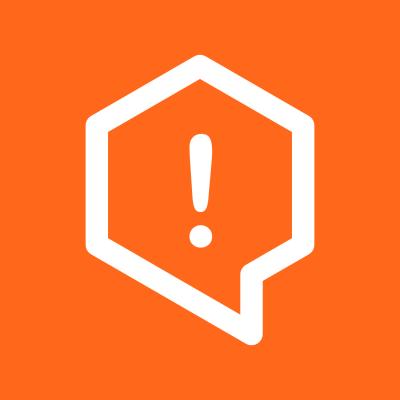
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
purecloud-platform-client-v2
Advanced tools
A JavaScript library to interface with the PureCloud Platform API
A JavaScript library to interface with the PureCloud Platform API
Install with Bower:
bower install purecloud-platform-client-v2
Install with NPM:
npm install purecloud-platform-client-v2
Reference from the CDN:
<!-- Replace `2.0.6` with the version you want to use. -->
<script src="https://sdk-cdn.mypurecloud.com/javascript/2.0.6/purecloud-platform-client-v2.min.js"></script>
View the documentation on the PureCloud Developer Center. View the source code on Github.
Reference the SDK in your HTML document. For convenience, all modules are bundled together.
<!-- Include the full library -->
<script type="text/javascript" src="purecloud-platform-client-v2.min.js"></script>
Require the SDK in your node app. All modules are obtained from the purecloud-platform-client-v2
package.
var platformClient = require('purecloud-platform-client-v2');
After authentication has completed, the access token is stored on the ApiClient
instance and the access token will be sent with all API requests.
Node.js Client Credentials grant
The Client Credentials grant only works when used in node.js. This is restricted intentionally because it is impossible for client credentials to be handled securely in a browser application.
var platformClient = require('purecloud-platform-client-v2');
var client = platformClient.ApiClient.instance;
client.loginClientCredentialsGrant(clientId, clientSecret)
.then(function() {
// Do authenticated things
})
.catch(function(response) {
console.log(`${response.status} - ${response.error.message}`);
console.log(response.error);
});
Web Implicit grant
The Implicit grant only works when used in a browser. This is because a node.js application does not have a browser interface that can display the PureCloud login window.
var platformClient = require('purecloud-platform-client-v2');
var client = platformClient.ApiClient.instance;
client.loginImplicitGrant(clientId, redirectUri)
.then(function() {
// Do authenticated things
})
.catch(function(response) {
console.log(`${response.status} - ${response.error.message}`);
console.log(response.error);
});
Any platform Provide an existing auth token
var platformClient = require('purecloud-platform-client-v2');
var client = platformClient.ApiClient.instance;
client.setAccessToken(yourAccessToken);
// Do authenticated things; no login function needed
If connecting to a PureCloud environment other than mypurecloud.com (e.g. mypurecloud.ie), set the environment on the ApiClient
instance.
var platformClient = require('purecloud-platform-client-v2');
var client = platformClient.ApiClient.instance;
client.setEnvironment('mypurecloud.ie');
In a web environment, it is possible to persist the access token to prevent an authentication request from being made on each page load. To enable this function, simply enable settings persistence prior to attempting a login. To maintain multiple auth tokens in storage, specify the prefix to use for storage/retrieval when enabling persistence. Otherwise, the prefix is optional and will default to purecloud
.
var platformClient = require('purecloud-platform-client-v2');
var client = purecloud-platform-client-v2.ApiClient.instance;
client.setPersistSettings(true, 'optional_prefix');
All API requests return a Promise which resolves to the response body, otherwise it rejects with an error. After authenticating using one of the methods defined above, the following code will make an authenticated request:
Node.js
// Create API instance
var authorizationApi = new platformClient.AuthorizationApi();
// Authenticate
client.loginClientCredentialsGrant(clientId, clientSecret)
.then(function()
// Make request to GET /api/v2/authorization/permissions
return authorizationApi.getAuthorizationPermissions();
})
.then(function(permissions) {
// Handle successful result
console.log(permissions);
})
.catch(function(response) {
// Handle failure response
console.log(`${response.status} - ${response.error.message}`);
console.log(response.error);
});
Web
// Create API instance
var usersApi = new platformClient.UsersApi();
// Authenticate
client.loginImplicitGrant(clientId, redirectUri)
.then(function() {
// Make request to GET /api/v2/users/me?expand=presence
return usersApi.getUsersMe({ 'expand': ["presence"] });
})
.then(function(userMe) {
// Handle successful result
console.log(`Hello, ${userMe.name}!`);
})
.catch(function(response) {
// Handle failure response
console.log(`${response.status} - ${response.error.message}`);
console.log(response.error);
});
By default, the SDK will return only the response body as the result of an API function call. To retrieve additional information about the response, enable extended responses. This will return the extended response for all API function calls:
var platformClient = require('purecloud-platform-client-v2');
var client = purecloud-platform-client-v2.ApiClient.instance;
client.setReturnExtendedResponses(true);
Extended response object example (body
and text
have been truncated):
{
"status": 200,
"statusText": "",
"headers": {
"inin-ratelimit-allowed": "180",
"inin-ratelimit-count": "3",
"inin-ratelimit-reset": "3",
"pragma": "no-cache",
"inin-correlation-id": "ec35f2a8-289b-42d4-8893-c50eaf81a3c1",
"content-type": "application/json",
"cache-control": "no-cache, no-store, must-revalidate",
"expires": "0"
},
"body": {},
"text": "",
"error": null
}
Error responses will always be thrown as an extended response object. Note that the error
property will contain a JavaScript Error object.
Example error response object:
{
"status": 404,
"statusText": "",
"headers": {
"inin-ratelimit-allowed": "300",
"inin-ratelimit-count": "6",
"inin-ratelimit-reset": "38",
"pragma": "no-cache",
"inin-correlation-id": "d11bd3b3-ab7e-4fd4-9687-d04af9f30a63",
"content-type": "application/json",
"cache-control": "no-cache, no-store, must-revalidate",
"expires": "0"
},
"body": {
"status": 404,
"code": "not.found",
"message": "The requested operation failed with status 404",
"contextId": "d11bd3b3-ab7e-4fd4-9687-d04af9f30a63",
"details": [],
"errors": []
},
"text": "{\"status\":404,\"code\":\"not.found\",\"message\":\"The requested operation failed with status 404\",\"contextId\":\"d11bd3b3-ab7e-4fd4-9687-d04af9f30a63\",\"details\":[],\"errors\":[]}",
"error": {
"original": null
}
}
There are hooks to trace requests and responses. To enable debug tracing, provide a log object. Optionally, specify a maximum number of lines. If specified, the response body trace will be truncated. If not specified, the entire response body will be traced out.
var platformClient = require('purecloud-platform-client-v2');
var client = purecloud-platform-client-v2.ApiClient.instance;
client.setDebugLog(console.log, 25);
FAQs
A JavaScript library to interface with the PureCloud Platform API
The npm package purecloud-platform-client-v2 receives a total of 6,390 weekly downloads. As such, purecloud-platform-client-v2 popularity was classified as popular.
We found that purecloud-platform-client-v2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.