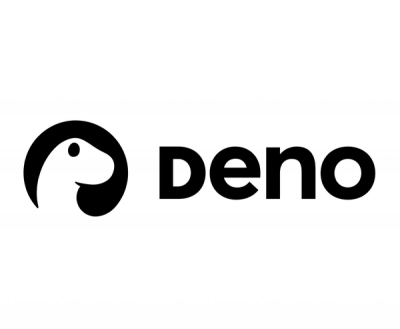
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
puppeteer-extra-plugin-devtools
Advanced tools
Make puppeteer browser debugging possible from anywhere (devtools with screencasting on the internet).
A plugin for puppeteer-extra.
yarn add puppeteer-extra-plugin-devtools
Make puppeteer browser debugging possible from anywhere.
const puppeteer = require('puppeteer-extra')
const devtools = require('puppeteer-extra-plugin-devtools')()
puppeteer.use(devtools)
puppeteer.launch().then(async browser => {
const tunnel = await devtools.createTunnel(browser)
console.log(tunnel.url) // => https://devtools-tunnel-sdoqqj95vg.localtunnel.me
const page = await browser.newPage()
await page.goto('https://www.google.com')
await page.waitFor(60 * 1000)
browser.close()
})
Extends: PuppeteerExtraPlugin
As the tunnel page is public the plugin will require basic auth.
You can set your own credentials using opts
or setAuthCredentials()
.
If you don't specify basic auth credentials the plugin will generate a password and print it to STDOUT.
opts
Type: function (opts)
opts
Object Options (optional, default {}
)
opts.auth
Object? Basic auth credentials for the public page
opts.prefix
Object? The prefix to use for the localtunnel.me subdomain (default: 'devtools-tunnel')opts.localtunnel
Object? Advanced options to pass to localtunnelExample:
const puppeteer = require('puppeteer-extra')
const devtools = require('puppeteer-extra-plugin-devtools')({
auth: { user: 'francis', pass: 'president' }
})
puppeteer.use(devtools)
puppeteer.launch().then(async browser => {
console.log('tunnel url:', (await devtools.createTunnel(browser)).url)
// => tunnel url: https://devtools-tunnel-n9aogqwx3d.localtunnel.me
})
Create a new public tunnel.
Supports multiple browser instances (will create a new tunnel for each).
Type: function (browser): Tunnel
browser
Puppeteer.Browser The browser to create the tunnel for (there can be multiple)Example:
const puppeteer = require('puppeteer-extra')
const devtools = require('puppeteer-extra-plugin-devtools')()
devtools.setAuthCredentials('bob', 'swordfish')
puppeteer.use(devtools)
;(async () => {
const browserFleet = await Promise.all(
[...Array(3)].map(slot => puppeteer.launch())
)
for (const [index, browser] of browserFleet.entries()) {
const {url} = await devtools.createTunnel(browser)
console.info(`Browser ${index}'s devtools frontend can be found at: ${url}`)
}
})()
// =>
// Browser 0's devtools frontend can be found at: https://devtools-tunnel-fzenb4zuav.localtunnel.me
// Browser 1's devtools frontend can be found at: https://devtools-tunnel-qe2t5rghme.localtunnel.me
// Browser 2's devtools frontend can be found at: https://devtools-tunnel-pp83sdi4jo.localtunnel.me
Set the basic auth credentials for the public tunnel page.
Alternatively the credentials can be defined when instantiating the plugin.
Type: function (user, pass)
Example:
const puppeteer = require('puppeteer-extra')
const devtools = require('puppeteer-extra-plugin-devtools')()
puppeteer.use(devtools)
puppeteer.launch().then(async browser => {
devtools.setAuthCredentials('bob', 'swordfish')
const tunnel = await devtools.createTunnel(browser)
})
Convenience function to get the local devtools frontend URL.
Type: function (browser): string
browser
Puppeteer.BrowserExample:
const puppeteer = require('puppeteer-extra')
const devtools = require('puppeteer-extra-plugin-devtools')()
puppeteer.use(devtools)
puppeteer.launch().then(async browser => {
console.log(devtools.getLocalDevToolsUrl(browser))
// => http://localhost:55952
})
Extends: RemoteDevTools.DevToolsTunnel
The devtools tunnel for a browser instance.
Type: function (wsEndpoint, opts)
wsEndpoint
opts
(optional, default {}
)Get the public devtools frontend url.
Type: function (): string
Example:
const tunnel = await devtools.createTunnel(browser)
console.log(tunnel.url)
// => https://devtools-tunnel-sdoqqj95vg.localtunnel.me
Get the devtools frontend deep link for a specific page.
Type: function (page): string
page
Puppeteer.PageExample:
const page = await browser.newPage()
const tunnel = await devtools.createTunnel(browser)
console.log(tunnel.getUrlForPage(page))
// => https://devtools-tunnel-bmkjg26zmr.localtunnel.me/devtools/inspector.html?ws(...)
Close the tunnel.
The tunnel will automatically stop when your script exits.
Type: function ()
Example:
const tunnel = await devtools.createTunnel(browser)
tunnel.close()
FAQs
Make puppeteer browser debugging possible from anywhere (devtools with screencasting on the internet).
The npm package puppeteer-extra-plugin-devtools receives a total of 732 weekly downloads. As such, puppeteer-extra-plugin-devtools popularity was classified as not popular.
We found that puppeteer-extra-plugin-devtools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.