will be
mocked with the given value.
The function's arguments are the same as with Module._load
.
Most of the time you will look at the first argument, a string
.
var mockStyles = (req) => 'style.css' === req;
prunk.mock( mockStyles, 'no css, dude.');
test
can also be a RegExp
that is matched agains the name
of the import or a string. It can be anything else, too, if your
imports are gone totally crazy.
prunk.mock( 'style.css', 'no css, dude.' );
prunk.mock( /\.(css|scss|sass|less)/, 'no styles, dude.');
This function returns the prunk object so that you can chain calls.
prunk.mock( ... )
.mock( ... )
.mock( ... );
Removes the mock registered for the given test
.
unmock()
uses strict equal to compare the registered
mocks.
This function returns the prunk object so that you can chain calls.
Removes all mocks. This function returns the prunk object so that you can chain calls.
Suppresses all imports that matches the given test
.
test
can be a function that is used to compare
whatever is required and returns true
or false
.
If the return value is truthy the import will be suppressed
and thus returns undefined
.
The function's arguments are the same as with Module._load
.
Most of the time you will look at the first argument, a string
.
var mockStyles = (req) => 'style.css' === req;
prunk.mock( mockStyles, 'no css, dude.');
test
can also be a RegExp
that is matched agains the name
of the import or a string or something else.
prunk.mock( 'style.css', 'no css, dude.' );
prunk.mock( /\.(css|scss|sass|less)/, 'no styles, dude.');
This function returns the prunk object so that you can chain calls.
Removes the mock registered for the given test
.
unsuppress()
uses strict equal to compare the suppressed
imports.
This function returns the prunk object so that you can chain calls.
Removes all suppressed imports. This function returns the prunk object so that you can chain calls.
FAQs
A mocking utility for node.js require
The npm package prunk receives a total of 515 weekly downloads. As such, prunk popularity was classified as not popular.
We found that prunk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.